Building a Restful API with Laravel and JWT Authentication
In the ever-evolving world of web development, building efficient and secure APIs has become a crucial aspect. Laravel, a popular PHP framework, offers a seamless way to construct RESTful APIs while ensuring enhanced security through JWT (JSON Web Token) authentication. In this guide, we will walk you through the process of creating a RESTful API using Laravel and implementing JWT authentication, empowering you to develop powerful and secure APIs for your applications.
Table of Contents
1. Understanding RESTful APIs
1.1. What is a RESTful API?
A RESTful API (Representational State Transfer) is a set of architectural principles that define a scalable and stateless approach to creating web services. It allows different software applications to communicate with each other over the HTTP protocol using standard HTTP methods, such as GET, POST, PUT, and DELETE.
1.2. Why use RESTful APIs?
RESTful APIs provide a structured and standardized way for applications to interact with each other. They promote loose coupling between the client and server, allowing for easier scalability and maintenance. RESTful APIs are platform-independent, making them suitable for various types of clients, such as web browsers, mobile apps, and other servers.
1.3. Principles of RESTful architecture
- Stateless: Each request from a client to the server must contain all the information required to understand and process the request. The server should not store any client state between requests.
- Uniform Interface: RESTful APIs follow a uniform interface consisting of resources, HTTP methods, representations, and hypermedia. This uniformity simplifies communication and ensures consistency.
- Client-Server: The client and server are separate entities, allowing them to evolve independently without affecting each other.
- Cacheable: Responses from the server can be cached to improve performance and reduce the need for repeated requests.
- Layered System: A client can interact with a RESTful API through multiple layers, such as proxy servers or load balancers, without being aware of the underlying complexity.
2. Getting Started with Laravel
2.1. Installation and setup
Before we dive into building the API, make sure you have PHP and Composer installed on your system. Then, you can install Laravel using Composer with the following command:
bash composer global require laravel/installer
2.2. Creating a new Laravel project
Once Laravel is installed, you can create a new project using the following command:
bash laravel new YourProjectName
This command will set up a fresh Laravel installation in the specified directory.
2.3. Project structure overview
Laravel follows the Model-View-Controller (MVC) architectural pattern. The key directories you’ll interact with include:
- app: Contains your application’s models, controllers, and other core files.
- routes: Defines the routes that map to your API endpoints.
- database: Manages database migrations and seeds.
- config: Holds configuration files for your application.
- resources: Contains views, assets, and other frontend-related files.
- tests: Houses your application’s test suite.
3. Designing the API
3.1. Defining endpoints and resources
A well-designed API requires careful consideration of endpoints and resources. Each endpoint corresponds to a specific resource, and it should follow a consistent naming convention and structure. For example, to manage users, you might have endpoints like /api/users and /api/users/{id}.
3.2. Creating routes and controllers
In the routes/api.php file, you can define routes for your API endpoints using the Laravel routing system. For example:
php Route::get('/users', 'UserController@index'); Route::get('/users/{id}', 'UserController@show'); Route::post('/users', 'UserController@store'); Route::put('/users/{id}', 'UserController@update'); Route::delete('/users/{id}', 'UserController@destroy');
These routes map to the corresponding methods in the UserController controller.
3.3. Implementing CRUD operations
Inside the UserController controller, you can define methods to handle CRUD (Create, Read, Update, Delete) operations:
php public function index() { // Retrieve and return all users } public function show($id) { // Retrieve and return a specific user } public function store(Request $request) { // Create a new user } public function update(Request $request, $id) { // Update a user } public function destroy($id) { // Delete a user }
These methods will handle requests to the corresponding API endpoints.
4. JWT Authentication
4.1. What is JWT?
JSON Web Token (JWT) is a compact and self-contained way to transmit information between parties as a JSON object. It’s commonly used for authentication and authorization purposes. A JWT consists of three parts: header, payload, and signature.
4.2. Why use JWT for authentication?
JWT offers several benefits for authentication in APIs:
- Stateless: As JWTs contain all the necessary information, the server doesn’t need to maintain session state.
- Scalable: JWTs can be distributed to multiple servers or services, allowing for better scalability.
- Security: JWTs can be digitally signed, ensuring data integrity and authentication.
- Cross-Domain: JWTs can be easily shared across different domains or platforms.
4.3. Installing and configuring tymon/jwt-auth package
To implement JWT authentication in Laravel, we can use the tymon/jwt-auth package. Install it using Composer:
bash composer require tymon/jwt-auth
Next, publish the package’s configuration:
bash php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
This command will generate a config/jwt.php configuration file.
5. Setting Up User Authentication
5.1. Creating the User model
Laravel provides an Eloquent ORM for working with databases. Create the User model using the following command:
bash php artisan make:model User -m
This command generates a User model along with a migration file.
