Laravel Queues: Managing Background Jobs and Task Processing
In today’s world of web applications, user experience and performance are paramount. As users interact with web applications, tasks such as sending emails, generating reports, or processing large data sets can put a strain on the application’s responsiveness. To tackle this challenge, Laravel provides a robust queue system that allows you to offload time-consuming tasks to background workers, ensuring your application remains snappy and responsive.
In this blog post, we will explore Laravel Queues and discover how they can be used to manage background jobs and task processing in your application. We’ll cover the fundamentals of queues, explain different queue drivers, demonstrate how to define jobs, and showcase how to monitor and manage queues effectively.
Let’s dive in!
What are Laravel Queues?
Laravel Queues provide a way to defer the processing of a time-consuming task to a later time. Instead of executing a task immediately, it is added to a queue and processed asynchronously in the background. This approach allows your application to respond quickly to user requests while ensuring that resource-intensive tasks are completed efficiently.
Queues in Laravel consist of three main components:
Jobs: A job represents a unit of work that needs to be processed. It encapsulates the task you want to perform asynchronously.
Queues: Queues act as containers for jobs, holding tasks that need to be processed.
Workers: Workers are responsible for fetching jobs from the queue and executing them.
The queue system is designed to be flexible, allowing you to choose from a variety of queue drivers depending on your specific needs.
Advantages of Using Laravel Queues
- Using Laravel Queues can bring several advantages to your application:Improved Performance: By offloading time-consuming tasks to background workers, your application can remain responsive and handle user requests promptly.
- Scalability: Queues allow you to distribute the workload across multiple workers, enabling your application to handle a large number of tasks simultaneously.
- Fault Tolerance: If an error occurs during the processing of a job, Laravel’s queue system provides a way to retry failed jobs automatically, ensuring that no tasks go unnoticed or unprocessed.
- Task Prioritization: With queues, you can assign different priorities to jobs, ensuring that critical tasks are processed before less important ones.
Configuring Queue Drivers
Laravel supports a variety of queue drivers out of the box, including:
- Database: Stores jobs in a database table.
- Beanstalkd: Uses the Beanstalkd queue service.
- Amazon SQS: Integrates with the Amazon Simple Queue Service.
- Redis: Utilizes Redis as the queue driver.
- Null: Discards jobs without actually processing them (useful for testing).
To configure the queue driver, you can modify the QUEUE_CONNECTION variable in the .env file of your Laravel application:
dotenv QUEUE_CONNECTION=redis
You can also configure additional driver-specific options, such as connection details or specific queues, by editing the config/queue.php file.
Creating Jobs in Laravel
In Laravel, jobs are classes that define the tasks to be performed in the background. Jobs are typically stored in the app/Jobs directory of your application.
Let’s create an example job called SendEmailJob that sends an email to a user:
php <?php namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; use Illuminate\Support\Facades\Mail; class SendEmailJob implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $user; public function __construct(User $user) { $this->user = $user; } public function handle() { // Code to send email to $this->user } }
The ShouldQueue interface indicates that the job should be pushed to the queue rather than executed immediately.
Dispatching Jobs to the Queue
Once you have defined a job, you can dispatch it to the queue using Laravel’s dispatching syntax. Dispatching a job is as simple as calling its dispatch method:
php use App\Jobs\SendEmailJob; use App\User; $user = User::find(1); SendEmailJob::dispatch($user);
In this example, we find a user with ID 1 and dispatch the SendEmailJob with the user as its parameter. The job is then added to the default queue for processing.
Running Queue Workers
To start processing jobs in the queue, you need to run one or more queue workers. Queue workers are responsible for fetching jobs from the queue and executing them.
To run the queue worker, you can use the queue:work Artisan command:
shell php artisan queue:work --queue=default
This command instructs Laravel to start a worker that will process jobs from the default queue. You can specify multiple queues to be processed by separating them with commas.
Monitoring and Managing Queues
Laravel provides various tools to monitor and manage queues effectively. The Horizon package offers a beautiful dashboard to monitor queues, track job statuses, and configure various queue settings. It allows you to monitor the health of your queues and gain insights into their performance.
Additionally, Laravel provides commands such as queue:retry, queue:failed, and queue:flush that allow you to retry failed jobs, view failed jobs, and flush all jobs from the queue, respectively.
Conclusion
Laravel Queues provide a powerful mechanism for managing background jobs and processing tasks asynchronously. By offloading time-consuming tasks to queues, your application can maintain responsiveness, handle high traffic, and improve user experience. With the ability to choose from various queue drivers and a range of built-in features for managing queues, Laravel empowers developers to build scalable and efficient applications.
In this blog post, we explored the fundamentals of Laravel Queues, discussed the advantages they bring to your application, and demonstrated how to define jobs, dispatch them to the queue, and process them using queue workers. We also touched upon monitoring and managing queues effectively.
By incorporating Laravel Queues into your development workflow, you can enhance your application’s performance, scalability, and fault tolerance, ensuring a smooth user experience even under heavy workloads.
Table of Contents
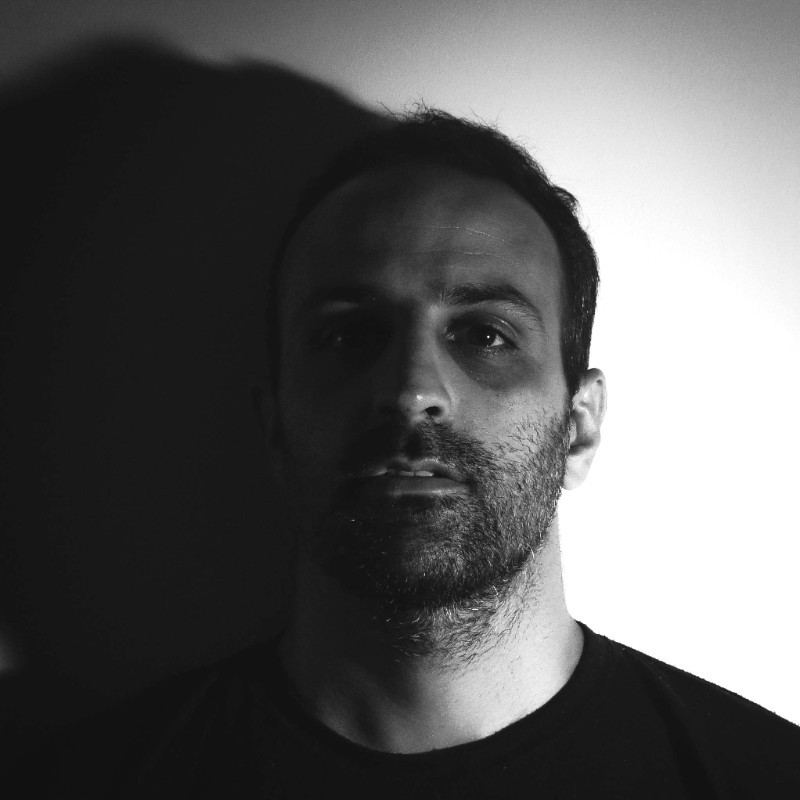
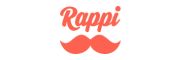