Empower Your Tech Team: Harnessing GraphQL with NEXT.js & AWS AppSync
In the fast-paced world of technology, having the right tools and frameworks can make a significant difference in the development process. One powerful combination that has gained popularity among early-stage startup founders, VC investors, and tech leaders is NEXT.js and AWS AppSync. In this blog post, we’ll dive deep into how these two tools can be harnessed together to create efficient and scalable GraphQL APIs.
Table of Contents
GraphQL has revolutionized the way we interact with APIs, offering a flexible and efficient way to fetch data. NEXT.js, on the other hand, is a popular React framework known for its server-side rendering capabilities. By integrating NEXT.js with AWS AppSync, you can create robust GraphQL APIs that not only perform well but also scale effortlessly to meet the demands of your application.
1. Getting Started
Before we jump into the details, let’s set up our development environment and explore the basic concepts of NEXT.js and AWS AppSync. You can find detailed installation instructions and setup guides for NEXT.js and AWS AppSync in their respective documentation.
1.1 Creating a NEXT.js Project
To get started, let’s create a new NEXT.js project. You can use the following command to initialize a new project:
```bash npx create-next-app my-graphql-app ```
Once your project is set up, navigate to the project directory and install the necessary dependencies:
```bash cd my-graphql-app npm install aws-amplify @aws-amplify/ui-react ```
1.2 Setting Up AWS AppSync
Now, let’s set up AWS AppSync to handle our GraphQL API. Follow these steps:
- Log in to your AWS account or create one if you don’t have an account already.
- Go to the AWS AppSync console and create a new API.
- Define your data model using the schema definition language (SDL). Here’s a simple example:
```graphql type Post { id: ID! title: String! content: String! } type Query { getPosts: [Post] } ```
- Create a data source (e.g., Amazon DynamoDB) and connect it to your GraphQL API.
- Set up resolvers to handle the queries and mutations defined in your schema.
- Deploy your API.
1.3 Integrating AWS AppSync with NEXT.js
With your GraphQL API up and running, it’s time to integrate it with your NEXT.js application. You can achieve this using the AWS Amplify library. Here’s how to do it:
Import and configure AWS Amplify in your NEXT.js project:
```javascript import Amplify from 'aws-amplify'; import config from './aws-exports'; Amplify.configure(config); ```
Use the `withAuthenticator` HOC (Higher Order Component) from `@aws-amplify/ui-react` to secure your application with authentication:
```javascript import { withAuthenticator } from '@aws-amplify/ui-react'; const MyApp = () => { // Your application code }; export default withAuthenticator(MyApp); ```
Now, you can make GraphQL queries to your AWS AppSync API using the `Amplify` library. Here’s an example of fetching posts:
```javascript import { API, graphqlOperation } from 'aws-amplify'; import { getPosts } from './graphql/queries'; const fetchPosts = async () => { try { const response = await API.graphql(graphqlOperation(getPosts)); const posts = response.data.getPosts; // Handle the fetched data } catch (error) { // Handle errors } }; ```
Conclusion
In this blog post, we’ve explored how to use NEXT.js with AWS AppSync to build GraphQL APIs. This powerful combination allows you to create efficient and scalable APIs that are essential for the success of your early-stage startup or tech venture.
Now that you have a solid foundation, it’s time to dive even deeper into the capabilities of these tools and leverage them to outperform the competition in the tech industry.
External Resource:
To further enhance your understanding, here are three external links that provide additional insights into using NEXT.js and AWS AppSync:
- NEXT.js Documentation – https://nextjs.org/docs/getting-started/introduction
- AWS AppSync Documentation – https://docs.aws.amazon.com/appsync/latest/devguide/introduction.html
- GraphQL Official Website – https://graphql.org/
With the right tools and knowledge, you’re well on your way to building cutting-edge applications and APIs that will impress your target audience of early-stage startup founders, VC investors, and tech leaders.
Table of Contents
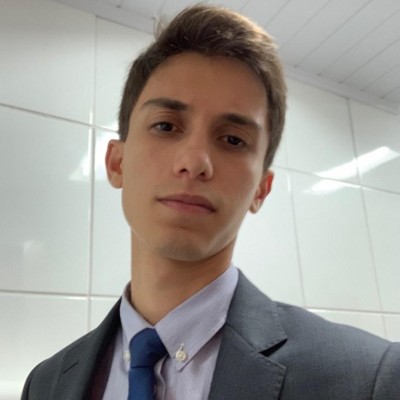
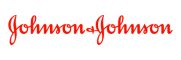