NEXT.js & Eventbrite API Mastery: Your Path to Event Booking Excellence
In the fast-paced world of tech startups, the ability to roll out interactive, user-friendly applications quickly is a game-changer. For those in the event management sector or any entrepreneur looking to bridge the gap between event organizers and attendees, developing an event booking app is a strategic move. Leveraging the power of NEXT.js alongside the Eventbrite API can make this not just a possibility but a reality. Let’s dive into the nitty-gritty of building an event booking app that could potentially outperform the competition.
Table of Contents
1. Why NEXT.js and Eventbrite API?
NEXT.js stands as a React framework that offers server-side rendering, which helps in boosting the app’s performance and improving its SEO friendliness. Its automatic routing, optimized prefetching, and ease of deployment make NEXT.js a go-to choice for developers aiming for production-grade applications.
Eventbrite API, on the other hand, is your golden ticket to accessing a wide range of event-related data. From creating events to fetching details about event attendees, the Eventbrite API provides a robust backend to manage events effectively.
2. Getting Started
Step 1: Setting Up Your NEXT.js Environment
Kick things off by creating a new NEXT.js app. If you haven’t already, you’ll need to install Node.js on your machine. Once done, open your terminal and run the following command:
```bash npx create-next-app your-event-app ```
Navigate into your project directory:
```bash cd your-event-app ```
And boom! You’re in the heart of your very own NEXT.js project. For more on NEXT.js and its capabilities, dive into the [official NEXT.js documentation](https://nextjs.org/docs).
Step 2: Integrating Eventbrite API
Before you can start pulling in data from Eventbrite, you’ll need to get your hands on an API key. Head over to the [Eventbrite Developer Page](https://www.eventbrite.com/platform), sign up or log in, and create a new application to obtain your API key.
With your API key in tow, it’s time to integrate it into your app. Create a `.env.local` file at the root of your NEXT.js project and add your Eventbrite API key like so:
```plaintext EVENTBRITE_API_KEY=your_api_key_here ```
This will keep your API key secure and accessible throughout your app.
Step 3: Fetching Events from Eventbrite
To display events, you’ll need to interact with the Eventbrite API. NEXT.js makes fetching data a breeze with its built-in `getServerSideProps` function. Here’s a quick example of how you might fetch events:
```javascript // pages/index.js export async function getServerSideProps(context) { const res = await fetch(`https://www.eventbriteapi.com/v3/events/search/?token=${process.env.EVENTBRITE_API_KEY}`); const data = await res.json(); return { props: { events: data.events }, // will be passed to the page component as props } } ```
Step 4: Displaying the Events
With the data fetched, displaying it is just a matter of iterating over the events array and rendering the details. NEXT.js’s dynamic routing can be used to create dedicated pages for each event, enhancing the user experience.
```javascript // pages/index.js export default function Home({ events }) { return ( <div> <h1>Upcoming Events</h1> {events.map((event) => ( <div key={event.id}> <h2>{event.name.text}</h2> <p>{event.description.text}</p> {/* More event details */} </div> ))} </div> ) } ```
4. Taking It Further
4.1 Adding Authentication
For a more personalized experience, consider adding authentication. NEXT.js supports various authentication strategies that can be integrated with little hassle. [NextAuth.js](https://next-auth.js.org/) is a fantastic library for this, offering easy setup for multiple providers.
4.2 Event Management
Give your users the ability to create, update, and delete their events through your app. This requires a deeper integration with the Eventbrite API, handling form inputs, and ensuring secure API calls.
4.3 Payment Integration
If your app involves paid events, integrating a payment gateway like Stripe or PayPal is crucial. NEXT.js’s API routes can help manage backend logic for payment processing, providing a seamless checkout experience.
Conclusion
Building an event booking app with NEXT.js and the Eventbrite API is a potent combination for any tech entrepreneur looking to make a splash in the event management industry. This setup offers a balance of performance, SEO, and user engagement that can set your application apart from the competition.
For further reading and resources, check out:
Table of Contents
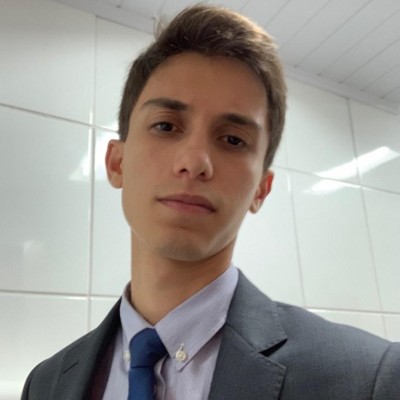
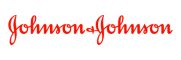