Laravel Packages: Extending Functionality with Third-Party Libraries
Laravel, one of the most popular PHP frameworks, provides a solid foundation for building robust and scalable web applications. One of the key strengths of Laravel is its extensibility through packages, which allow developers to easily integrate third-party libraries and extend the functionality of their applications.
In this blog post, we’ll explore the world of Laravel packages and how they can help you enhance your applications with additional features and integrations. We’ll cover the basics of packages, their installation, and demonstrate how to integrate some commonly used third-party libraries into your Laravel projects. So, let’s dive in!
What are Laravel Packages?
In Laravel, packages are reusable libraries or components that can be easily integrated into your application. They encapsulate specific functionalities, such as authentication, payment gateways, image manipulation, and much more. Packages provide a modular approach to development, allowing you to focus on specific features without reinventing the wheel.
Packages are typically hosted on platforms like Packagist, which is the default package repository for Composer, the popular PHP dependency manager. Laravel utilizes Composer to manage packages, making it straightforward to install and include them in your projects.
Installing Laravel Packages
Before we delve into integrating specific libraries, let’s first understand how to install Laravel packages. The process is quite simple and can be accomplished in a few steps:
- Identify the Package: Search for a Laravel package that provides the functionality you need. You can browse Packagist (https://packagist.org/) or explore the Laravel community’s resources to find the right package for your requirements.
2. Install via Composer: Once you’ve identified the package, you can install it using Composer. Open a terminal window, navigate to your project’s root directory, and run the following command:
lua composer require vendor/package-name
Replace vendor/package-name with the actual package you want to install. Composer will automatically download the package and update your composer.json file.
3. Register the Service Provider: After installation, you need to register the package’s service provider in your Laravel application. Open the config/app.php file and add the service provider to the providers array:
php 'providers' => [ // Other service providers... Vendor\Package\ServiceProvider::class, ],
Make sure to replace Vendor\Package\ServiceProvider with the actual namespace of the package’s service provider.
4. Publish Configuration (if required): Some packages may require additional configuration files. You can publish these files to your application’s config directory by running the following command:
arduino php artisan vendor:publish --provider="Vendor\Package\ServiceProvider"
Replace Vendor\Package\ServiceProvider with the actual namespace of the package’s service provider.
By following these steps, you can easily install and integrate Laravel packages into your application. Now, let’s explore some popular third-party libraries and see how they can extend the functionality of your Laravel projects.
Integrating Third-Party Libraries
Intervention Image
The Intervention Image library provides a simple and powerful way to manipulate images in Laravel. With this package, you can resize, crop, apply filters, and perform various image operations effortlessly. Let’s see how to integrate this library into your Laravel application:
Install the Intervention Image package using Composer:
arduino composer require intervention/image
After installation, the package’s service provider should be automatically registered. You can start using the Intervention Image library in your code:
php use Intervention\Image\Facades\Image; // Load an image $image = Image::make('path/to/image.jpg'); // Resize the image $image->resize(400, 300); // Apply a filter $image->filter(new \Intervention\Image\Filters\Grayscale()); // Save the modified image $image->save('path/to/new-image.jpg');
By utilizing the Intervention Image package, you can easily manipulate and enhance images in your Laravel applications.
Laravel Scout
Laravel Scout is a full-text search package that provides a simple and elegant way to add search functionality to your Laravel applications. It supports various search engines, including Algolia, Elasticsearch, and Meilisearch. Let’s integrate Laravel Scout with Algolia:
Install the Laravel Scout and Algolia packages using Composer:
bash composer require laravel/scout composer require algolia/algoliasearch-client-php
After installation, register the Laravel Scout service provider in your config/app.php file:
php 'providers' => [ // Other service providers... Laravel\Scout\ScoutServiceProvider::class, ],
Next, configure your Algolia credentials in the .env file:
makefile SCOUT_DRIVER=algolia ALGOLIA_APP_ID=your-app-id ALGOLIA_SECRET=your-app-secret ALGOLIA_SEARCH=your-search-key
Replace the placeholders with your actual Algolia credentials.
Now, let’s create a searchable model:
php use Illuminate\Database\Eloquent\Model; use Laravel\Scout\Searchable; class Product extends Model { use Searchable; // Define searchable fields public function toSearchableArray() { return [ 'name' => $this->name, 'description' => $this->description, ]; } }
Once you’ve created the searchable model, you can perform searches:
php use App\Models\Product; $products = Product::search('keyword')->get();
Laravel Scout simplifies the process of adding powerful search capabilities to your Laravel applications.
Conclusion
Laravel packages offer a convenient way to extend the functionality of your applications with third-party libraries. In this blog post, we explored the basics of Laravel packages, including their installation process. We also showcased the integration of two popular libraries, Intervention Image for image manipulation and Laravel Scout for full-text search.
By leveraging Laravel packages, you can save development time, increase productivity, and benefit from the collective efforts of the Laravel community. So, explore the vast ecosystem of Laravel packages, and unlock new possibilities for your applications!
Remember, while integrating packages, always ensure they are actively maintained and compatible with your Laravel version to avoid compatibility issues.
Table of Contents
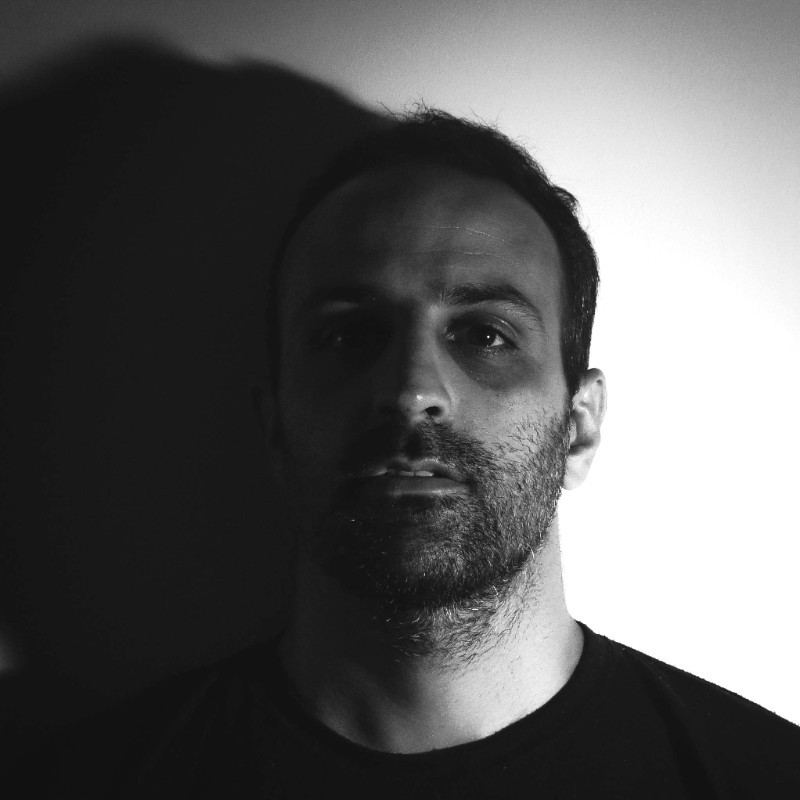
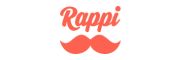