Unveiling the Power of Data Fetching in Next.js: A Deep Dive into SSR, SSG, and Client-Side Strategies
Next.js has become one of the most sought-after technologies for building server-side rendered (SSR) applications with React, leading many organizations to hire Next.js developers to leverage its capabilities. One of its standout features is the unique approach to data fetching. This feature has become a significant factor for businesses looking to hire Next.js developers, as it allows them to create efficient, high-performing applications. Next.js provides several data fetching strategies, each designed to fulfill different requirements, further emphasizing the value and versatility that Next.js developers can bring to a project.
In this blog post, we’ll be looking at three main data fetching strategies that Next.js provides:
- Server-Side Rendering (SSR)
- Static Site Generation (SSG)
- Client-Side Data Fetching
Server-Side Rendering (SSR)
Server-Side Rendering means that the page is rendered on the server at runtime. This approach is ideal for pages that need dynamic data that changes on every request. Next.js provides a function, `getServerSideProps()`, to fetch data at request time.
Example of SSR
Let’s look at an example of fetching a list of posts from an API using SSR.
```jsx import axios from 'axios'; export async function getServerSideProps(context) { const res = await axios.get('https://api.example.com/posts'); const data = res.data; if (!data) { return { notFound: true, }; } return { props: { data }, // will be passed to the page component as props }; } const Posts = ({ data }) => { return ( <ul> {data.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }; export default Posts; ```
In this example, we are fetching the posts during the server-side rendering phase using `getServerSideProps()`. The fetched data is passed to the page component as props.
Static Site Generation (SSG)
Static Site Generation is another strategy provided by Next.js. Here, the HTML pages are generated at build time and reused on each request. This approach is ideal for pages that can be pre-rendered ahead of a user’s request, especially when the data changes infrequently.
Next.js provides a function, `getStaticProps()`, to fetch data at build time.
Example of SSG
Let’s see an example where we pre-render a blog post using SSG.
```jsx import axios from 'axios'; export async function getStaticProps(context) { const res = await axios.get(`https://api.example.com/posts/${context.params.id}`); const post = res.data; if (!post) { return { notFound: true, }; } return { props: { post }, }; } export async function getStaticPaths() { const res = await axios.get('https://api.example.com/posts'); const posts = res.data; const paths = posts.map((post) => ({ params: { id: post.id.toString() }, })); return { paths, fallback: false }; } const Post = ({ post }) => { return ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); }; export default Post; ```
In this example, we pre-render all posts at build time using `getStaticProps()` and `getStaticPaths()`. The fetched post is passed to the page component as props.
Client-Side Data Fetching
In some cases, it’s better to fetch data on the client side rather than pre-rendering it server-side. This approach is useful when parts of a page need to update frequently, or if the data is user-specific.
Next.js does not provide a specific function for client-side data fetching, but you can use any data fetching library, like `axios` or `fetch`.
Example of Client-Side Data Fetching
Let’s see how to fetch a user profile on the client side.
```jsx import axios from 'axios'; import { useState, useEffect } from 'react'; const Profile = () => { const [profile, setProfile] = useState(null); useEffect(() => { const fetchData = async () => { const res = await axios.get('/api/profile'); setProfile(res.data); }; fetchData(); }, []); if (!profile) { return <div>Loading...</div>; } return ( <div> <h1>{profile.name}</h1> <p>{profile.description}</p> </div> ); }; export default Profile; ```
In this example, we use the `useEffect` React hook to fetch the user profile data when the component is mounted. The fetched profile data is stored in the state and re-renders the component whenever the state changes.
Conclusion
Choosing the right data fetching strategy with Next.js depends on your specific use case, and sometimes it might be beneficial to hire Next.js developers to make the most out of this powerful framework. Server-Side Rendering (SSR) is ideal when you need the most up-to-date data, while Static Site Generation (SSG) can significantly boost your performance by pre-rendering pages at build time.
Client-side fetching is the way to go when dealing with user-specific data or when parts of your page need to update independently. In many applications, you’ll likely use a combination of these strategies. Having expert Next.js developers in your team can ensure that these strategies are implemented correctly, optimizing your application’s performance.
Fortunately, Next.js is flexible enough to support all these scenarios, making it a robust solution for your React-based web applications. It is this versatility and robustness that has led to a growing demand to hire Next.js developers in the industry.
Table of Contents
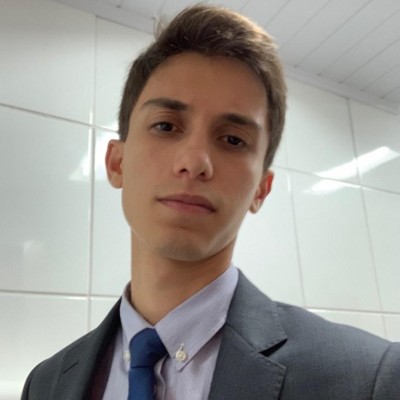
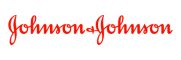