The Ultimate Guide to Streamlining Assets in Laravel with Mix and Webpack
When developing web applications, it’s crucial to maintain a smooth and efficient process for managing and compiling assets. Laravel Mix, a powerful API for Webpack, simplifies this task for Laravel developers. In this blog post, we’ll dive into Laravel Mix and explore how it uses Webpack to compile and optimize assets for Laravel projects.
1. What is Laravel Mix?
Laravel Mix is an elegant wrapper around Webpack for the 80% use case. It simplifies the configuration process, making asset compilation a breeze. With Mix, you can compile CSS, JavaScript, and images, and even handle versioning and source maps.
2. Setting up Laravel Mix
If you’ve started a new Laravel project, Laravel Mix will be included by default. If not, you can easily install it using NPM:
``` npm install laravel-mix --save-dev ```
Then, in your project’s root directory, you’ll find a `webpack.mix.js` file, which is where you’ll define your asset compilation.
3. Basic Compilation with Laravel Mix
Consider this simple example:
```javascript const mix = require('laravel-mix'); mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css'); ```
Here, we’re instructing Mix to compile the `app.js` file from our `resources` directory to `public/js` and our `app.scss` file to `public/css`.
Run the following command to compile the assets:
``` npm run dev ```
4. Advanced Features of Laravel Mix
- Versioning: Mix can handle versioning of your files to cache-bust them. It appends a unique hash to the filename, ensuring the browser fetches the updated file.
```javascript mix.js('resources/js/app.js', 'public/js') .version(); ```
- Source Maps: They help in debugging compiled files. By default, Mix generates them only for development.
```javascript mix.js('resources/js/app.js', 'public/js') .sourceMaps(); ```
- BrowserSync: This tool automatically reloads the page upon file changes. Mix integrates seamlessly with it.
```javascript mix.browserSync('my-domain.dev'); ```
5. Delving into Webpack
Under the hood, Laravel Mix is using Webpack. Webpack is a module bundler and task runner that takes modules with dependencies and generates static assets.
5.1 Examples of Webpack with Laravel Mix
- Handling Images
You might want to optimize images or move them to the public directory. With Webpack and Mix, you can do this effortlessly.
```javascript mix.copy('resources/images', 'public/images'); ```
For image optimization:
```javascript const ImageMinimizerPlugin = require("image-minimizer-webpack-plugin"); mix.webpackConfig({ plugins: [ new ImageMinimizerPlugin({ minimizerOptions: { plugins: [ ["gifsicle", { interlaced: true }], ["jpegtran", { progressive: true }], ["optipng", { optimizationLevel: 5 }], ], }, }), ], }); ```
- React Support
You might also want to use React in your Laravel app. This is made simple with Mix.
```javascript mix.react('resources/js/app.jsx', 'public/js'); ```
- Extract Vendor Libraries
For better caching, you might want to split your app and vendor libraries.
```javascript mix.js('resources/js/app.js', 'public/js') .extract(['vue', 'axios', 'lodash']); ```
6. Optimizing with Webpack and Laravel Mix
Webpack’s real strength is in its vast ecosystem and plugins. To further optimize your Laravel application, you can tap into these.
– UglifyJsPlugin: Minifies your JavaScript.
```javascript const UglifyJsPlugin = require('uglifyjs-webpack-plugin'); mix.webpackConfig({ plugins: [ new UglifyJsPlugin() ] }); ```
– CSSNano: Minifies your CSS.
It’s included in Laravel Mix’s `mix.sass()` and `mix.less()` methods, so no additional configuration is required!
Conclusion
Laravel Mix, backed by the robustness of Webpack, provides Laravel developers with an elegant and easy-to-use solution for asset compilation and optimization. This ensures your application not only functions correctly but also remains efficient and performant. Embrace Laravel Mix and let it handle the heavy lifting of assets, allowing you to focus on crafting amazing applications.
Table of Contents
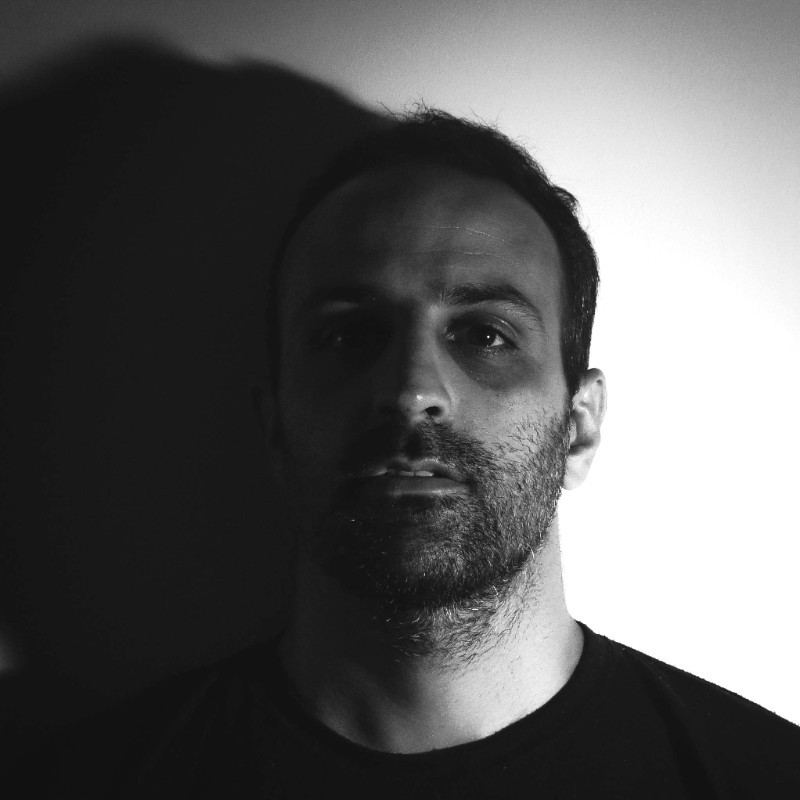
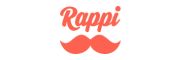