Master Subscription Billing in Laravel Applications with Laravel Cashier
Subscription billing, a common business model in web applications, often presents challenges in managing subscriptions, payment plans, and billing. An elegant solution exists in the Laravel framework through Laravel Cashier. This comprehensive library aids in managing subscription billing services, adeptly handling aspects like single charges, coupons, invoices, refunds, and more.
Table of Contents
When you hire Laravel developers, they can utilize Laravel Cashier’s fluent and expressive interface with payment gateways such as Stripe and Paddle to simplify and implement a subscription model in your application. In this post, we explore the world of Laravel Cashier and how it streamlines subscription billing, supported by practical examples.
1. Setting Up Laravel Cashier
The first step in using Laravel Cashier is installation and setup. It can be installed via Composer, a PHP dependency management tool:
```bash composer require laravel/cashier ```
Next, you will need to add the `Billable` trait to your model definition:
```php use Laravel\Cashier\Billable; class User extends Authenticatable { use Billable; } ```
This `Billable` trait adds various methods to the model, allowing it to handle subscriptions, invoices, card updates, etc. For proper functioning, you will need to add several columns to your database. Laravel Cashier comes with prebuilt migrations for this:
```bash php artisan cashier:table users php artisan migrate ```
You will also need to set up API keys for the payment gateway in your `.env` file:
```bash STRIPE_KEY=your-stripe-key STRIPE_SECRET=your-stripe-secret ```
2. Creating Subscriptions
Once setup is complete, creating subscriptions is straightforward. Let’s say we want to create a new subscription for a user. Here is how you would do it:
```php $user = User::find(1); $user->newSubscription('main', 'premium')->create($creditCardToken); ```
In the above example, ‘main’ is the name of the subscription, and ‘premium’ is the specific plan. The `$creditCardToken` would come from your payment gateway’s JavaScript library on the front end.
3. Checking Subscription Status
After the subscription is created, you might want to check the subscription status. Laravel Cashier makes this incredibly simple:
```php if ($user->subscription('main')->active()) { // User is on the 'main' subscription and the subscription is active... } ```
This makes it easy to control access to various parts of your application based on the subscription status.
4. Handling Subscription Trials
One common feature in subscription models is offering trials to new users. Laravel Cashier offers built-in support for this:
```php $user->newSubscription('main', 'premium')->trialDays(10)->create($creditCardToken); ```
The `trialDays()` method sets the trial period for the new subscription. Checking if a user is on trial is also simple:
```php if ($user->subscription('main')->onTrial()) { // User is on a trial... } ```
5. Swapping Subscriptions
In a flexible subscription model, users should be able to upgrade or downgrade their subscription plans. Laravel Cashier has a `swap()` method for this:
```php $user->subscription('main')->swap('premium-plus'); ```
In this example, the user’s ‘main’ subscription is swapped from the ‘premium’ plan to the ‘premium-plus’ plan.
6. Canceling Subscriptions
Canceling subscriptions is as easy as creating them:
```php $user->subscription('main')->cancel(); ```
You can also allow a user’s subscription to remain active until the end of the billing cycle with the `cancelAtEndOfPeriod()` method:
```php $user->subscription('main')->cancelAtEndOfPeriod(); ```
7. Single Charges
Laravel Cashier can also handle one-off charges:
```php $user->charge(1000, $creditCardToken); ```
In this example, the user is charged $10.00. The charge amount is in the smallest common currency unit (like cents for USD).
8. Invoices
Laravel Cashier can also generate invoices for charges and provide invoice PDFs:
```php foreach ($user->invoices() as $invoice) { echo $invoice->total(); } $pdf = $user->downloadInvoice($invoice->id, [ 'vendor' => 'Your Company', 'product' => 'Your Product', ]); ```
This flexibility allows for a complete, seamless billing system within your Laravel application.
Wrapping Up
Laravel Cashier stands as a powerful and elegant tool that expert Laravel developers utilize to manage subscription billing in Laravel applications. With a highly expressive API, it dramatically simplifies subscription management, allowing developers to focus on crafting their applications rather than grappling with complex billing details.
Hiring Laravel developers who are skilled in Laravel Cashier can greatly streamline the process of creating and managing subscriptions, checking subscription statuses, offering subscription trials, and swapping and canceling subscriptions.
By leveraging Laravel Cashier, you can ensure the smooth operation of your subscription services, thereby enhancing user satisfaction and enabling your focus to remain fixed on continually improving your application.
It’s essential to remember that Laravel Cashier is only a tool – it’s the Laravel developers you hire who harness this tool to its full potential, shaping it to craft truly amazing applications. Happy Coding!
Table of Contents
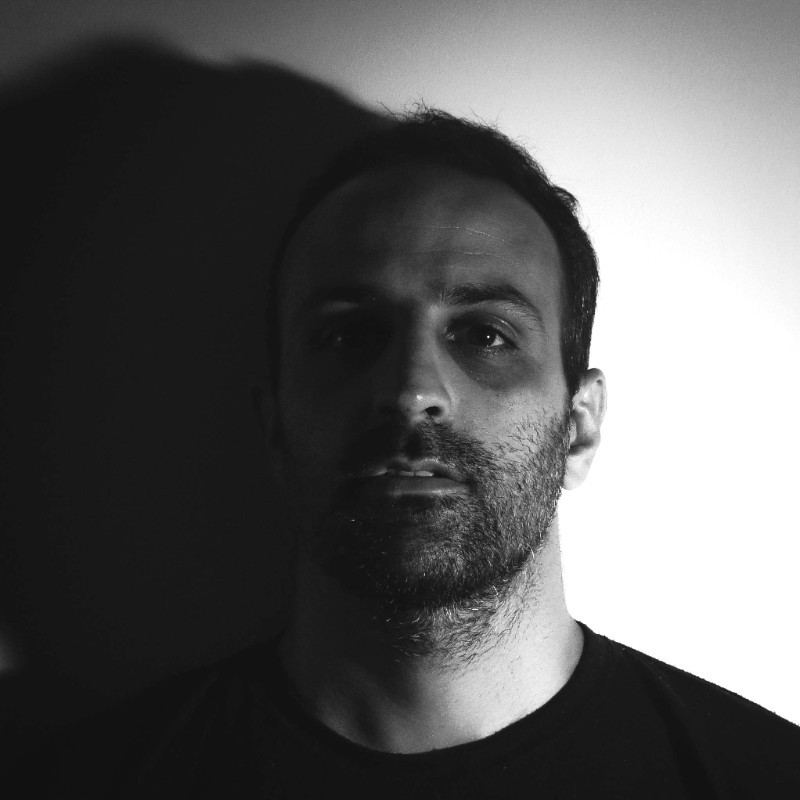
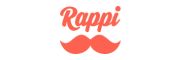