How to define relationships in Eloquent?
Defining relationships in Eloquent, the ORM (Object-Relational Mapping) of Laravel, is like telling a story of how different parts of your database connect and interact with each other. These relationships help you build rich, interconnected data models in your Laravel applications. Here’s how you can define relationships in Eloquent in a human-friendly way:
Imagine you have two database tables: users and posts. Each user can have multiple posts, creating a relationship between the two tables. In Eloquent, you define this relationship using simple, expressive syntax within your model classes.
To establish this relationship, first, you need to define the relationship in the User model class. You’ll use the hasMany method to indicate that a user can have multiple posts. Here’s how you’d define the relationship:
php class User extends Model { public function posts() { return $this->hasMany(Post::class); } }
In this code snippet, the hasMany method tells Laravel that each user can have multiple posts. The Post::class argument specifies the related model class, indicating that the posts method will return a collection of Post model instances associated with the user.
Next, you define the inverse relationship in the Post model class. Since each post belongs to a single user, you’ll use the belongsTo method to establish this relationship:
php class Post extends Model { public function user() { return $this->belongsTo(User::class); } }
Here, the belongsTo method indicates that each post belongs to a single user. The User::class argument specifies the related model class, indicating that the user method will return the User model instance associated with the post.
Once you’ve defined these relationships, you can effortlessly retrieve related data using Eloquent’s intuitive syntax. For example, to fetch all posts belonging to a specific user, you can simply call the posts method on the User model instance:
php $user = User::find(1); $posts = $user->posts; Similarly, to retrieve the user associated with a particular post, you can access the user property on the Post model instance: php $post = Post::find(1); $user = $post->user;
Defining relationships in Eloquent is about describing how different parts of your database connect and interact with each other. By defining these relationships in your model classes, you unlock the power of Eloquent’s intuitive syntax for fetching related data and building rich, interconnected data models in your Laravel applications.
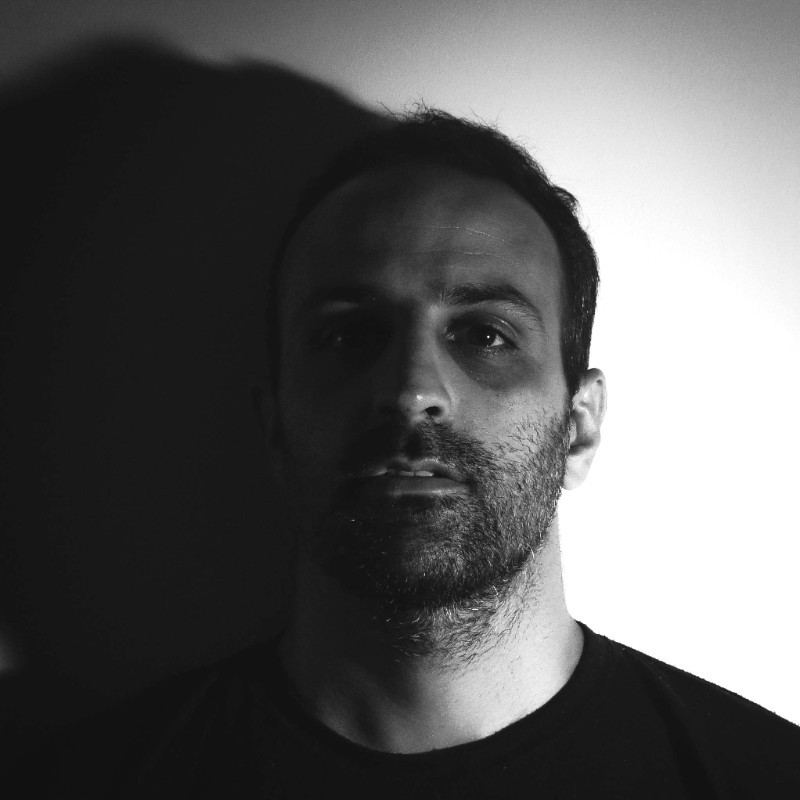
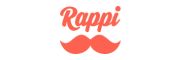