Adding Search Functionality to NEXT.js Apps: Integrating with Algolia
In today’s digital landscape, a fast and efficient search functionality is paramount for user engagement. Whether you’re building an e-commerce platform, a blog, or any web application, having a robust search feature can greatly enhance the user experience. In this tutorial, we will explore how to integrate Algolia, a powerful search and discovery API, into your NEXT.js apps to provide lightning-fast, typo-tolerant search capabilities.
1. Why Algolia?
Algolia is a cloud-based search platform that offers developers a suite of tools to build lightning-fast search experiences. It’s trusted by thousands of companies, including Twitch, Slack, and Stripe, for its speed, reliability, and scalability. Here are some reasons why you should consider integrating Algolia into your NEXT.js app:
1.1. Speed and Performance
Algolia is built for speed. It provides sub-millisecond search results, making it ideal for applications that require real-time search capabilities. Users expect instant responses, and Algolia delivers just that.
1.2. Typo-Tolerance
Users don’t always type perfectly, but Algolia understands their intent. It handles typos, synonyms, and partial queries gracefully, ensuring that users find what they’re looking for, even if they make mistakes in their search queries.
1.3. Scalability
Whether you have a small blog or a massive e-commerce platform, Algolia scales effortlessly to meet your needs. It can handle millions of records without compromising on speed or performance.
1.4. Easy Integration
Algolia provides robust JavaScript libraries and APIs that make integration with popular frameworks like NEXT.js a breeze. You can get your search functionality up and running quickly.
Now that we understand why Algolia is a great choice let’s dive into the steps to integrate it into your NEXT.js app.
2. Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- A NEXT.js app set up and running.
- Node.js installed on your development machine.
- A free or paid Algolia account. You can sign up at Algolia’s website.
3. Setting Up Algolia
The first step in integrating Algolia into your NEXT.js app is to set up an Algolia account and configure an index. Follow these steps:
3.1. Sign Up for an Algolia Account
If you don’t already have an Algolia account, head over to Algolia’s website and sign up. Once you’re signed in, you’ll be able to create and manage your indices.
3.2. Create an Index
In Algolia, an index is a collection of records that you want to make searchable. For example, if you’re building an e-commerce site, you might have an index for products. To create an index:
- Log in to your Algolia dashboard.
- Click on “Indices” in the left sidebar.
- Click the “New Index” button.
- Give your index a name and configure its settings according to your data structure.
3.3. Obtain API Credentials
To interact with your Algolia index, you’ll need API credentials. You can find these credentials in your Algolia dashboard. Make sure to keep them safe, as they’ll be used to authenticate requests to the Algolia API.
4. Installing the Algolia JavaScript Client
To start using Algolia in your NEXT.js app, you need to install the Algolia JavaScript client library. This library provides an easy-to-use interface for interacting with your Algolia index.
Open your project directory in your terminal and run the following command to install the Algolia JavaScript client:
bash npm install algoliasearch
5. Initializing the Algolia Client
With the Algolia JavaScript client library installed, you can now initialize it in your NEXT.js app. Create a new file named algolia.js in your project directory and add the following code:
javascript import algoliasearch from 'algoliasearch/lite'; const algoliaClient = algoliasearch( process.env.ALGOLIA_APP_ID, process.env.ALGOLIA_API_KEY ); export const algoliaIndex = algoliaClient.initIndex('your_index_name');
In this code, we import the algoliasearch module and initialize it with your Algolia Application ID and API Key. Replace ‘your_index_name’ with the name of the index you created earlier.
Don’t forget to add your Algolia credentials to your project’s environment variables, as we’re using process.env to access them securely.
6. Creating a Search Component
Now that we’ve set up Algolia and initialized the client, it’s time to create a search component in your NEXT.js app. This component will allow users to input their search queries and display the search results.
