Transform User Interactions: Send Dynamic SMS & Voice Notifications with Laravel and Twilio
Laravel, one of the most popular PHP frameworks, offers a robust notification system that enables developers to send notifications across various channels, such as email, Slack, and SMS. Twilio, a cloud communications platform, offers APIs for sending SMS, making voice calls, and even video calls. Combining Laravel Notifications with Twilio can enable developers to quickly send SMS and voice notifications. In this blog post, we’ll walk you through how to send SMS and voice notifications using Laravel and Twilio.
1. Prerequisites
Before getting started, you need:
- Laravel installation.
- Twilio account and the necessary credentials (Account SID, Auth Token, Phone Number).
- Twilio PHP SDK: `composer require twilio/sdk`
2. Laravel Notification
Laravel provides a straightforward way to create notifications using the artisan command-line tool:
``` php artisan make:notification TwilioNotification ```
This command will create a new notification class in the `app/Notifications` directory.
3. Sending an SMS Notification
Step 1: Setting up Twilio Configuration
In your `.env` file, add your Twilio credentials:
``` TWILIO_SID=your_account_sid TWILIO_TOKEN=your_auth_token TWILIO_PHONE=your_twilio_phone_number ```
And then, in your `config/services.php`, add:
```php 'twilio' => [ 'sid' => env('TWILIO_SID'), 'token' => env('TWILIO_TOKEN'), 'phone' => env('TWILIO_PHONE'), ], ```
Step 2: Define the Notification
In the `TwilioNotification.php` file, you need to set up the toTwilio method that sends the SMS.
```php use Illuminate\Notifications\Notification; public function toTwilio($notifiable) { return (new TwilioMessage()) ->content('Your SMS content here.'); } ```
Step 3: Sending the Notification
To send the SMS, you’d typically do:
```php $user->notify(new TwilioNotification()); ```
Ensure the user model (or whichever notifiable model you’re notifying) has a `routeNotificationForTwilio()` method that returns the phone number:
```php public function routeNotificationForTwilio() { return $this->phone; // assuming 'phone' is a field in your database } ```
4. Sending a Voice Notification
Voice notifications are similar to SMS but with a twist. Instead of sending a text, you’ll be instructing Twilio to make a call and play a message.
Step 1: Create a TwiML Bin in Twilio
First, you need to set up a TwiML Bin in your Twilio dashboard. This is an XML that instructs Twilio on what to do when the call is answered.
Here’s a basic example:
```xml <?xml version="1.0" encoding="UTF-8"?> <Response> <Say voice="alice">Hello! This is your voice notification from our app.</Say> </Response> ```
Make note of the TwiML Bin URL after saving it.
Step 2: Modify the Notification
In `TwilioNotification.php`, we’ll create a new method called `toTwilioCall()`.
```php public function toTwilioCall($notifiable) { return (new TwilioCall()) ->url('your_twiml_bin_url'); } ```
Step 3: Sending the Voice Notification
Much like the SMS, you’d use:
```php $user->notify(new TwilioNotification()); ```
And ensure the user model has a `routeNotificationForTwilioCall()` method:
```php public function routeNotificationForTwilioCall() { return $this->phone; } ```
Conclusion
Integrating Laravel with Twilio offers a seamless way to communicate with your users. Whether it’s a timely SMS or a personalized voice call, these notifications enhance the user experience and offer more flexibility for developers.
With the rapid advancement of technology, leveraging such integrations can set your application apart and provide users with timely updates in the form they prefer. Experiment with different types of notifications and find what works best for your application and user base.
Table of Contents
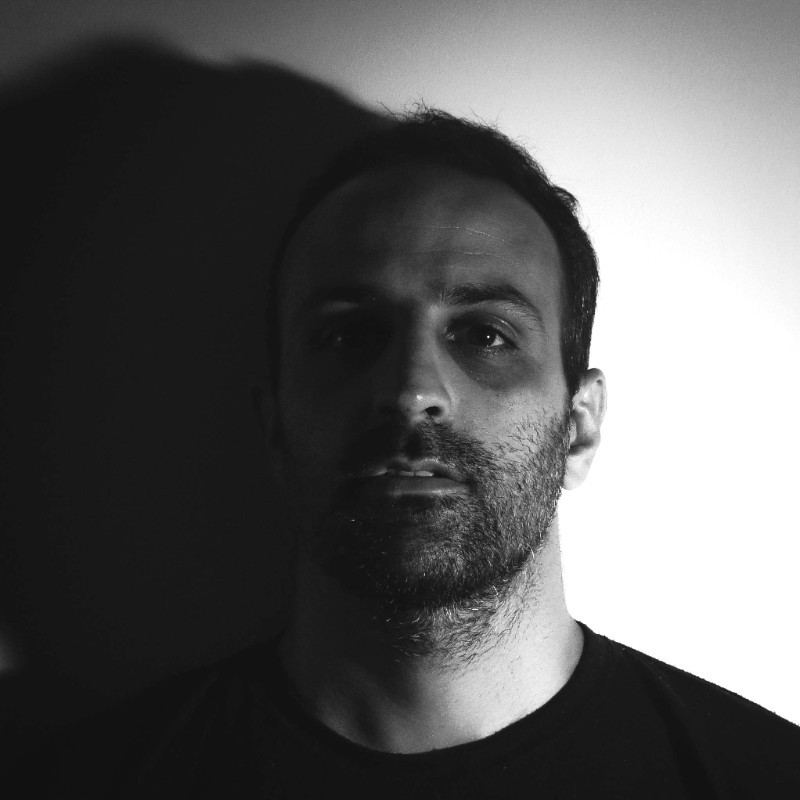
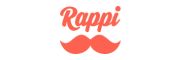