Laravel Authentication: Implementing User Authentication and Authorization
Laravel is one of the most popular PHP frameworks available today, known for its ease of use and comprehensive set of features. An important aspect of any web application built with Laravel is its authentication and authorization systems. These systems ensure that only authorized users have access to specific parts of the application, providing security and control over user access.
This makes it a top choice for businesses looking to hire Laravel developers for their web applications, as it simplifies the process of implementing robust security measures. This blog post will provide a step-by-step guide on how these Laravel developers implement user authentication and authorization in Laravel, thereby enhancing the security of your application.
Authentication
Authentication is the process of verifying a user’s identity. Laravel provides several ways to handle authentication, including the built-in Laravel UI and the newer Laravel Breeze and Laravel Jetstream packages. For this guide, we’ll use Laravel Breeze due to its simplicity and compatibility with Laravel 8.
Step 1: Installation
To begin, ensure you have a new Laravel project setup. After that, install Laravel Breeze using Composer:
```bash composer require laravel/breeze --dev
After the package is installed, use the `breeze:install` Artisan command to install it:
```bash php artisan breeze:install
This command will install authentication controllers, views, and routes for your application.
Finally, run:
```bash npm install && npm run dev
This command compiles your assets.
Step 2: Database Configuration
Before proceeding, ensure your database connection settings are correctly configured in your `.env` file.
Then, run the migrations using the Artisan command:
```bash php artisan migrate
This command will create the necessary tables in your database, including the `users` table.
Step 3: Using Authentication
With Breeze installed, Laravel automatically generates routes and views for registering and logging in. Users can register at `/register` and login at `/login`.
To check if a user is authenticated, use the `auth` middleware on your routes:
```php Route::get('/dashboard', function () { // Only authenticated users may enter... })->middleware('auth');
Authorization
Authorization is about determining what an authenticated user can and cannot access. Laravel’s primary way of handling authorization is through Policies.
Step 1: Creating Policies
For this guide, let’s say we have a `Post` model, and we want to authorize actions like creating, updating, and deleting posts.
To create a policy, use the `make:policy` Artisan command:
```bash php artisan make:policy PostPolicy --model=Post
This command creates a `PostPolicy` class in your `app/Policies` directory.
Step 2: Writing Authorization Logic
In your new `PostPolicy` class, methods corresponding to various actions can be added. These methods should return `true` if the user is authorized to perform the given action, and `false` otherwise.
Here’s an example for a `delete` method:
```php public function delete(User $user, Post $post) { return $user->id === $post->user_id; }
This checks if the logged-in user is the owner of the post.
Step 3: Authorizing Actions
Once your policies are set up, you can use them to authorize actions. You can do this within your controller methods:
```php public function destroy(Post $post) { $this->authorize('delete', $post); // The current user can delete the post... }
The `authorize` method reads the incoming HTTP request and retrieves the authenticated user from the session. Then, it determines if the user can delete the given post.
Step 4: Registering Policies
Lastly, your policy needs to be registered. This is typically done in the
`AuthServiceProvider`:
```php protected $policies = [ Post::class => PostPolicy::class, ];
By mapping the `Post` model to the `PostPolicy`, Laravel knows which policy to use when authorizing actions on `Post` instances.
Conclusion
Laravel’s authentication and authorization systems are robust and flexible, enabling you to handle complex access control scenarios with ease. This makes Laravel a top choice for those looking to hire Laravel developers, as their expertise can fully leverage these systems. While this guide covers the basics, Laravel’s documentation offers a more comprehensive overview, including advanced features like email verification, password resetting, and multi-authentication systems.
Embodying Laravel’s design philosophy of making the difficult easy and the common tasks enjoyable, Laravel developers can focus more on what makes your application unique and less on the security plumbing. Implementing authentication and authorization might be just a part of building an application, but with the skills of hired Laravel developers, this process becomes a breeze. Happy coding!
Table of Contents
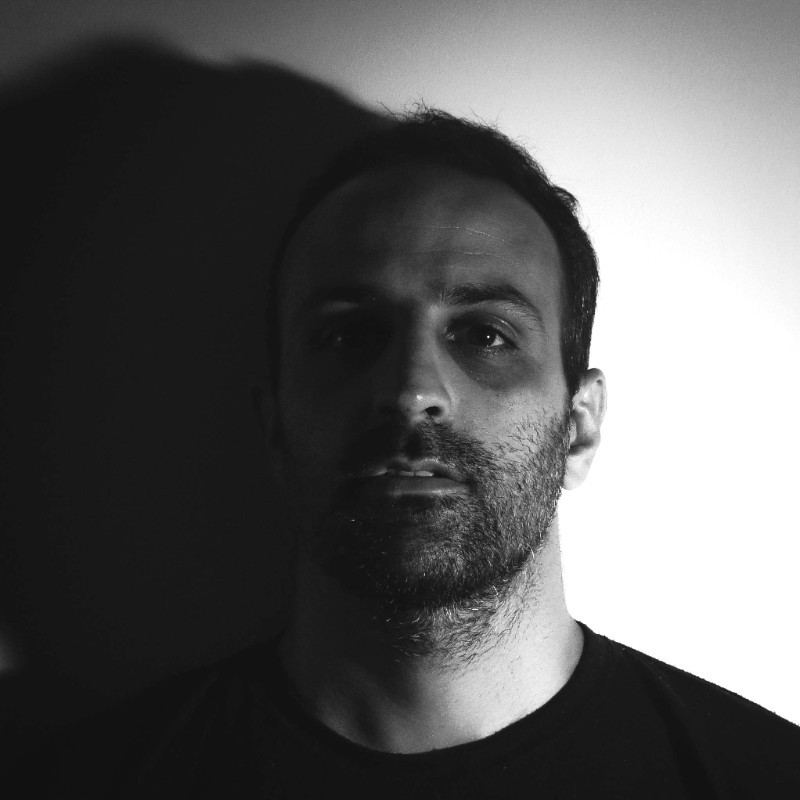
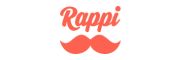