Exploring Meteor’s Templating Engine: Building Dynamic UIs
In today’s fast-paced world, web applications are becoming increasingly dynamic, with users expecting real-time updates and interactive interfaces. Meteor, a full-stack JavaScript framework, provides a powerful templating engine that enables developers to build dynamic UIs effortlessly. In this blog post, we will explore Meteor’s templating engine in depth, covering its core concepts, syntax, and code examples. By the end, you’ll be equipped with the knowledge to create engaging and interactive web applications using Meteor.
1. What is a Templating Engine?
A templating engine is a tool or framework that simplifies the process of generating dynamic HTML by combining data with predefined HTML templates. It provides a structured way to organize and render data-driven content on the client-side. Templating engines are essential for building modern web applications that require real-time updates and interactive elements.
2. Introducing Meteor
Meteor is a popular full-stack JavaScript framework that allows developers to build web and mobile applications using a single codebase. It provides an integrated development environment (IDE), a powerful templating engine, and a reactive data system, making it ideal for creating real-time applications. Meteor’s templating engine plays a crucial role in building dynamic UIs and enables developers to write concise and readable code.
3. Key Concepts of Meteor’s Templating Engine
Before diving into the syntax and examples, let’s explore some key concepts of Meteor’s templating engine.
3.1 Templates and Template Instances
In Meteor, a template is a reusable component that defines the structure and behavior of a specific part of the UI. Templates are defined using HTML-like syntax and can contain dynamic data and logic. Each template can have multiple instances, and each instance represents a specific occurrence of the template in the DOM.
3.2 Reactive Data Sources
Meteor’s templating engine leverages the reactivity concept, allowing templates to automatically update when their underlying data sources change. Reactive data sources can be created using the ReactiveVar or ReactiveDict classes, or by using reactive data sources provided by Meteor, such as Session.
3.3 Helpers
Helpers are functions associated with templates that provide dynamic data or perform calculations for rendering in the UI. They are defined inside the template definition block and can be accessed within the template using the {{helperName}} syntax. Helpers can be used to transform data, format dates, filter arrays, and much more.
3.4 Events
Events in Meteor’s templating engine enable developers to respond to user interactions, such as button clicks, form submissions, or mouse movements. Event handlers are defined inside the template definition block and can be associated with specific DOM elements or template instances. By utilizing events, developers can create interactive experiences by updating the UI or triggering server-side actions.
4. Syntax and Usage
Let’s now explore the syntax and usage of Meteor’s templating engine.
4.1 Template Definition
To define a template in Meteor, we use the Template object provided by the framework. The following code snippet demonstrates how to define a template named “exampleTemplate”:
javascript // HTML <template name="exampleTemplate"> <!-- Template content goes here --> </template> // JavaScript Template.exampleTemplate.helpers({ // Helpers go here }); Template.exampleTemplate.events({ // Events go here });
4.2 Template Helpers
Template helpers are defined inside the Template.exampleTemplate.helpers block. They are functions that return dynamic data or perform calculations for rendering in the UI. Here’s an example of a helper that retrieves and formats a user’s full name:
javascript Template.exampleTemplate.helpers({ fullName() { const user = Meteor.user(); if (user) { return `${user.firstName} ${user.lastName}`; } return ''; }, });
4.3 Template Events
Template events are defined inside the Template.exampleTemplate.events block. They allow you to specify event handlers for user interactions. Here’s an example of an event handler that logs a message when a button is clicked:
javascript Template.exampleTemplate.events({ 'click .my-button'(event, instance) { console.log('Button clicked!'); }, });
5. Building a Dynamic UI with Meteor’s Templating Engine
Now, let’s put everything together and build a dynamic UI using Meteor’s templating engine.
5.1 Creating a Reactive Data Source
First, we’ll create a reactive data source using ReactiveVar. This data source will hold a counter value that we can increment using a button.
javascript // JavaScript Template.exampleTemplate.onCreated(function () { this.counter = new ReactiveVar(0); }); // HTML <template name="exampleTemplate"> <h1>Counter: {{counter.get}}</h1> <button class="increment-button">Increment</button> </template>
5.2 Updating the UI with Helpers
Next, we’ll define a helper that retrieves the counter value and formats it for display in the UI.
javascript Template.exampleTemplate.helpers({ counter() { return Template.instance().counter.get(); }, });
5.3 Handling User Interactions with Events
Finally, we’ll define an event handler that increments the counter value when the button is clicked.
javascript Template.exampleTemplate.events({ 'click .increment-button'(event, instance) { const counter = instance.counter.get(); instance.counter.set(counter + 1); }, });
With these changes, our UI will display the counter value, and each click on the button will increment it.
Conclusion
Meteor’s templating engine provides a powerful and intuitive way to build dynamic UIs for web applications. By leveraging templates, helpers, and events, developers can create interactive experiences with ease. In this blog post, we explored the key concepts, syntax, and examples of using Meteor’s templating engine. Armed with this knowledge, you are now ready to create engaging and interactive web applications using Meteor’s templating engine. Happy coding!
Table of Contents
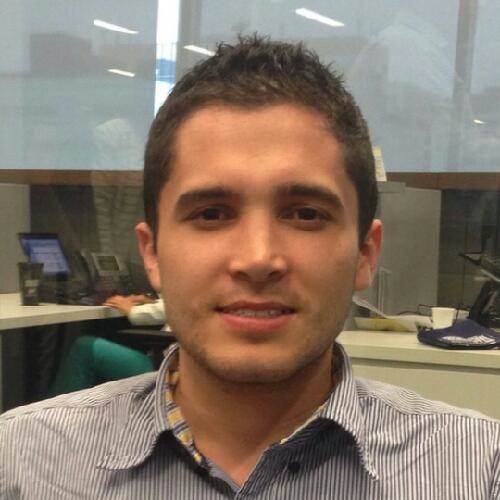
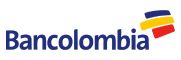