Laravel Notifications Uncovered: A Tutorial on Sending User Notifications
Laravel is a robust framework for web application development, appreciated for its elegant and expressive syntax. Among its numerous features, Laravel provides a unified API for various notification channels, making it incredibly convenient to send notifications through multiple channels such as email, SMS, Slack, and many more. This kind of functionality can greatly simplify the work of your in-house team or the Laravel developers you hire.
In this blog post, we will dive deep into Laravel Notifications. We’ll demonstrate how to send notifications to users, complete with practical examples. This knowledge is not only crucial for those who develop with Laravel themselves but also for those who plan to hire Laravel developers. Understanding the core functionalities of Laravel, like its Notifications feature, will help you to communicate your project requirements more effectively. So, let’s dive in and explore what Laravel Notifications has to offer!
c
Introduction to Laravel Notifications
Laravel Notifications are part of the framework that helps you send instant updates or messages to your users through various channels. It’s an excellent way to keep your users informed about updates, transactions, inquiries, etc. Laravel makes it extremely easy to send notifications via different channels like mail, database, broadcast, SMS, etc.
Setting Up Laravel Notifications
Before diving into examples, let’s make sure your Laravel environment is set up correctly. Firstly, ensure you have Laravel installed, and your application is up and running. Laravel Notifications system requires you to have a user model (or any model that can receive notifications) that is an instance of the `Notifiable` trait. This trait is included by default in the User model.
```php namespace App\Models; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; class User extends Authenticatable { use Notifiable; } ```
Now that we have our environment set up let’s explore creating and sending notifications.
Creating a Notification
To create a notification, we will use Laravel’s Artisan command-line tool:
```bash php artisan make:notification InvoicePaid ```
This command will create a notification class in the `app/Notifications` directory. Each notification class contains a `via` method and a variable number of message building methods (such as `toMail` or `toDatabase`) that convert the notification to a message optimized for that particular channel.
```php namespace App\Notifications; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Notification; class InvoicePaid extends Notification { use Queueable; public function via($notifiable) { return ['mail']; } public function toMail($notifiable) { return (new MailMessage) ->line('The introduction to the notification.') ->action('Notification Action', url('/')) ->line('Thank you for using our application!'); } } ```
Sending Notifications
Now that our notification class is set up let’s send our first notification. Laravel makes it super easy to send notifications. You can send a notification via the `notify` method on any Laravel model that uses the `Notifiable` trait.
```php use App\Models\User; use App\Notifications\InvoicePaid; $user = User::first(); // Notify the user $user->notify(new InvoicePaid($invoice)); ```
In this example, we’re sending the `InvoicePaid` notification to the first user. The `$invoice` is the invoice instance which would be passed to the notification class. The `notify` method will automatically determine how the notification should be sent based on the `via` method of the notification class.
Notification Via Email
The `toMail` method of the notification class is responsible for sending notifications via email. The method receives a `$notifiable` entity which should be an instance of the class that has the `Notifiable` trait. In our example, it is a `User` instance.
```php public function toMail($notifiable) { return (new MailMessage) ->subject('Invoice Paid Successfully') ->greeting('Hello, ' . $notifiable->name) ->line('Your invoice has been paid successfully.') ->action('View Invoice', url('/invoices/'.$this->invoice->id)) ->line('Thank you for using our application!'); } ```
Database Notifications
To store notifications in the database, we need to make a migration for the notifications table. Laravel provides a schema for this table that can be generated using the command:
```bash php artisan notifications:table php artisan migrate ```
After running these commands, a `notifications` table will be created in your database. Now, you can add `database` to the `via` method to instruct Laravel to store notifications in the database.
```php public function via($notifiable) { return ['database']; } public function toDatabase($notifiable) { return [ 'amount' => $this->invoice->amount, 'invoice_action' => 'Paid' ]; } ```
In this example, `toDatabase` method returns an array of data that will be stored as JSON in the `data` column of your `notifications` table.
Retrieving notifications from the database is also quite simple:
```php $user = User::find(1); foreach ($user->notifications as $notification) { echo $notification->type; } ```
Conclusion
The Laravel Notifications system is a flexible and convenient feature to send updates and messages to your users through various channels. It simplifies the complexity of managing different notification channels, allowing developers to focus on the core functionalities. It’s just another shining example of how Laravel continues to make developers’ lives easier.
Whether you’re working on your project or you decide to hire Laravel developers, understanding and implementing the Laravel Notifications system can streamline your workflow. Its flexibility allows you to customize it to fit the specific requirements of your project, including adding more channels, customizing notification messages, or even queuing notifications for background sending.
The possibilities are endless with Laravel at your fingertips! By understanding the principles and examples covered in this blog post, you’re well on your way to mastering Laravel Notifications. This knowledge can be highly beneficial, whether you’re a developer, or you’re seeking to communicate effectively with your team of Laravel developers.
Now, it’s your turn to put this knowledge into practice in your Laravel projects. Happy coding!
Table of Contents
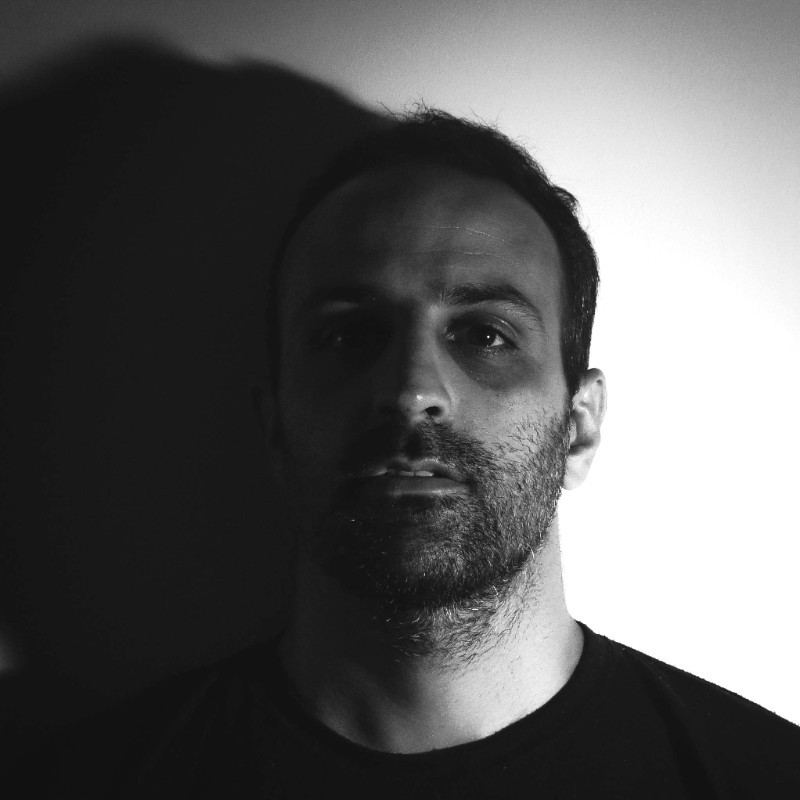
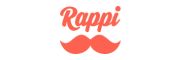