Step-by-Step Guide to Importing and Exporting Excel Files in Laravel
Laravel, an open-source PHP framework, has become a popular choice for developers due to its elegant syntax and extensive features. One such feature is the ability to handle Excel files, a common requirement in web applications. Laravel Excel, a package developed by Maatwebsite, simplifies the process of importing and exporting Excel files in Laravel applications. If you’re looking to leverage these capabilities but need professional help, you might consider to hire Laravel developers. In this blog post, we will explore how to use Laravel Excel to import and export Excel files, complete with examples.
Table of Contents
1. Installation
To start using Laravel Excel, you first need to install it via Composer. Run the following command in your terminal:
```bash composer require maatwebsite/excel ```
After the package is installed, you need to publish the config file:
```bash php artisan vendor:publish --provider="Maatwebsite\Excel\ExcelServiceProvider" ```
This command will create a `config/excel.php` file in your application. You can modify this file to suit your needs.
2. Importing Excel Files
Let’s start with importing Excel files. Laravel Excel provides a simple, fluent interface to import your Excel file’s data. Here’s a basic example:
```php namespace App\Imports; use App\User; use Maatwebsite\Excel\Concerns\ToModel; class UsersImport implements ToModel { public function model(array $row) { return new User([ 'name' => $row[0], 'email' => $row[1], 'password' => \Hash::make($row[2]), ]); } } ```
In this example, we’re importing a list of users from an Excel file. Each row in the Excel file corresponds to a user, with the first column being the name, the second column being the email, and the third column being the password.
To import the file, you would use the `import` method provided by Laravel Excel:
```php use App\Imports\UsersImport; use Maatwebsite\Excel\Facades\Excel; Excel::import(new UsersImport, 'users.xlsx'); ```
This code will import the users from the `users.xlsx` file.
3. Handling Import Failures
Laravel Excel provides a way to handle failures during the import process. You can implement the `SkipsOnError` and `SkipsOnFailure` interfaces to skip errors and failures:
```php namespace App\Imports; use App\User; use Maatwebsite\Excel\Concerns\ToModel; use Maatwebsite\Excel\Concerns\SkipsOnError; use Maatwebsite\Excel\Concerns\SkipsOnFailure; use Throwable; class UsersImport implements ToModel, SkipsOnError, SkipsOnFailure { public function model(array $row) { return new User([ 'name' => $row[0], 'email' => $row[1], 'password' => \Hash::make($row[2]), ]); } public function onError(Throwable $e) { // Handle the error } public function onFailure(Failure ...$failures) { // Handle the failure } } ```
4. Exporting Excel Files
Exporting Excel files is just as straightforward. Here’s a basic example:
```php namespace App\Exports; use App\User; use Maatwebsite\Excel\Concerns\FromCollection; class UsersExport implements FromCollection { public function collection() { return User::all(); } } ```
In this example, we’re exporting all users to an Excel file. The `collection` method returns the data that should be exported.
To export the data, you would use the `download` or `store` methods provided by Laravel Excel:
```php use App\Exports\UsersExport; use Maatwebsite\Excel\Facades\Excel; return Excel::download(new UsersExport, 'users.xlsx'); ```
This code will download the exported users as a `users.xlsx` file.
5. Customizing Exported Data
Laravel Excel provides several ways to customize the exported data. For example, you can implement the `WithHeadings` interface to add headings to the exported data:
```php namespace App\Exports; use App\User; use Maatwebsite\Excel\Concerns\FromCollection; use Maatwebsite\Excel\Concerns\WithHeadings; class UsersExport implements FromCollection, WithHeadings { public function collection() { return User::all(); } public function headings(): array { return [ 'ID', 'Name', 'Email', 'Created at', 'Updated at', ]; } } ```
In this example, the exported data will include a header row with the specified headings.
Conclusion
Laravel Excel is a powerful package that simplifies the process of importing and exporting Excel files in Laravel applications. With its fluent interface and extensive features, it’s a great tool to have in your Laravel toolkit. Whether you’re importing a list of users or exporting a report, Laravel Excel has got you covered. If you need specialized assistance, you can hire Laravel developers to implement these features seamlessly in your project.
Table of Contents
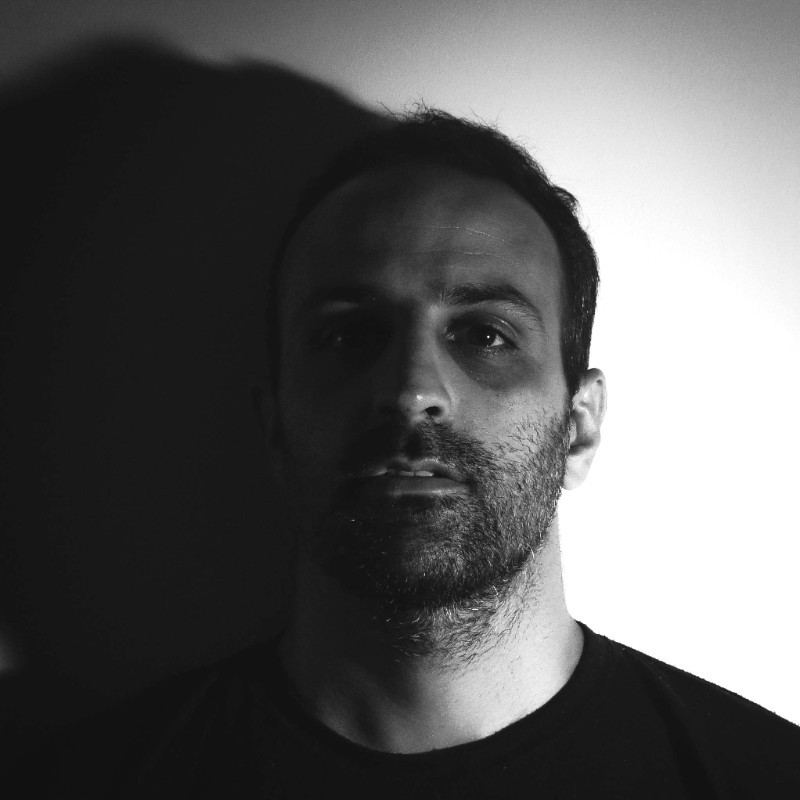
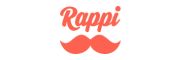