Exploring Authentication Options in NEXT.js: OAuth, JWT, and Cookies
Authentication is a fundamental aspect of web development, ensuring that users have secure access to their accounts and data. In the world of Next.js, a popular React framework for building web applications, several authentication options are available. In this blog post, we’ll explore three common methods: OAuth, JSON Web Tokens (JWT), and cookies. We’ll discuss their strengths, weaknesses, and how to implement them effectively in your Next.js projects.
1. Understanding Authentication
Before diving into the specifics of OAuth, JWT, and cookies, let’s take a moment to understand the concept of authentication. Authentication is the process of verifying the identity of a user or system. In web development, this typically involves confirming that a user is who they claim to be before granting access to certain resources or features.
In the context of Next.js applications, authentication plays a crucial role in ensuring that only authorized users can access protected routes, perform actions, or view sensitive data. Different authentication methods offer various levels of security, ease of implementation, and user experience.
2. OAuth: A Standard for External Authentication
2.1. What is OAuth?
OAuth, which stands for “Open Authorization,” is an industry-standard protocol used for delegated authorization. It allows a user to grant limited access to their resources on one website (known as the “resource server”) to another website or application (known as the “client”) without exposing their credentials, such as their username and password.
2.2. Strengths of OAuth
- Third-party Authentication: OAuth excels at enabling third-party authentication. It allows users to log in using their existing accounts on platforms like Google, Facebook, or GitHub. This simplifies the registration process and enhances user convenience.
- Secure Access Control: OAuth allows fine-grained control over what data or actions the client can access on behalf of the user. This makes it suitable for applications that require specific permissions, such as accessing a user’s social media posts or email contacts.
- Single Sign-On (SSO): OAuth supports Single Sign-On, enabling users to log in once and access multiple services without needing to enter credentials repeatedly.
2.3. Weaknesses of OAuth
- Complex Implementation: Implementing OAuth can be complex, especially for beginners. It involves multiple steps, including obtaining OAuth tokens and handling refresh tokens for prolonged sessions.
- User Experience: While OAuth offers convenience, some users may be concerned about sharing their data with third-party applications. Ensuring transparent data usage practices is crucial to maintain user trust.
2.4. Implementing OAuth in NEXT.js
To implement OAuth in a Next.js application, you’ll typically use a library like next-auth or passport.js along with OAuth provider-specific strategies. Let’s look at a simplified example using next-auth for GitHub authentication:
javascript // Install next-auth and required dependencies npm install next-auth axios // Create a pages/api/auth/[...nextauth].js file import NextAuth from "next-auth"; import Providers from "next-auth/providers"; export default NextAuth({ providers: [ Providers.GitHub({ clientId: process.env.GITHUB_CLIENT_ID, clientSecret: process.env.GITHUB_CLIENT_SECRET, }), // Add more providers as needed ], // Additional configuration options });
Remember to set up OAuth credentials for the respective providers and configure your environment variables securely.
3. JSON Web Tokens (JWT): A Stateless Authentication Solution
3.1. What are JWTs?
JSON Web Tokens, or JWTs, are a compact and self-contained means of securely transmitting information between parties. They are often used for authentication and authorization purposes. A JWT consists of three parts: a header, a payload, and a signature.
3.2. Strengths of JWT
- Stateless: JWTs are stateless, meaning that the server doesn’t need to store session information. This simplifies server-side scaling and reduces the load on the backend.
- Decentralized Authentication: JWTs are particularly useful in microservices architectures, as they can carry authentication information that’s verified by each service independently.
- Custom Data: The payload of a JWT can contain custom data, allowing you to include user-specific information or permissions.
3.3. Weaknesses of JWT
- Security Implications: If not implemented correctly, JWTs can introduce security risks. It’s crucial to validate and sanitize the data in a JWT to prevent attacks like token substitution.
- Token Expiry: JWTs can have a fixed expiration time, but refreshing them can be challenging. You may need to implement a separate mechanism for token renewal.
