Interview Guide: Hire Laravel Developers
Laravel has emerged as one of the leading PHP frameworks, making it essential to hire skilled Laravel developers to build robust and efficient web applications.
Table of Contents
This comprehensive interview guide will help you identify top Laravel talent and build a proficient development team.
1. Key Skills of Laravel Developers to Look Out For:
Before diving into the interview process, take note of the crucial skills to look for in Laravel developers:
- PHP Proficiency: Strong knowledge of PHP programming language, with a focus on Laravel framework.
- Laravel Framework: Experience with Laravel’s features, including Eloquent ORM, Blade templating engine, routing, and middleware.
- RESTful APIs: Proficiency in building and consuming RESTful APIs for seamless integration with third-party services.
- Database Management: Knowledge of MySQL or other relational databases and the ability to design efficient database schemas.
- Authentication & Security: Understanding of authentication mechanisms in Laravel, such as JWT and OAuth, and ensuring secure application development.
- Testing & Debugging: Familiarity with testing frameworks like PHPUnit and debugging techniques for delivering bug-free code.
2. An Overview of the Laravel Developer Hiring Process:
- Defining Job Requirements & Skills: Clearly outline the technical skills and experience you are seeking in a Laravel developer, including PHP proficiency, Laravel framework knowledge, and database management expertise.
- Creating an Effective Job Description: Craft a comprehensive job description with a clear title, specific requirements, and details about the projects the developer will work on.
- Preparing Interview Questions: Develop a list of technical questions to assess candidates’ Laravel knowledge, problem-solving abilities, and experience in building web applications.
- Evaluating Candidates: Use a combination of technical assessments, coding challenges, and face-to-face interviews to evaluate potential candidates and determine the best fit for your organization.
3. Technical Interview Questions and Sample Answers:
Q1. Explain the difference between Laravel’s Eloquent ORM and Query Builder. When would you use each?
Sample Answer:
Eloquent ORM is an Object-Relational Mapping system in Laravel that allows us to interact with the database using object-oriented syntax. It simplifies database interactions by representing database tables as model classes. On the other hand, Query Builder provides a convenient way to build database queries using a chainable syntax. We use Eloquent ORM for complex relationships and data manipulations, while Query Builder is suitable for simple and straightforward queries.
Q2. How do you handle user authentication in Laravel? Explain the steps to implement JWT (JSON Web Tokens) authentication.
Sample Answer:
In Laravel, we handle user authentication using the built-in Auth
facade and middleware. To implement JWT authentication, we first install the tymon/jwt-auth
package. Then, we configure the package, create a custom middleware to handle JWT authentication, and protect the relevant routes. JWT allows us to generate a token upon successful authentication, which the client can use for subsequent API requests.
Q3. Explain Laravel’s middleware and how it is used in web applications. Provide an example of creating custom middleware.
Sample Answer:
Middleware in Laravel acts as a filter for HTTP requests, allowing us to perform tasks before or after the request reaches the controller. It helps with authentication, logging, and other request-related operations. For example, we can create a custom middleware to log requests and responses:
namespace App\Http\Middleware; use Closure; use Illuminate\Support\Facades\Log; class LogRequestsMiddleware { public function handle($request, Closure $next) { Log::info('Request: ' . $request->getPathInfo()); $response = $next($request); Log::info('Response: ' . $response->status()); return $response; } }
Q4. How do you handle form validation in Laravel? Provide an example of validating user input and displaying errors.
Sample Answer:
In Laravel, we use validation rules and the Validator
class to handle form validation. For example:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Validator; public function store(Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required|string|max:255', 'email' => 'required|email|unique:users', 'password' => 'required|string|min:8', ]); if ($validator->fails()) { return redirect()->back()->withErrors($validator)->withInput(); } // Store the data in the database }
Q5. Explain the concept of Laravel migrations and how they are used to manage database schema changes.
Sample Answer:
Laravel migrations are version control for your database, allowing you to manage and share changes to the database schema across the development team. Each migration file represents a set of database changes. We use Artisan commands to create and run migrations, ensuring that the database schema is up-to-date across different environments.
Q6. How do you handle database relationships in Laravel’s Eloquent ORM? Explain one-to-many and many-to-many relationships with examples.
Sample Answer:
In Laravel’s Eloquent ORM, we use the belongsTo
, hasMany
, and belongsToMany
methods to define database relationships. For example:
One-to-Many Relationship:
class User extends Model { public function posts() { return $this->hasMany(Post::class); } }
Many-to-Many Relationship:
class User extends Model { public function roles() { return $this->belongsToMany(Role::class); } }
Q7. How do you optimize the performance of Laravel applications? Mention some techniques to reduce database queries and improve response times.
