Achieve Streamlined User Logins in Laravel with Facebook, Twitter & More!
In the age of interconnected applications, enabling users to login with their preferred social networks has become an essential feature. It saves time, enhances the user experience, and can even increase sign-ups. Laravel, a popular PHP web application framework, offers a neat package called Socialite to make this process smooth. In this article, we’ll walk you through the steps to integrate social login in a Laravel application using Socialite.
1. Prerequisites
- Laravel project (v5.8 or above)
- Composer (for package management)
- Supported OAuth providers’ credentials (like Client ID and Client Secret)
Step 1: Install the Socialite Package
Run the following command to install Socialite via Composer:
```bash composer require laravel/socialite ```
Step 2: Configure Service Providers
After installation, register the `Laravel\Socialite\SocialiteServiceProvider` in the `providers` array of your `config/app.php`:
```php 'providers' => [ // ... Laravel\Socialite\SocialiteServiceProvider::class, ], ```
Also, add the `Socialite` facade to the `aliases` array:
```php 'aliases' => [ // ... 'Socialite' => Laravel\Socialite\Facades\Socialite::class, ], ```
Step 3: Set up OAuth Credentials
For each social network, you’ll need to retrieve the app’s credentials. This typically includes a `Client ID` and a `Client Secret`. Once retrieved, add them to your `.env` file:
For Facebook, as an example:
```env FACEBOOK_CLIENT_ID=your_facebook_client_id FACEBOOK_CLIENT_SECRET=your_facebook_client_secret FACEBOOK_REDIRECT_URL=http://your-domain/callback/facebook ```
Repeat this process for each provider you plan to support.
Now, configure these services in `config/services.php`:
```php 'facebook' => [ 'client_id' => env('FACEBOOK_CLIENT_ID'), 'client_secret' => env('FACEBOOK_CLIENT_SECRET'), 'redirect' => env('FACEBOOK_REDIRECT_URL'), ], // Add similar configurations for other providers... ```
Step 4: Implement Routes and Controllers
First, let’s set up routes in `routes/web.php`:
```php Route::get('login/{provider}', 'Auth\LoginController@redirectToProvider'); Route::get('callback/{provider}', 'Auth\LoginController@handleProviderCallback'); ```
In the `LoginController`, implement the `redirectToProvider` and `handleProviderCallback` methods:
```php use Socialite; public function redirectToProvider($provider) { return Socialite::driver($provider)->redirect(); } public function handleProviderCallback($provider) { $user = Socialite::driver($provider)->user(); // Handle user login or registration logic here... return redirect()->intended('home'); } ```
Step 5: Add Social Login Buttons
In your login view, you can add buttons or links that redirect to the appropriate route:
```html <a href="{{ url('login/facebook') }}" class="btn btn-primary">Login with Facebook</a> <!-- Add similar buttons for other providers... --> ```
Example: Integrating More Providers
Let’s integrate Twitter:
- Register your app on the [Twitter Developer Console](https://developer.twitter.com/) and retrieve your credentials.
- Add them to `.env`:
```env TWITTER_CLIENT_ID=your_twitter_client_id TWITTER_CLIENT_SECRET=your_twitter_client_secret TWITTER_REDIRECT_URL=http://your-domain/callback/twitter ```
- Add the configuration in `config/services.php`:
```php 'twitter' => [ 'client_id' => env('TWITTER_CLIENT_ID'), 'client_secret' => env('TWITTER_CLIENT_SECRET'), 'redirect' => env('TWITTER_REDIRECT_URL'), ], ```
- Add a login button:
```html <a href="{{ url('login/twitter') }}" class="btn btn-info">Login with Twitter</a> ```
Conclusion
Integrating social logins using Socialite in Laravel is a straightforward process, making it easier for users to log in with their preferred social networks. With support for a wide range of OAuth providers, you can cater to a diverse user base. By enhancing user experience with such features, you’re likely to see an increase in user registrations and engagement. So, go ahead, integrate, and let your users enjoy a seamless login experience!
Table of Contents
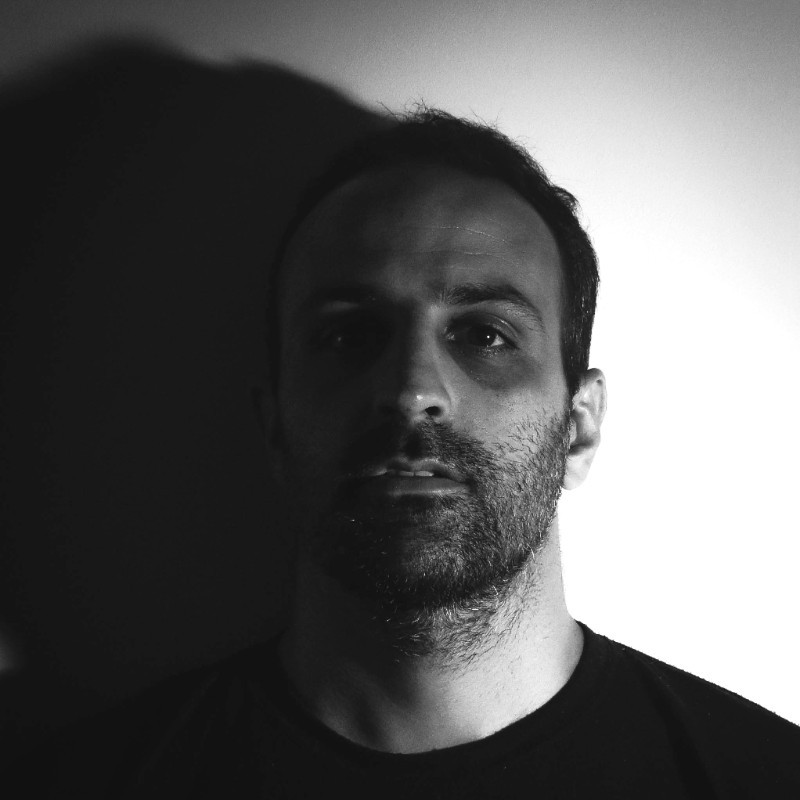
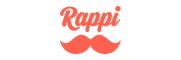