6.1. Create a Search.js Component
In your project directory, create a new file named Search.js inside a components folder. Add the following code to create a basic search component:
jsx import React, { useState } from 'react'; import { algoliaIndex } from '../algolia'; const Search = () => { const [query, setQuery] = useState(''); const [results, setResults] = useState([]); const search = async () => { if (query.trim() === '') { setResults([]); return; } try { const { hits } = await algoliaIndex.search(query); setResults(hits); } catch (error) { console.error('Error searching Algolia:', error); } }; return ( <div> <input type="text" placeholder="Search..." value={query} onChange={(e) => setQuery(e.target.value)} /> <button onClick={search}>Search</button> <ul> {results.map((result) => ( <li key={result.objectID}>{result.name}</li> ))} </ul> </div> ); }; export default Search;
In this code, we create a functional component called Search that uses React state to manage the search query and results. The search function sends a query to Algolia and updates the results state.
6.2. Include the Search Component
Now, you can include the Search component in your NEXT.js pages where you want to provide search functionality. For example, you can include it in your index.js page like this:
jsx import React from 'react'; import Search from '../components/Search'; const Home = () => { return ( <div> <h1>Welcome to My NEXT.js App</h1> <Search /> {/* Rest of your content */} </div> ); }; export default Home;
This will add the search component to your homepage, allowing users to search for content within your app.
7. Styling the Search Component
The Search component we created is functional, but it might not look visually appealing in its current state. You can style it to match your app’s design using CSS or a CSS-in-JS solution like styled-components. Here’s an example of how to style it using styled-components:
7.1. Install styled-components
If you haven’t already, install styled-components by running the following command:
bash npm install styled-components
7.2. Style the Search Component
Modify the Search.js file to include styled-components and style the component:
jsx import React, { useState } from 'react'; import { algoliaIndex } from '../algolia'; import styled from 'styled-components'; const SearchContainer = styled.div` display: flex; flex-direction: column; max-width: 400px; margin: 0 auto; padding: 16px; border: 1px solid #ccc; border-radius: 4px; `; const SearchInput = styled.input` padding: 8px; margin-bottom: 8px; `; const SearchButton = styled.button` padding: 8px 16px; background-color: #007bff; color: #fff; border: none; cursor: pointer; `; const ResultsList = styled.ul` list-style: none; padding: 0; `; const ResultItem = styled.li` margin-bottom: 4px; `; const Search = () => { const [query, setQuery] = useState(''); const [results, setResults] = useState([]); const search = async () => { if (query.trim() === '') { setResults([]); return; } try { const { hits } = await algoliaIndex.search(query); setResults(hits); } catch (error) { console.error('Error searching Algolia:', error); } }; return ( <SearchContainer> <SearchInput type="text" placeholder="Search..." value={query} onChange={(e) => setQuery(e.target.value)} /> <SearchButton onClick={search}>Search</SearchButton> <ResultsList> {results.map((result) => ( <ResultItem key={result.objectID}>{result.name}</ResultItem> ))} </ResultsList> </SearchContainer> ); }; export default Search;
In this code, we’ve created styled-components for various elements of the Search component, making it easier to apply custom styles. Adjust the styles to match your app’s design.
8. Deploying Your NEXT.js App with Algolia
With the search functionality integrated into your NEXT.js app, you’re ready to deploy it. Ensure that you’ve added your Algolia API credentials as environment variables in your deployment environment. When you deploy your app, it should work seamlessly with Algolia’s search functionality.
Conclusion
In this tutorial, we’ve covered the process of integrating Algolia into your NEXT.js app to supercharge your search functionality. Algolia’s speed, typo-tolerance, and scalability make it an excellent choice for providing an enhanced search experience to your users.
Table of Contents
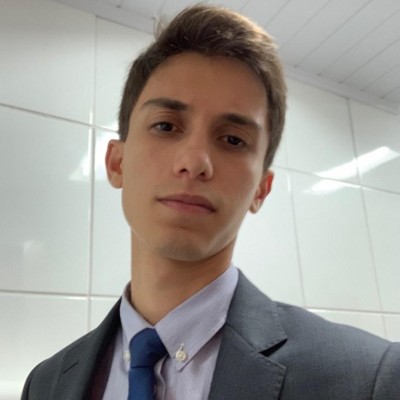
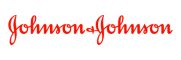