Sample Answer:
To optimize Laravel applications, we can implement several techniques. Caching is one method to reduce database queries by storing frequently accessed data in cache memory. We can use Laravel’s caching mechanisms such as Redis or Memcached. Additionally, eager loading relationships helps in avoiding the N+1 query problem. Implementing proper indexes on frequently queried columns in the database can also significantly improve response times.
Q8. Explain the purpose of Laravel’s Service Container and how it helps with dependency injection.
Sample Answer:
Laravel’s Service Container is a powerful tool for managing class dependencies and performing dependency injection. It acts as a centralized repository for all classes and their dependencies in the application. When a class requires an instance of another class or interface, the Service Container automatically resolves and injects the required instance. This promotes decoupling and facilitates testing, as we can easily mock dependencies during unit testing.
Q9. How do you handle RESTful API development in Laravel? Provide an example of creating an API endpoint to retrieve data.
Sample Answer:
In Laravel, we use the api
middleware group to handle RESTful API development. Here’s an example of creating an API endpoint to retrieve data:
use Illuminate\Http\Request; Route::get('/products', function () { $products = Product::all(); return response()->json($products); });
Q10. How do you handle file uploads in Laravel? Provide an example of uploading and storing a file.
Sample Answer:
In Laravel, we handle file uploads using the store
method of the Request
object and the Storage
facade. For example:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Storage; public function uploadFile(Request $request) { if ($request->hasFile('file')) { $path = $request->file('file')->store('uploads'); // Store the file path in the database or perform other operations } }
4. The Importance of the Interview Process to Find the Right Laravel Talent:
The interview process plays a crucial role in identifying the right Laravel developers who possess the necessary technical skills and expertise to build efficient and scalable web applications. Laravel development involves complex tasks, including database management, authentication, and RESTful API integration. Thorough technical interviews help assess candidates’ problem-solving abilities, code quality, and familiarity with Laravel’s best practices.
5. How CloudDevs Can Help You Hire Laravel Developers:
Hiring top Laravel developers can be a challenging task, especially when you need to find developers with specific expertise. CloudDevs offers a reliable platform to simplify your hiring process and connect you with pre-screened senior Laravel developers.
Here’s how CloudDevs can assist you:
- Discuss your project requirements and desired skill sets with a dedicated consultant.
- Receive a curated shortlist of top Laravel developers within a short period.
- Conduct technical interviews and coding assessments to evaluate candidates’ proficiency.
- Start a risk-free trial with your selected Laravel developer to ensure a perfect fit for your projects.
With CloudDevs, you can build a highly capable Laravel development team and focus on creating robust and feature-rich web applications. Leave the hiring process to the experts at CloudDevs and embark on your Laravel development journey with confidence.
Table of Contents
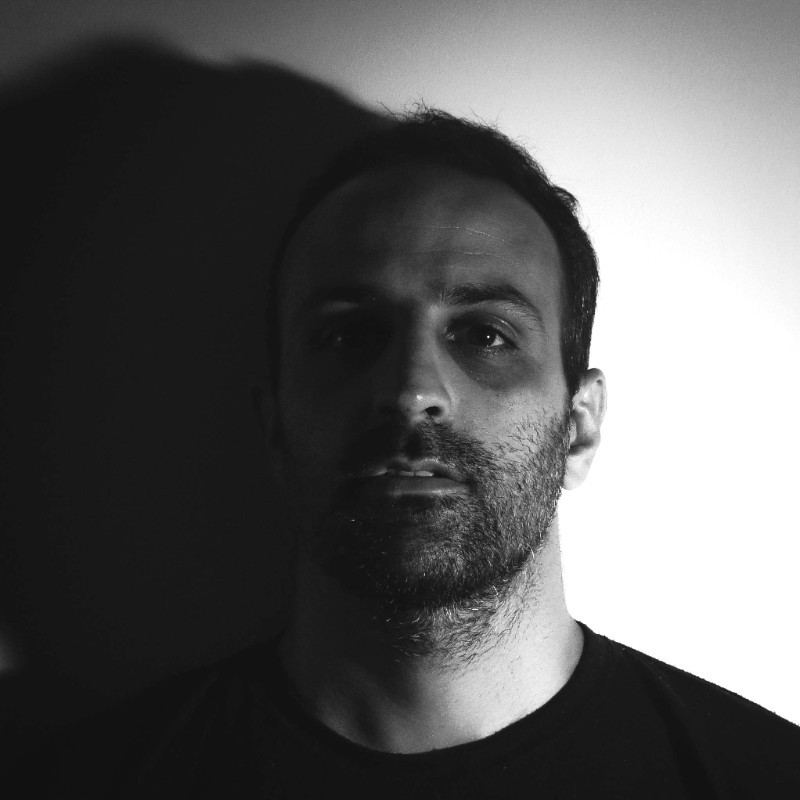
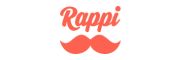