5.2. Registration and validation
Inside the migration file, define the user table schema with necessary fields like name, email, and password. After migrating the database using php artisan migrate, you can implement user registration and validation in the UserController:
php public function register(Request $request) { // Validate the input $this->validate($request, [ 'name' => 'required|string', 'email' => 'required|email|unique:users', 'password' => 'required|string|min:6', ]); // Create and store the new user }
5.3. User login functionality
Implement user login functionality by verifying the credentials and generating a JWT token:
php public function login(Request $request) { // Validate the input $credentials = $request->only('email', 'password'); // Verify credentials and generate JWT token }
6. Generating JWT Tokens
6.1. Issuing JWT tokens upon successful login
In the login method, after verifying the user’s credentials, you can issue a JWT token using the jwt-auth package:
php if (! $token = auth()->attempt($credentials)) { return response()->json(['error' => 'Unauthorized'], 401); } return $this->respondWithToken($token);
6.2. Customizing token payload
You can customize the token payload to include additional data when generating the token. This is useful for storing user-specific information:
php $customClaims = ['user_id' => auth()->user()->id]; $token = auth()->claims($customClaims)->attempt($credentials);
6.3. Token expiration and refresh
JWT tokens have a defined expiration time to enhance security. You can configure the token expiration time in the config/jwt.php file:
php 'exp' => Carbon::now()->addDays(7)->timestamp,
Tokens can also be refreshed using a refresh token mechanism to avoid forcing users to log in frequently:
php $refreshedToken = auth()->refresh();
7. Securing API Endpoints
7.1. Middleware and authorization
To secure your API endpoints with JWT authentication, you can use middleware provided by the jwt-auth package. In your routes, apply the auth:api middleware:
php Route::group(['middleware' => ['auth:api']], function () { // Your secured routes here });
7.2. Protecting routes with JWT authentication
With the auth:api middleware applied, only authenticated users with valid JWT tokens can access the protected routes.
7.3. Handling unauthorized access
When a request doesn’t have a valid JWT token, the auth:api middleware will automatically return a 401 Unauthorized response.
8. Testing the API
8.1. Using tools like Postman
Postman is a popular tool for testing APIs. You can use it to make requests to your API endpoints and inspect the responses.
8.2. Making API requests
In Postman, create a new request and specify the API endpoint along with the HTTP method (GET, POST, PUT, DELETE). You can also provide any required parameters or request bodies.
8.3. Validating responses
After making a request, Postman will display the response, including status codes, headers, and the JSON body. Ensure that the responses match your expectations and that error handling is working as intended.
9. Error Handling and Validation
9.1. Implementing validation rules
Laravel provides a powerful validation system to ensure the correctness of incoming data. You can define validation rules for request parameters and bodies in your controller methods:
php $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|email|unique:users', 'password' => 'required|string|min:6', ]);
9.2. Handling errors gracefully
When validation fails or other errors occur, it’s essential to return meaningful error responses. You can use Laravel’s response() function to send appropriate error messages along with corresponding status codes.
9.3. Returning meaningful error responses
php return response()->json(['error' => 'Validation failed'], 422);
10. Best Practices for Building APIs
10.1. Versioning your API
As APIs evolve, it’s crucial to maintain backward compatibility for existing clients. Versioning your API allows you to introduce changes without breaking existing functionality.
10.2. Using resource transformers
Resource transformers allow you to control the structure and formatting of API responses. Laravel’s built-in resource classes provide a convenient way to transform data before sending it to clients.
10.3. Pagination and sorting
For endpoints that return multiple records, implement pagination to limit the number of results returned per request. Additionally, allow clients to sort results based on different criteria.
Conclusion
Building a RESTful API with Laravel and JWT authentication empowers you to create secure and efficient communication channels between your applications. By following the principles of RESTful architecture, setting up user authentication, and using JWT for secure communication, you can develop powerful APIs that provide seamless experiences for your users. Laravel’s robust ecosystem, combined with JWT authentication, ensures that your API is not only functional but also secure and scalable for your application’s growth. With the knowledge gained from this guide, you’re well-equipped to embark on your journey of building modern, secure, and feature-rich APIs using Laravel.
Table of Contents
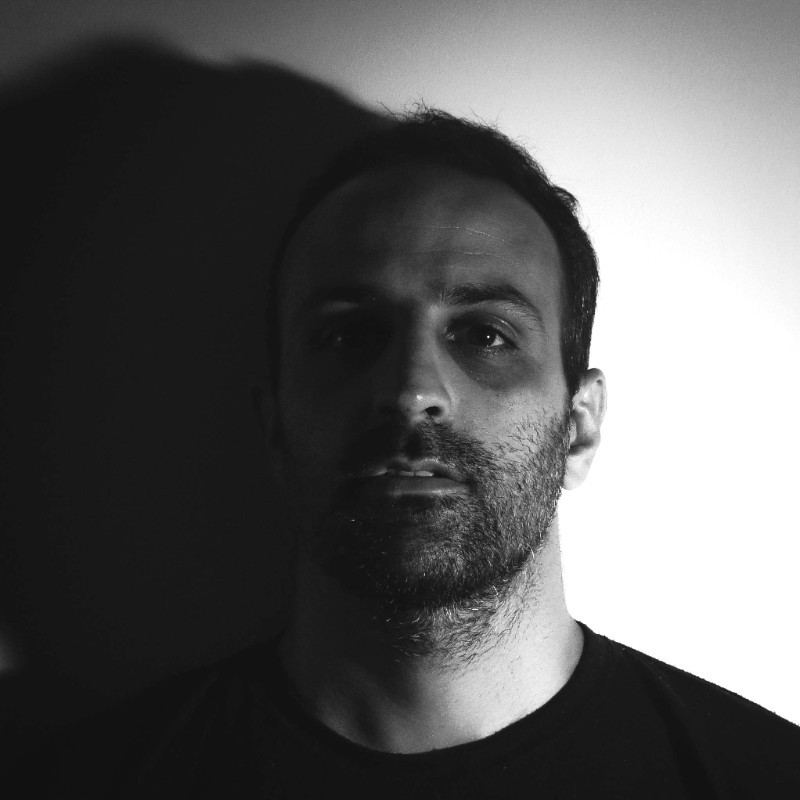
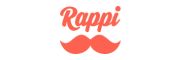