Laravel Dusk: The Ultimate Tool for Web Developers & Testers Alike
In the realm of web application development, one can never stress enough the importance of testing. Automated tests ensure that new changes don’t introduce regressions, thus maintaining the integrity of the application. Among the myriad of testing tools, Laravel Dusk stands out for its intuitive, browser-based testing capabilities. In this blog post, we’ll delve into Laravel Dusk, demonstrating its power for browser automation and testing.
1. What is Laravel Dusk?
Laravel Dusk provides an expressive, easy-to-use browser automation and testing API. Unlike the usual HTTP tests, Dusk tests use a real browser, which allows you to test JavaScript-driven applications. By offering a direct API into ChromeDriver and even support for other drivers, Dusk ensures your application works flawlessly in real-life browsers.
1.1 Setting Up Laravel Dusk
Before diving into the code, let’s set up Laravel Dusk:
- Install it via composer:
```bash composer require --dev laravel/dusk ```
- Next, register the `DuskServiceProvider`:
```php // app/Providers/AppServiceProvider.php use Laravel\Dusk\DuskServiceProvider; public function register() { if ($this->app->environment('local', 'testing')) { $this->app->register(DuskServiceProvider::class); } } ```
- Lastly, run `php artisan dusk:install` to install Dusk and generate the scaffolding.
2. Writing Your First Dusk Test
Laravel Dusk’s tests are located in the `tests/Browser` directory. Let’s write a basic test to ensure the home page loads correctly:
```php // tests/Browser/ExampleTest.php use Laravel\Dusk\Browser; use Tests\DuskTestCase; class ExampleTest extends DuskTestCase { /** @test */ public function basicExample() { $this->browse(function (Browser $browser) { $browser->visit('/') ->assertSee('Laravel'); }); } } ```
Run the test with `php artisan dusk`, and Dusk will automatically handle starting a ChromeDriver instance and running the test.
3. Advanced Dusk Interactions
Now that you have a grasp of the basics, let’s delve deeper with more advanced interactions.
3.1. Form Interaction
For instance, if we want to test a login form:
```php $this->browse(function (Browser $browser) { $browser->visit('/login') ->type('email', 'taylor@example.com') ->type('password', 'secret') ->press('Login') ->assertPathIs('/dashboard'); }); ```
3.2. JavaScript Interactions
With Dusk, JavaScript interactions, like clicking Vue.js components, are a breeze:
```php $this->browse(function (Browser $browser) { $browser->visit('/notification') ->click('@notification-link') // Vue component identifier ->waitForText('Notification Loaded') ->assertSee('New Notification'); }); ```
3.3. Pages & Components
Leveraging the Page Object pattern, Dusk allows for expressive testing. Imagine having a `Login` page:
```php // tests/Browser/Pages/LoginPage.php class LoginPage extends Page { public function url() { return '/login'; } public function assert(Browser $browser) { $browser->assertPathIs($this->url()); } public function typeEmail(Browser $browser, $email) { $browser->type('email', $email); } public function typePassword(Browser $browser, $password) { $browser->type('password', $password); } } ```
In your test:
```php $this->browse(function (Browser $browser) { $browser->visit(new LoginPage) ->typeEmail('taylor@example.com') ->typePassword('secret') ->press('Login') ->assertPathIs('/dashboard'); }); ```
4. Continuous Integration with Dusk
An essential aspect of testing is integrating it into a continuous integration (CI) process. Thankfully, Dusk is compatible with popular CI tools like Travis CI and GitHub Actions. With the `–headless` flag, Dusk runs in environments without GUI.
Conclusion
Laravel Dusk offers an expressive way to perform browser-based testing. From basic interactions to advanced JavaScript-driven applications, Dusk has got you covered. Embrace Dusk to ensure your Laravel applications are robust, reliable, and error-free in a real-world browsing context. Happy testing!
Table of Contents
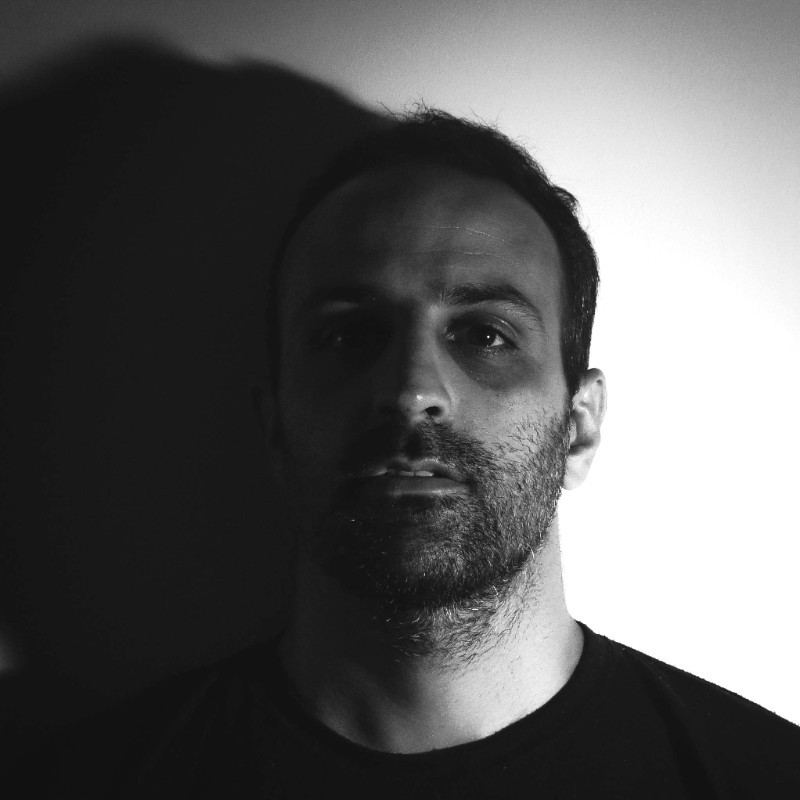
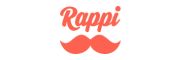