Meteor and Angular: Building Dynamic Single-Page Applications
In the world of modern web development, single-page applications (SPAs) have gained immense popularity due to their responsiveness and seamless user experience. Meteor and Angular, two cutting-edge technologies, come together to offer developers a powerful combination for building dynamic SPAs. This blog post will delve into the benefits of using Meteor and Angular together, walk you through the basics of each technology, and provide comprehensive code samples to demonstrate how they work in harmony. Whether you are a seasoned developer or just starting your journey in web development, this guide will equip you with the knowledge to create stunning SPAs.
1. Understanding Single-Page Applications (SPAs):
1.1 What are SPAs?
Single-Page Applications are web applications that load a single HTML page initially and dynamically update the content as the user interacts with the application. This approach reduces the need for full-page reloads, resulting in a smoother and more responsive user experience.
1.2 Advantages of SPAs:
SPAs offer numerous benefits, including faster page loads, reduced server requests, and a more engaging user interface. They also provide better support for building mobile-friendly applications and improved search engine optimization (SEO) through techniques like server-side rendering.
1.3 Challenges with SPAs:
Although SPAs provide a superior user experience, they come with their own set of challenges, such as handling navigation, managing state, and dealing with browser compatibility issues.
2. Introducing Meteor:
2.1 Meteor Overview:
Meteor is an open-source JavaScript platform for building real-time web and mobile applications. It provides a complete stack, including frontend, backend, and database, which simplifies the development process and allows developers to focus on building features rather than managing configurations.
2.2 Key Features of Meteor:
- Integrated Build System: Meteor includes a build system that automatically bundles and serves all your client and server-side code.
- Real-Time Data Communication: Meteor’s data synchronization capabilities allow real-time updates between clients and the server.
- Reactive UI: Meteor leverages reactive programming to automatically update the UI when underlying data changes.
- Full Stack Reactivity: The platform provides a seamless full-stack reactivity, ensuring consistent data updates across the application.
2.3 Setting up a Meteor Project:
To get started with Meteor, you’ll need to install Node.js and the Meteor CLI. Once installed, you can create a new Meteor project using the following command:
bash meteor create my-app cd my-app meteor
This will create a new Meteor project and start the development server.
3. Introduction to Angular:
3.1 Angular Overview:
Angular is a widely-used open-source frontend framework developed and maintained by Google. It simplifies the development of SPAs by providing powerful tools and abstractions for building complex user interfaces.
3.2 Key Features of Angular:
- Component-based Architecture: Angular applications are built using components, which are self-contained, reusable building blocks.
- Dependency Injection: Angular’s dependency injection system enables efficient management of application dependencies.
- Two-Way Data Binding: Angular offers two-way data binding, allowing automatic synchronization of data between the model and the view.
- Directives: Directives in Angular allow extending HTML with custom attributes and elements to create dynamic and interactive components.
3.3 Creating an Angular Project:
To create a new Angular project, you need to have Node.js and Angular CLI installed. Use the following commands to create and run a new Angular application:
bash npm install -g @angular/cli ng new my-angular-app cd my-angular-app ng serve
This will create a new Angular project and start the development server.
4. Integrating Meteor with Angular:
Integrating Meteor with Angular offers the best of both worlds: Meteor’s real-time capabilities and Angular’s powerful frontend framework. Let’s walk through the steps to set up this integration.
4.1 Installing Necessary Packages:
To integrate Meteor with Angular, we need to install the angular-meteor package, which provides the necessary tools and services to connect the two frameworks seamlessly. Use the following command to install the package:
bash meteor npm install --save angular-meteor
4.2 Creating a Real-Time Data Connection:
Meteor’s strength lies in its real-time data synchronization. We can leverage this capability in our Angular application by creating a connection to Meteor’s reactive data sources.
First, let’s import the angular-meteor module and create a Meteor connection in our Angular app’s main module (usually app.module.ts):
typescript import { NgModule } from '@angular/core'; import { Meteor } from 'meteor/meteor'; @NgModule({ // ... other module configurations ... }) export class AppModule { constructor() { Meteor.connect('ws://localhost:3000/websocket'); // Adjust the URL to your Meteor server } }
This establishes a WebSocket connection between Angular and Meteor, enabling real-time data exchange.
4.3 Handling Data Synchronization:
With the connection established, we can now use Meteor’s collections to handle data synchronization between the server and clients. Let’s create a sample collection in our Meteor server:
javascript // In your server/main.js or server/main.ts import { Meteor } from 'meteor/meteor'; import { Mongo } from 'meteor/mongo'; export const Tasks = new Mongo.Collection('tasks'); Meteor.publish('tasks', function () { return Tasks.find({}); });
This will create a collection named ‘tasks’ and publish all its data to the clients.
Now, on the Angular side, we can use angular-meteor to access this collection and reactively display the data in our components:
typescript import { Component } from '@angular/core'; import { Observable } from 'rxjs'; import { Tasks } from '/imports/api/tasks'; @Component({ selector: 'app-tasks-list', template: ` <ul> <li *ngFor="let task of tasks$ | async">{{ task.name }}</li> </ul> `, }) export class TasksListComponent { tasks$: Observable<any[]>; constructor() { this.tasks$ = Tasks.find({}).zone(); } }
The tasks$ variable represents the observable that will reactively update the tasks list in real-time as new data is added or modified in the ‘tasks’ collection.
