Building APIs with NEXT.js: REST vs. GraphQL
When developing modern web applications with NEXT.js, one crucial decision you’ll encounter is how to design and implement your API. Two popular options are REST and GraphQL. Each approach has its strengths and weaknesses, and understanding the differences between them is vital for making an informed choice.
As web applications have evolved, so have the approaches to building APIs. REST (Representational State Transfer) has been the dominant choice for many years. However, in recent times, GraphQL has gained significant traction due to its unique features and advantages.
In this blog, we’ll walk you through both REST and GraphQL, highlighting their characteristics, strengths, and weaknesses. By the end, you’ll have a clearer understanding of which API approach best suits your NEXT.js project.
1. REST: Representational State Transfer
1.1. Understanding REST
REST is an architectural style that defines a set of constraints to create scalable web services. It revolves around the concept of resources, which are identified by URLs. RESTful APIs use HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on these resources.
1.1.1. REST Characteristics:
- Statelessness: Each request from a client to a server must contain all the information required to understand and process the request. The server doesn’t store any client state between requests.
- Uniform Interface: REST APIs follow a consistent and standardized set of constraints, including using unique URLs for resources, employing standard HTTP methods, and returning well-defined response formats (e.g., JSON or XML).
- Client-Server Architecture: The client and server are separate entities that communicate over HTTP. This separation allows for more flexibility and scalability.
- Layered System: RESTful APIs can be composed of multiple layers, with each layer having specific functionality. This promotes a clear separation of concerns and modularity.
- Cacheability: Responses from a REST API can be cacheable, improving performance and reducing server load.
1.2. REST Pros and Cons
1.2.1. Pros:
- Ease of Use: REST APIs are straightforward to understand and implement, making them an excellent choice for beginners.
- Caching Support: By leveraging HTTP cache mechanisms, REST APIs can enhance performance and reduce the number of requests to the server.
- Statelessness: The stateless nature of REST simplifies the server-side logic and allows for better scalability.
1.2.2. Cons:
- Overfetching/Underfetching: REST APIs may suffer from overfetching or underfetching of data. Clients might receive more data than needed or have to make multiple requests to fetch all required data.
- Versioning: Making changes to a REST API without breaking existing clients can be challenging. Versioning may become necessary as the API evolves.
1.3. Implementing a REST API with NEXT.js
In a NEXT.js application, building a REST API is relatively straightforward. You can start by defining your API routes using the built-in API routes feature. Let’s create a simple example of a RESTful API to manage a collection of books.
1. First, create a new file called books.js in the pages/api directory.
2. In the books.js file, define your REST API routes using the following code:
jsx // pages/api/books.js const books = [ { id: 1, title: 'Book 1', author: 'Author 1' }, { id: 2, title: 'Book 2', author: 'Author 2' }, { id: 3, title: 'Book 3', author: 'Author 3' }, ]; export default function handler(req, res) { if (req.method === 'GET') { res.status(200).json(books); } else if (req.method === 'POST') { const { id, title, author } = req.body; const newBook = { id, title, author }; books.push(newBook); res.status(201).json(newBook); } }
In this example, we have two endpoints: a GET endpoint to fetch all books and a POST endpoint to add a new book to the collection. Note that this is a simplified demonstration, and in a real-world application, you would likely use a database to store and retrieve data.
3. Start your NEXT.js development server using npm run dev, and your REST API will be available at /api/books.
That’s it! You’ve now created a basic RESTful API using NEXT.js.
2. GraphQL: Query Language for APIs
2.1. Understanding GraphQL
GraphQL is a query language for APIs, enabling clients to request precisely the data they need and nothing more. It was developed by Facebook to address the limitations and inefficiencies of REST APIs. Instead of relying on multiple endpoints, a GraphQL API provides a single endpoint where clients can specify their data requirements.
2.1.1. GraphQL Characteristics:
- Declarative Data Fetching: Clients can specify the exact shape of the data they require, including nested fields and relationships. This eliminates overfetching and underfetching issues.
- Single Endpoint: Unlike REST APIs, which might require multiple endpoints for different resources, GraphQL APIs have a single endpoint that handles all data requests.
- Strongly Typed Schema: GraphQL APIs are backed by a strongly typed schema, providing clarity on available data and operations. Clients can explore the schema using introspection.
- Real-time Updates: GraphQL supports real-time data updates using subscriptions. Clients can subscribe to specific events and receive live updates when data changes.
2.2. GraphQL Pros and Cons
2.2.1. Pros:
- Precise Data Fetching: With GraphQL, clients can request only the data they need, reducing the response size and improving performance.
- Schema Introspection: The strongly typed schema enables powerful tools and IDE support, making it easier to explore and interact with the API.
- Rapid Iteration: Since clients can request new data fields without backend changes, development cycles can be faster.
2.2.2. Cons:
- Learning Curve: GraphQL introduces a different way of thinking about APIs, which might require some learning and adjustment.
- Caching Complexity: GraphQL’s flexibility can make caching more challenging to implement effectively.
