User-Centric NEXT.js: Empower Your App with React DnD Drag-and-Drop
Are you looking to add a touch of interactivity to your NEXT.js application? Drag and drop functionality can be a great way to enhance user experience, and with React DnD, it’s easier than ever to implement. In this blog post, we’ll guide you through the process of integrating drag and drop features into your NEXT.js project with React DnD.
Table of Contents
1. What is React DnD?
React DnD, short for React Drag and Drop, is a popular library that provides a simple and flexible way to add drag and drop capabilities to your React applications. It’s built on top of the HTML5 drag and drop API and works seamlessly with NEXT.js. Let’s dive in and see how you can get started.
2. Setting Up Your NEXT.js Project
Before we start implementing drag and drop, make sure you have a NEXT.js project up and running. If you haven’t already, you can create a new NEXT.js app using the following command:
```bash npx create-next-app my-drag-and-drop-app ```
Once your project is set up, navigate to the project directory and install React DnD:
```bash npm install react-dnd react-dnd-html5-backend ```
3. Creating a Drag-and-Drop Component
Now that we have our project set up, let’s create a simple drag-and-drop component. We’ll create a list of items that can be dragged and dropped within the same list. First, create a new file named `DragAndDropList.js` in your components folder.
```javascript // components/DragAndDropList.js import React from 'react'; import { useDrag, useDrop } from 'react-dnd'; const ItemTypes = { CARD: 'card', }; const DragAndDropList = ({ items }) => { // Your drag-and-drop logic here }; export default DragAndDropList; ```
In the above code, we’ve imported `useDrag` and `useDrop` hooks from `react-dnd`. These hooks will help us manage the drag-and-drop behavior.
4. Implementing Drag-and-Drop Logic
Now, let’s implement the drag-and-drop logic inside our `DragAndDropList` component.
```javascript // components/DragAndDropList.js // ... const DragAndDropList = ({ items }) => { const [, ref] = useDrop({ accept: ItemTypes.CARD, }); return ( <div ref={ref}> {items.map((item, index) => { const [, cardRef] = useDrag({ type: ItemTypes.CARD, item: { index }, }); return ( <div key={index} ref={cardRef} style={{ padding: '8px', backgroundColor: 'lightgray', margin: '4px', cursor: 'move', }} > {item} </div> ); })} </div> ); }; ```
In the code above, we’ve added the `useDrop` hook to our container element, which specifies that it can accept items of type `CARD`. Each item within the list is wrapped with the `useDrag` hook, indicating that it can be dragged and its index is stored as data.
5. Using the Drag-and-Drop Component
Now that we have our `DragAndDropList` component, let’s use it in our NEXT.js pages. Suppose you have a page called `DragAndDropPage.js`.
```javascript // pages/DragAndDropPage.js import React from 'react'; import DragAndDropList from '../components/DragAndDropList'; const items = ['Item 1', 'Item 2', 'Item 3', 'Item 4']; const DragAndDropPage = () => { return ( <div> <h1>Drag and Drop Example</h1> <DragAndDropList items={items} /> </div> ); }; export default DragAndDropPage; ```
In this example, we’ve imported our `DragAndDropList` component and passed an array of items to it. You can customize the list items and styling as needed for your project.
6. Testing Your Drag-and-Drop Feature
Now, when you run your NEXT.js app and navigate to the `DragAndDropPage`, you should see a list of items that you can drag and drop within the list.
Congratulations! You’ve successfully implemented drag and drop functionality in your NEXT.js application using React DnD.
7. Further Resources
To deepen your understanding and explore more advanced features of React DnD, here are some external resources you may find helpful:
- React DnD Documentation – https://react-dnd.github.io/react-dnd/docs/overview: The official documentation for React DnD provides in-depth information and examples.
- GitHub Repository – https://github.com/react-dnd/react-dnd: Check out the source code and open issues or contribute to the development of React DnD.
- Drag and Drop HTML5 API – https://developer.mozilla.org/en-US/docs/Web/API/HTML_Drag_and_Drop_API: Understanding the HTML5 drag and drop API can provide valuable insights into how React DnD works under the hood.
In this blog post, we covered the basics of implementing drag and drop functionality in NEXT.js with React DnD, provided a step-by-step guide, and included external resource links for further exploration. If you have any questions or need more details on specific aspects, feel free to reach out. Happy coding!
Table of Contents
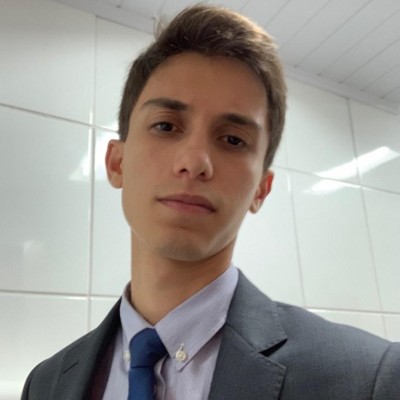
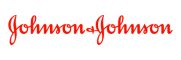