5. Building a Real-Time Chat Application:
Let’s take our integration to the next level by building a real-time chat application using Meteor and Angular.
5.1 Designing the UI with Angular Components:
First, let’s design the chat interface using Angular components. We’ll have a chat window to display messages and an input field to send new messages:
html <!-- chat.component.html --> <div class="chat-container"> <div class="chat-window"> <div *ngFor="let message of messages">{{ message }}</div> </div> <input [(ngModel)]="newMessage" (keyup.enter)="sendMessage()" placeholder="Type your message..."> </div> typescript Copy code // chat.component.ts import { Component } from '@angular/core'; import { Meteor } from 'meteor/meteor'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', }) export class ChatComponent { messages: string[] = []; newMessage: string; sendMessage() { if (this.newMessage.trim() !== '') { this.messages.push(this.newMessage); this.newMessage = ''; } } }
In the example above, we have a simple chat window that displays messages in real-time as the user types new ones.
5.2 Implementing Real-Time Features with Meteor:
To make this chat application truly real-time, we need to integrate Meteor’s real-time capabilities. We’ll use the same ‘tasks’ collection we created earlier to store chat messages.
javascript // server/main.js or server/main.ts import { Meteor } from 'meteor/meteor'; import { Mongo } from 'meteor/mongo'; export const Messages = new Mongo.Collection('messages'); Meteor.publish('messages', function () { return Messages.find({}); });
On the Angular side, we can modify our chat component to use this collection to store and display messages:
typescript // chat.component.ts import { Component } from '@angular/core'; import { Meteor } from 'meteor/meteor'; import { Observable } from 'rxjs'; import { Messages } from '/imports/api/messages'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', }) export class ChatComponent { messages$: Observable<any[]>; newMessage: string; constructor() { this.messages$ = Messages.find({}).zone(); } sendMessage() { if (this.newMessage.trim() !== '') { Messages.insert({ text: this.newMessage }); this.newMessage = ''; } } }
With these changes, our chat application now stores messages in the ‘messages’ collection and automatically updates the UI for all connected clients when a new message is added.
5.3 Enhancing User Experience with Angular Animations:
To add some flair to our chat application, we can utilize Angular’s animations. Let’s add a simple animation to make the messages slide in from the right as they appear:
typescript // chat.component.ts import { Component } from '@angular/core'; import { Meteor } from 'meteor/meteor'; import { Observable } from 'rxjs'; import { Messages } from '/imports/api/messages'; import { animate, state, style, transition, trigger } from '@angular/animations'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', animations: [ trigger('messageAnimation', [ state('void', style({ transform: 'translateX(100%)' })), state('*', style({ transform: 'translateX(0)' })), transition('void <=> *', animate('300ms ease-in-out')), ]), ], }) export class ChatComponent { messages$: Observable<any[]>; newMessage: string; constructor() { this.messages$ = Messages.find({}).zone(); } sendMessage() { if (this.newMessage.trim() !== '') { Messages.insert({ text: this.newMessage }); this.newMessage = ''; } } }
Now, when a new message is added, it will smoothly slide into view from the right, enhancing the overall user experience.
6. Optimizing Performance:
Building SPAs requires careful consideration of performance to ensure a smooth user experience. Here are some performance optimization techniques for Meteor and Angular applications:
6.1 Minifying and Bundling Assets:
Minifying and bundling assets, such as CSS and JavaScript files, can significantly reduce the loading time of your application. Both Meteor and Angular offer built-in tools to perform these optimizations automatically during the build process.
6.2 Caching and Offline Capabilities:
Utilize caching strategies and Service Workers to enable offline capabilities for your SPA. This ensures that users can still access your application even when they are offline or experiencing slow internet connections.
6.3 Performance Tuning Tips:
- Optimize database queries to reduce data retrieval time.
- Lazy load components to reduce the initial load time.
- Use server-side rendering (SSR) to improve SEO and initial page load times.
Conclusion
Meteor and Angular together form a powerful duo for building dynamic single-page applications. Meteor’s real-time capabilities, combined with Angular’s robust frontend framework, enable developers to create seamless and engaging user experiences. In this blog post, we explored the basics of Meteor and Angular, integrated them to build a real-time chat application, and discussed performance optimization techniques. Armed with this knowledge and code samples, you are now well-equipped to embark on your journey to craft feature-rich, responsive, and real-time single-page applications. Happy coding!
Table of Contents
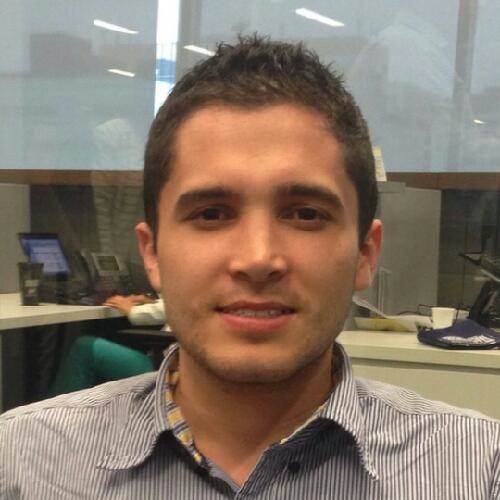
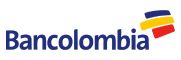