2.3. Implementing a GraphQL API with NEXT.js
Setting up a GraphQL API in a NEXT.js application requires an additional step compared to REST APIs. We need to add a GraphQL server to handle incoming queries. Let’s create a basic GraphQL server for our book
1. First, make sure you have the necessary dependencies installed. You’ll need apollo-server-micro for the GraphQL server and graphql for defining the schema.
bash npm install apollo-server-micro graphql
2. Create a new file called graphql.js in the pages/api directory. This file will handle GraphQL queries and mutations.
3. Define your GraphQL schema in the graphql.js file. For our book collection example, the schema will look like this:
jsx // pages/api/graphql.js import { ApolloServer, gql } from 'apollo-server-micro'; const typeDefs = gql` type Book { id: Int title: String author: String } type Query { books: [Book] } type Mutation { addBook(id: Int, title: String, author: String): Book } `; const books = [ { id: 1, title: 'Book 1', author: 'Author 1' }, { id: 2, title: 'Book 2', author: 'Author 2' }, { id: 3, title: 'Book 3', author: 'Author 3' }, ]; const resolvers = { Query: { books: () => books, }, Mutation: { addBook: (_, { id, title, author }) => { const newBook = { id, title, author }; books.push(newBook); return newBook; }, }, }; const apolloServer = new ApolloServer({ typeDefs, resolvers, }); export const config = { api: { bodyParser: false, }, }; export default apolloServer.createHandler({ path: '/api/graphql' });
In this example, we have defined a GraphQL schema with two types: Book and Query. The Query type has a books field, allowing clients to fetch all books. Additionally, we have a Mutation type with an addBook field, which enables clients to add new books to the collection.
4. Now, start your NEXT.js development server using npm run dev. Your GraphQL API will be available at /api/graphql.
Congratulations! You’ve successfully implemented a basic GraphQL API with NEXT.js.
4. Comparing REST and GraphQL
Both REST and GraphQL have their merits, and the choice between them depends on your specific project requirements. Let’s compare them based on some crucial factors:
4.1. Performance
In terms of performance, REST APIs can have an advantage when it comes to simple queries that retrieve specific resources. Since REST follows a resource-oriented approach, fetching individual items can be more efficient.
On the other hand, GraphQL shines when dealing with complex data requirements. By allowing clients to request only the data they need, GraphQL reduces the response size and the number of requests to the server, resulting in a more efficient data retrieval process.
4.2. Flexibility
REST APIs can sometimes suffer from underfetching or overfetching issues. Clients might receive too much or too little data, which can impact performance. However, REST provides more fine-grained control over which resources to request and manipulate.
GraphQL, being more flexible, allows clients to request precisely the data they need in a single request. This flexibility empowers frontend developers, as they can request new fields and features without requiring changes to the backend API.
4.3. Complexity
REST APIs tend to have a more straightforward structure, making them easier to learn and use, especially for simple projects. The standard HTTP methods provide clear guidelines on how to interact with the API.
On the other hand, GraphQL introduces a steeper learning curve due to its unique querying language and schema definition. However, once developers are familiar with GraphQL, the benefits of its flexibility and declarative data fetching become apparent.
5. Choosing the Right Approach
When deciding between REST and GraphQL for your NEXT.js project, consider the following factors:
- Data Complexity: If your application deals with complex data structures and relationships, GraphQL might be a better fit to efficiently fetch the required data.
- Client-Side Control: If frontend developers require more control over the data they retrieve, update, or delete, GraphQL’s declarative nature is advantageous.
- Scalability: REST’s statelessness makes it easier to scale horizontally. However, GraphQL’s ability to request only needed data can lead to better performance in certain scenarios.
- Existing Expertise: Consider the expertise of your development team. If your team is more familiar with REST and has no immediate need for GraphQL’s features, sticking with REST might be reasonable.
- Ecosystem and Tooling: Both REST and GraphQL have extensive ecosystems and tooling support. Evaluate the available libraries and integrations that align with your project’s requirements.
Conclusion
In this blog post, we explored the differences between REST and GraphQL for building APIs with NEXT.js. We discussed the core concepts, benefits, and drawbacks of each approach, providing code samples for implementing both RESTful and GraphQL APIs.
REST offers simplicity and ease of use, making it suitable for straightforward projects and when precise control over resources is essential. On the other hand, GraphQL provides more flexibility and efficiency for complex data retrieval, empowering frontend developers with precise control over data requirements.
Ultimately, the choice between REST and GraphQL depends on the specific needs and goals of your NEXT.js application. By understanding the strengths and weaknesses of both approaches, you can make an informed decision and build a robust and efficient API that perfectly suits your project.
Now, armed with this knowledge, you can confidently embark on building APIs that will drive the success of your web applications. Happy coding!
Table of Contents
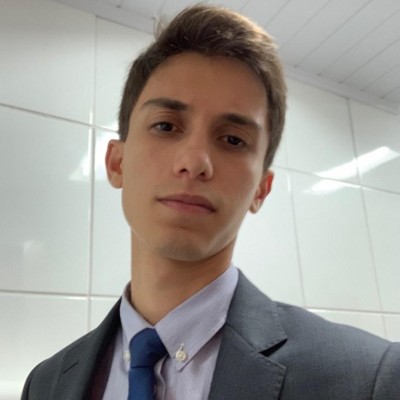
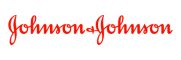