Exploring Authentication Providers in NEXT.js: Google, Facebook, and GitHub
Authentication is a fundamental aspect of modern web applications, ensuring that only authorized users can access certain features or data. In this blog post, we will explore how to integrate authentication providers into your NEXT.js application using popular services like Google, Facebook, and GitHub. We’ll provide step-by-step guidance and code samples to help you implement these authentication methods effectively.
1. Why Use Authentication Providers?
Authentication providers offer a convenient way to enable users to log in or sign up to your web application without the need to create and remember another set of login credentials. They allow users to use their existing accounts from well-known platforms, such as Google, Facebook, or GitHub, to access your application, simplifying the registration process and enhancing user experience.
Here are some key benefits of using authentication providers in your NEXT.js app:
1.1. Improved User Experience
Authentication providers offer a seamless and familiar login experience for users who are already registered with these platforms. This reduces friction during the sign-up process and can lead to higher user retention.
1.2. Enhanced Security
By relying on trusted authentication providers, you can benefit from their robust security mechanisms, reducing the risk of security breaches and unauthorized access.
1.3. Social Integration
Integrating with popular platforms like Google, Facebook, and GitHub allows your application to tap into users’ social networks, enabling social features such as sharing and social interactions within your app.
Now that we understand the advantages of using authentication providers, let’s dive into the implementation details for each of these platforms.
2. Setting Up Google Authentication
Google is one of the most widely used authentication providers, and integrating it into your NEXT.js app is relatively straightforward. Follow these steps to get started:
2.1. Create a Google Developer Project
To begin, you’ll need to create a project in the Google Developer Console. Follow the on-screen instructions to set up your project and obtain the necessary credentials.
2.2. Configure OAuth 2.0
Within your Google Developer Project, configure OAuth 2.0 by adding your application’s details. You’ll receive a Client ID and a Client Secret, which you’ll use in your NEXT.js app to enable Google authentication.
2.3. Install Dependencies
In your NEXT.js project, install the required dependencies for Google authentication using npm or yarn:
bash npm install react-google-login
2.4. Implement Google Login Component
Create a component for Google login using the react-google-login package. Here’s an example:
jsx import React from 'react'; import { GoogleLogin } from 'react-google-login'; const GoogleAuth = () => { const responseGoogle = (response) => { console.log(response); // Handle the response, e.g., authenticate the user on your server }; return ( <GoogleLogin clientId="YOUR_CLIENT_ID" buttonText="Login with Google" onSuccess={responseGoogle} onFailure={responseGoogle} cookiePolicy={'single_host_origin'} /> ); }; export default GoogleAuth;
Replace ‘YOUR_CLIENT_ID’ with the actual Client ID obtained from your Google Developer Project.
2.5. Authenticate on the Server
When a user successfully logs in with Google, you’ll receive a response containing the user’s information, including their Google ID and email. You can use this information to authenticate the user on your server and create a session.
This is a basic overview of implementing Google authentication in your NEXT.js app. You can customize the login flow and user experience based on your application’s requirements.
3. Integrating Facebook Authentication
Facebook authentication is another popular choice for allowing users to sign in to your application. Follow these steps to integrate Facebook authentication into your NEXT.js app:
3.1. Create a Facebook App
Start by creating a new Facebook App on the Facebook Developer Portal. This will provide you with the necessary credentials for authentication.
3.2. Configure OAuth Settings
In your Facebook App settings, configure the OAuth settings by adding your NEXT.js app’s URL and defining the redirect URL for successful logins.
3.3. Install Dependencies
In your NEXT.js project, install the required dependencies for Facebook authentication using npm or yarn:
bash npm install react-facebook-login
3.4. Implement Facebook Login Component
Create a component for Facebook login using the react-facebook-login package. Here’s an example:
jsx import React from 'react'; import FacebookLogin from 'react-facebook-login'; const FacebookAuth = () => { const responseFacebook = (response) => { console.log(response); // Handle the response, e.g., authenticate the user on your server }; return ( <FacebookLogin appId="YOUR_APP_ID" autoLoad={false} fields="name,email,picture" callback={responseFacebook} /> ); }; export default FacebookAuth;
Replace ‘YOUR_APP_ID’ with the actual App ID obtained from your Facebook Developer App.
3.5. Authenticate on the Server
Similar to Google authentication, when a user successfully logs in with Facebook, you’ll receive a response containing the user’s information. You can use this information to authenticate the user on your server.
4. Implementing GitHub Authentication
GitHub authentication is an excellent choice for applications targeting developers and technical users. Follow these steps to integrate GitHub authentication into your NEXT.js app:
4.1. Register a GitHub OAuth Application
Visit the GitHub Developer Settings page and create a new OAuth application. This will provide you with a Client ID and Client Secret for authentication.
4.2. Install Dependencies
In your NEXT.js project, install the required dependencies for GitHub authentication using npm or yarn:
bash npm install react-github-login
4.3. Implement GitHub Login Component
Create a component for GitHub login using the react-github-login package. Here’s an example:
jsx import React from 'react'; import GitHubLogin from 'react-github-login'; const GitHubAuth = () => { const onSuccess = (response) => { console.log(response); // Handle the response, e.g., authenticate the user on your server }; const onFailure = (response) => { console.error(response); }; return ( <GitHubLogin clientId="YOUR_CLIENT_ID" onSuccess={onSuccess} onFailure={onFailure} /> ); }; export default GitHubAuth;
Replace ‘YOUR_CLIENT_ID’ with the actual Client ID obtained from your GitHub OAuth application.
4.4. Authenticate on the Server
As with other authentication providers, when a user logs in with GitHub, you’ll receive a response containing their GitHub ID and other information. You can use this information to authenticate the user on your server.
Conclusion
In this blog post, we’ve explored how to implement authentication providers in your NEXT.js application using popular platforms like Google, Facebook, and GitHub. These authentication methods provide a convenient and secure way for users to log in to your app, enhancing the overall user experience.
Remember that authentication is a critical aspect of any web application, and you should take the necessary precautions to ensure the security of your users’ data. Always store user information securely, use encryption where necessary, and keep your authentication libraries up to date.
By following the steps and code samples provided in this guide, you can quickly integrate these authentication providers into your NEXT.js app and offer a seamless login experience for your users.
Start implementing authentication providers in your NEXT.js app today and take your user experience to the next level!
Table of Contents
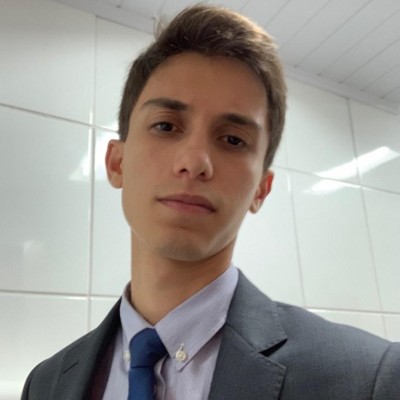
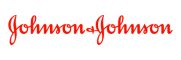