Building an E-commerce Store with NEXT.js and Snipcart
In the ever-evolving world of online commerce, creating a user-friendly and efficient E-commerce store is crucial for success. Two technologies that have gained significant popularity in recent years are NEXT.js and Snipcart. NEXT.js, a React framework for building web applications, provides server-side rendering and excellent performance out of the box. Snipcart, on the other hand, is a powerful e-commerce shopping cart platform that enables developers to integrate customizable and secure e-commerce functionality into their websites with ease. In this tutorial, we’ll guide you through the process of building an E-commerce store using the synergy of NEXT.js and Snipcart.
1. Prerequisites
Before we dive into the tutorial, make sure you have the following prerequisites:
- Basic knowledge of JavaScript, React, and HTML/CSS.
- Node.js and npm (Node Package Manager) installed on your machine.
- A code editor of your choice (e.g., Visual Studio Code).
2. Getting Started
2.1. Setting Up the Project
The first step is to set up a new NEXT.js project. Open your terminal and run the following commands:
bash npx create-next-app my-ecommerce-store cd my-ecommerce-store
This will create a new NEXT.js project in a folder named my-ecommerce-store.
2.2. Installing Snipcart
To integrate Snipcart into your project, you need to sign up for a Snipcart account and obtain your API key. Once you have your API key, install Snipcart’s JavaScript SDK by running:
bash npm install snipcart
Next, open the pages/_app.js file and add the following code to import and initialize Snipcart with your API key:
javascript import { useEffect } from 'react'; import '../styles/globals.css'; function MyApp({ Component, pageProps }) { useEffect(() => { const script = document.createElement('script'); script.src = 'https://cdn.snipcart.com/themes/v3.2.1/default/snipcart.js'; script.setAttribute('data-api-key', 'YOUR_SNIPCART_API_KEY'); document.head.appendChild(script); }, []); return <Component {...pageProps} />; } export default MyApp;
Replace ‘YOUR_SNIPCART_API_KEY’ with your actual Snipcart API key.
3. Building the Product Listing Page
Now, let’s start building the product listing page. Create a new file pages/products.js and add the following code:
javascript import Head from 'next/head'; import Link from 'next/link'; const products = [ { id: 1, name: 'Product 1', price: 29.99, image: '/product1.jpg', }, { id: 2, name: 'Product 2', price: 39.99, image: '/product2.jpg', }, // Add more products as needed ]; const ProductsPage = () => { return ( <div> <Head> <title>My E-commerce Store - Products</title> </Head> <h1>Products</h1> <div className="product-list"> {products.map((product) => ( <div key={product.id} className="product-card"> <img src={product.image} alt={product.name} /> <h2>{product.name}</h2> <p>${product.price}</p> <button className="snipcart-add-item" data-item-id={product.id} data-item-price={product.price} data-item-image={product.image} data-item-name={product.name} > Add to Cart </button> </div> ))} </div> </div> ); }; export default ProductsPage;
In this code, we’re rendering a list of products with their names, prices, and images. The Snipcart’s snipcart-add-item button allows users to add items to their cart.
4. Building the Cart
To create a cart page where users can view and manage their selected items, create a new file pages/cart.js and add the following code:
javascript import Head from 'next/head'; const CartPage = () => { return ( <div> <Head> <title>My E-commerce Store - Cart</title> </Head> <h1>Your Cart</h1> <div className="snipcart-summary"> <p>Number of items: <span className="snipcart-total-items"></span></p> <p>Total: <span className="snipcart-total-price"></span></p> </div> </div> ); }; export default CartPage;
This code renders a basic cart page with information about the number of items and the total price.
5. Styling the Store
To make the store visually appealing, you can add styles using CSS or a CSS framework of your choice. Create a styles folder in the project root directory and add a global.css file with your styles. Here’s a basic example:
css /* styles/global.css */ body { font-family: Arial, sans-serif; margin: 0; padding: 0; } .product-list { display: flex; justify-content: space-around; flex-wrap: wrap; } .product-card { border: 1px solid #ccc; padding: 1rem; margin: 1rem; text-align: center; } .product-card img { max-width: 100%; height: auto; } .snipcart-summary { text-align: center; margin-top: 2rem; font-size: 1.2rem; }
6. Testing the Store Locally
To test your store locally, run the following command in your terminal:
bash npm run dev
This will start a development server, and you can access your store by visiting http://localhost:3000 in your web browser. You should be able to navigate between the product listing page and the cart page, and items should be added to the cart when clicking the “Add to Cart” button.
7. Deploying the Store
Once you’re satisfied with the functionality and styling of your E-commerce store, it’s time to deploy it to a production environment. There are various hosting options available, such as Vercel, Netlify, and AWS Amplify. Choose the one that best fits your needs and follow their deployment instructions.
Conclusion
Building an E-commerce store with NEXT.js and Snipcart offers a powerful combination of performance, flexibility, and user-friendly shopping cart functionality. By following the steps outlined in this tutorial, you’ve learned how to set up a NEXT.js project, integrate Snipcart for E-commerce capabilities, create product listing and cart pages, and style your store for a polished appearance. With your newfound knowledge, you’re well-equipped to create your own online store and provide an exceptional shopping experience for your customers.
Table of Contents
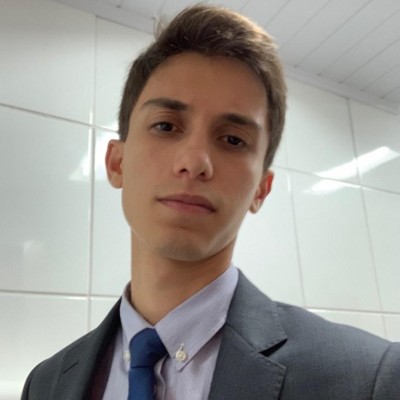
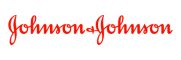