How to handle form submissions in Laravel?
Handling form submissions in Laravel is like receiving messages from users and knowing exactly how to respond—it’s all about efficiently processing the data submitted through HTML forms and taking appropriate actions based on that data. Let’s dive into a human-friendly guide on how to handle form submissions in Laravel:
When a user submits a form on your Laravel-powered website, the data from the form is sent to a route defined in your application. This route typically points to a controller method responsible for processing the form data and performing any necessary actions, such as storing the data in a database or sending an email.
To handle form submissions, you’ll first need to define a route that points to the controller method you want to use. Laravel’s routing system makes it easy to define routes for various endpoints within your application.
For example, suppose you have a form for submitting contact messages on your website. You might define a route like this in your routes/web.php file:
php Route::post('/contact', 'ContactController@store');
In this route definition, we’re specifying that POST requests to the /contact URL should be handled by the store method of the ContactController class.
Next, you’ll need to create the ContactController and define the store method to handle the form submission. Inside the store method, you’ll have access to the incoming request object, which contains all the data submitted through the form.
Here’s a simplified example of how you might define the store method in your ContactController:
php class ContactController extends Controller { public function store(Request $request) { // Validate the form data $validatedData = $request->validate([ 'name' => 'required', 'email' => 'required|email', 'message' => 'required', ]); // Store the validated data in the database, send email, etc. // Redirect back to the form with a success message return redirect('/contact')->with('success', 'Message sent successfully!'); } }
In this example, we’re using Laravel’s built-in validation feature to validate the form data before processing it. If the validation fails, Laravel will automatically redirect the user back to the form with validation error messages. If the validation passes, you can perform any necessary actions, such as storing the data in the database or sending an email, and then redirect the user back to the form with a success message.
You’ve successfully handled form submissions in Laravel, ensuring that user input is processed securely and efficiently. By leveraging Laravel’s powerful features and intuitive syntax, you can create robust and user-friendly forms that enhance the interactivity and functionality of your web applications.
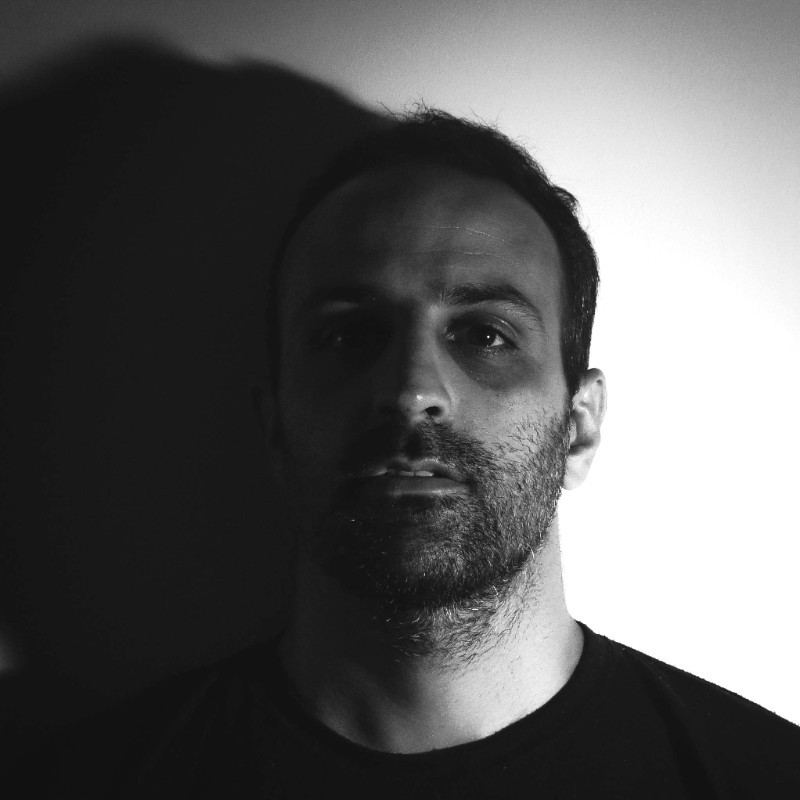
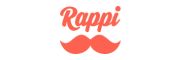