3.4. Implementing JWT in NEXT.js
To implement JWT-based authentication in a Next.js application, you’ll need to create an authentication flow for issuing and verifying tokens. Here’s a simplified example using the jsonwebtoken library:
javascript // Install the jsonwebtoken library npm install jsonwebtoken // Example of issuing a JWT token const jwt = require("jsonwebtoken"); const secretKey = "your-secret-key"; const user = { id: 1, username: "example_user" }; const token = jwt.sign(user, secretKey, { expiresIn: "1h" }); // Example of verifying a JWT token const decodedToken = jwt.verify(token, secretKey);
In a real Next.js application, you would integrate JWT-based authentication into your routes and user management system.
4. Cookies: Session-Based Authentication
4.1. What are Cookies?
Cookies are small pieces of data stored on the client’s browser. They are commonly used for session management and authentication purposes. In a typical authentication scenario, a session ID or token is stored as a cookie on the client side, and the server uses it to identify the user.
4.2. Strengths of Cookies
- Simplicity: Cookies are easy to implement and widely supported by web browsers. They are a straightforward way to manage user sessions.
- Automatic Expiry: You can set cookies to expire automatically after a specified period, enhancing security.
4.3. Weaknesses of Cookies
- Stateful: Cookies introduce statefulness to your application since session data is stored on the client side. This can complicate server-side scaling and maintenance.
- CSRF Vulnerability: Cookies are vulnerable to Cross-Site Request Forgery (CSRF) attacks if not properly secured with anti-CSRF tokens.
4.4. Implementing Cookies in NEXT.js
To implement cookie-based authentication in a Next.js application, you can use libraries like cookie and cookie-parser. Here’s a simplified example:
javascript // Install the cookie and cookie-parser libraries npm install cookie cookie-parser // Example of setting a cookie const cookie = require("cookie"); const cookieParser = require("cookie-parser"); const secretKey = "your-secret-key"; const user = { id: 1, username: "example_user" }; const token = jwt.sign(user, secretKey, { expiresIn: "1h" }); const encodedToken = cookie.serialize("authToken", token, { httpOnly: true, maxAge: 3600, // 1 hour sameSite: "strict", secure: process.env.NODE_ENV === "production", }); // Example of parsing a cookie const cookies = cookie.parse(req.headers.cookie || ""); const authToken = cookies.authToken;
This example demonstrates how to set and parse cookies for authentication purposes. Remember to secure your cookies and handle session expiration.
5. Choosing the Right Authentication Method
Choosing the right authentication method for your Next.js application depends on your specific requirements and constraints. Here’s a quick summary of when to consider each method:
- OAuth: Use OAuth when you want to enable third-party authentication or require fine-grained control over user data access. It’s suitable for applications with social login options or integrations with external services.
- JWT: Consider JWT when you need stateless authentication, custom data in tokens, or a decentralized authentication mechanism for microservices. JWTs are a good fit for modern, scalable web applications.
- Cookies: Cookies are a solid choice for simple session-based authentication. Use them when you want a straightforward implementation with automatic session management.
Remember that you can also combine these methods in your Next.js application to achieve the desired level of security and user experience.
Conclusion
Authentication is a critical aspect of web development, and Next.js offers flexibility when it comes to choosing the right method for your application. OAuth, JWT, and cookies each have their strengths and weaknesses, making them suitable for different use cases.
When implementing authentication in Next.js, consider factors like user experience, security, and the complexity of implementation. Always prioritize security by following best practices for the chosen authentication method, and keep your users’ data safe and accessible.
In summary, explore the world of authentication options in Next.js, experiment with different methods, and choose the one that best aligns with your project’s requirements and goals. Whether you opt for OAuth, JWT, cookies, or a combination of these, Next.js provides a robust platform for building secure and user-friendly web applications.
Happy coding!
Table of Contents
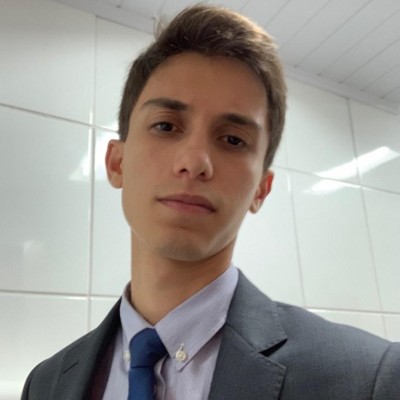
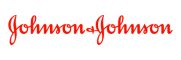