Creating Custom Hooks in NEXT.js: Reusability at Its Best
When developing applications with Next.js, a popular React framework, maximizing reusability and maintainability is essential. Custom hooks offer an elegant solution to achieve this by extracting component logic into reusable functions. In this blog post, we will dive into the world of custom hooks and learn how they can make our code more organized, scalable, and easy to maintain.
1. What are Custom Hooks?
Custom hooks are JavaScript functions that utilize React’s hooks like useState, useEffect, and more to encapsulate logic and state management that can be reused across multiple components. The convention for naming custom hooks is to prefix the function name with use, which signals to other developers that it is intended to be used as a hook.
The beauty of custom hooks lies in their simplicity and reusability. They allow developers to abstract away complex logic and state handling into easily accessible functions. This not only makes the code more concise and easier to understand but also facilitates collaborative development by providing a standardized interface for sharing logic across components.
2. Creating a Custom Hook
Creating a custom hook is as simple as creating a JavaScript function with the use prefix. Let’s start by building a basic custom hook to fetch data from an API using fetch.
Step 1: Initializing the Custom Hook
jsx // useFetch.js import { useState, useEffect } from 'react'; const useFetch = (url) => { const [data, setData] = useState(null); const [isLoading, setLoading] = useState(true); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await fetch(url); if (!response.ok) { throw new Error('Network response was not ok'); } const jsonData = await response.json(); setData(jsonData); } catch (error) { setError(error); } finally { setLoading(false); } }; fetchData(); }, [url]); return { data, isLoading, error }; }; export default useFetch;
Step 2: Using the Custom Hook
Now that we have our custom hook useFetch, we can utilize it in any of our components to fetch data from the API without duplicating the fetching logic.
jsx // MyComponent.js import React from 'react'; import useFetch from '../hooks/useFetch'; const MyComponent = () => { const apiUrl = 'https://api.example.com/data'; const { data, isLoading, error } = useFetch(apiUrl); if (isLoading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return ( <div> {/* Render your component using the fetched data */} </div> ); }; export default MyComponent;
By using the custom hook useFetch, we have separated the data fetching logic from the component itself. This makes the component cleaner and more focused on rendering the data, while the custom hook handles the fetching process.
3. Advantages of Using Custom Hooks
3.1. Code Reusability
Custom hooks promote code reusability by encapsulating logic that can be shared across different components. As demonstrated in the previous example, we can easily reuse the useFetch hook in multiple components without duplicating the fetching logic.
3.2. Separation of Concerns
By abstracting away complex logic into custom hooks, we achieve a clear separation of concerns. Components become simpler and more focused on their primary task, improving code readability and maintainability.
3.3. Improved Testability
Custom hooks are easier to test since they are independent functions with clear inputs and outputs. By writing unit tests for the custom hooks, we can ensure their correctness, which in turn enhances the reliability of our components.
3.4. Collaboration and Consistency
Custom hooks foster collaboration among developers, especially in larger teams, by providing a standardized way of handling common functionalities. This consistency across the codebase improves overall development speed and reduces bugs due to differing implementations.
4. Tips for Creating Custom Hooks
4.1. Follow the use Prefix Convention
To avoid confusion and clearly indicate the intent of the function, always prefix custom hook names with use. This aligns with the established naming convention for hooks in React.
4.2. Keep Hooks Small and Focused
Custom hooks should ideally focus on one specific concern or functionality. This ensures that the hooks remain reusable and easy to understand. If a hook becomes too large and complex, consider splitting it into smaller, more focused hooks.
4.3. Leverage Built-in Hooks
Custom hooks can also use other built-in React hooks, allowing you to compose functionality. For example, a custom hook can use useState or useEffect to manage component state or handle side effects.
4.4. Document Your Custom Hooks
Just like any other piece of code, it’s essential to document your custom hooks properly. Clearly explain what the hook does, its input parameters, return values, and any additional considerations for using it effectively.
Conclusion
In this blog post, we explored the power of custom hooks in Next.js and how they enable us to achieve reusability at its best. By encapsulating logic into custom hooks, we can make our code more modular, maintainable, and scalable.
Whether you’re building a small personal project or a large-scale application, custom hooks offer an elegant solution to improve code organization and collaboration among developers. Embrace the world of custom hooks, and unlock the true potential of your Next.js applications!
Table of Contents
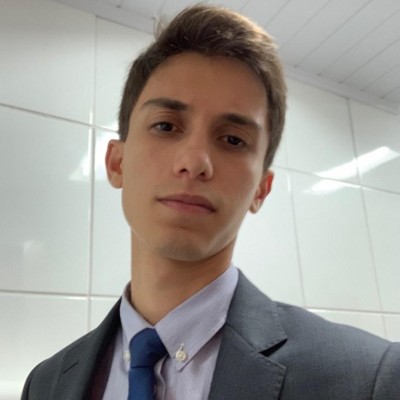
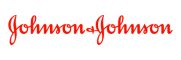