Mastering Laravel: A Guide to Building Powerful Web Applications
Laravel, an open-source PHP framework, has gained a significant following in the developer community in recent years. Its elegant syntax and feature-rich toolkit make it an excellent choice when businesses are looking to hire Laravel developers.
With an emphasis on application structure and organization, Laravel provides a superb platform for building robust, scalable web applications. Whether you’re an aspiring developer or an organization seeking to hire Laravel developers, this blog post is your comprehensive guide to mastering Laravel, offering a deep dive into its core concepts and powerful features.
Understanding Laravel
Created by Taylor Otwell in 2011, Laravel offers an expressive, elegant syntax designed to make web development tasks enjoyable and creative. It’s these qualities that make it a go-to for businesses looking to hire Laravel developers. Laravel is built with a focus on developers’ tasks such as routing, caching, security, and authentication. Its goal is to take the pain out of development by simplifying common tasks used in most web projects. So, when you hire Laravel developers, you’re investing in efficiency and creativity for your web projects.
Installation and Setup
Before we dive into Laravel’s main features, let’s start with its installation. Laravel utilizes Composer to manage its dependencies. If you haven’t installed Composer, download it from https://getcomposer.org/download/.
Once Composer is installed, you can install Laravel via the command line:
composer global require laravel/installer
Creating a New Laravel Project
After installing Laravel, you can easily create a new Laravel project with the following command
laravel new projectName
Replace “projectName” with your preferred project name, and Laravel will create a new directory with all the necessary Laravel files and configurations ready to go.
Routing
Routing is a critical part of any web application. It helps you direct application requests to appropriate controllers. In Laravel, all routes are defined in your route files, which are located in the `routes` directory.
```php Route::get('/', function () { return view('welcome'); });
The `Route::get` method accepts two arguments: the URI that the route responds to and a closure that receives the incoming HTTP request.
Controllers
Controllers group related HTTP request handling logic. You can create a controller using the `make:controller` Artisan command:
```bash php artisan make:controller UserController
This command will create a new `UserController` class in the `app/Http/Controllers` directory. You can now define methods on the controller that can be attached to routes.
```php Route::get('user', 'UserController@index');
Here, when the application receives a GET request to the ‘/user’ URI, it executes the `index` method on the `UserController`.
Blade Templating Engine
Laravel comes with a powerful templating engine called Blade. It is intuitive and helps to work with the typical PHP/HTML spaghetti that is common in traditional PHP applications, making your views cleaner and more readable.
Here is an example of a Blade view:
```html <!DOCTYPE html> <html> <head> <title>My App</title> </head> <body> <div class="container"> @yield('content') </div> </body> </html>
In this example, `@yield(‘content’)` is a placeholder where your page’s specific content will be inserted.
Database: Migrations, Seeding, and Eloquent ORM
Laravel makes interacting with databases extremely easy across a variety of database backends using either raw SQL, a query builder, and Eloquent ORM.
Migrations are like version control for your database. They allow a team to modify the database schema and stay up-to-date on the current schema state.
To create a migration, you can use the `make:migration` command:
```bash php artisan make:migration create_users_table
Eloquent ORM included with Laravel provides a simple ActiveRecord implementation for working with your database. Each database table has a corresponding “Model” that allows you to interact with that table.
Database Seeding is the process of filling your database with data. This data can be testing data or default data like roles or user statuses.
Authentication
Laravel makes implementing authentication very straightforward. It ships with views, routes, and controllers as a part of its `laravel/ui` package that is needed for the entire authentication process, including registering, logging in, and password resets.
```bash composer require laravel/ui php artisan ui bootstrap --auth
Conclusion
There’s a lot more to Laravel than what we’ve covered here: middleware, events, notifications, file storage, and so much more. But with the basics under your belt, you’re well on your way to mastering Laravel. As you learn more about its features and experiment with its capabilities, you increase your proficiency. This skill set is exactly what companies look for when they hire Laravel developers.
Laravel is a powerful tool in the hands of those who know how to use it. By mastering Laravel, you’ll be prepared to create robust, secure, and efficient web applications that can scale as needed. This mastery is especially crucial for businesses that hire Laravel developers, as it ensures high-quality results.
Remember, mastering Laravel isn’t just about memorizing methods or using preset commands. It’s about understanding the philosophy behind the framework, its elegant syntax, and its intention to make the process of coding not just easier but more enjoyable. So, whether you’re looking to hire Laravel developers or aspire to become one, always remember – Happy coding!
Table of Contents
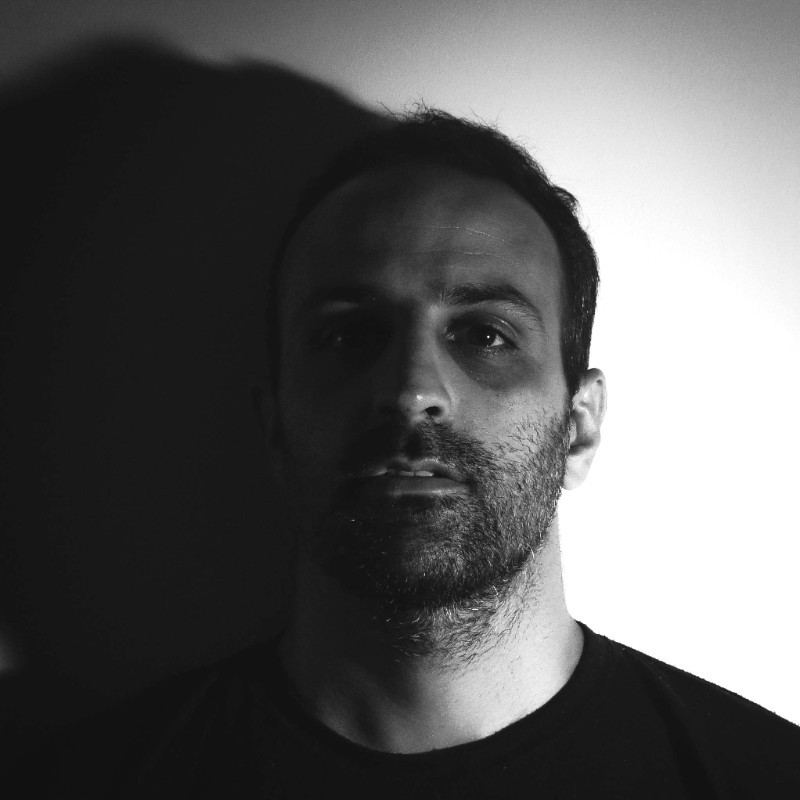
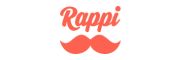