Building E-commerce Websites with NEXT.js and Stripe
In today’s digital age, e-commerce has become an integral part of the business landscape. Building a robust and secure e-commerce website is crucial for any business looking to sell products or services online. In this guide, we will explore how to leverage the power of NEXT.js, a popular React framework, and integrate Stripe, a leading payment gateway, to create an exceptional e-commerce experience.
Why Choose NEXT.js and Stripe for E-commerce Websites?
NEXT.js is a powerful framework for building React applications. It provides server-side rendering (SSR) and static site generation (SSG) capabilities out of the box, making it an excellent choice for e-commerce websites. With NEXT.js, you can create fast and SEO-friendly pages that enhance the user experience.
Stripe, on the other hand, is a popular payment gateway that offers a developer-friendly API and robust security features. It allows you to accept payments from various sources, including credit cards, digital wallets, and bank transfers. Integrating Stripe into your e-commerce website ensures seamless and secure payment processing.
Setting Up Your Development Environment
Before diving into the development process, you need to set up your development environment. Make sure you have Node.js and npm (Node Package Manager) installed on your system. Create a new directory for your project and navigate to it using the terminal.
To initialize a new NEXT.js project, run the following command:
bash npx create-next-app my-ecommerce-app
This command will set up a new NEXT.js project with all the necessary dependencies. Once the command completes, navigate to the project directory:
bash cd my-ecommerce-app
Creating the Project Structure
The project structure plays a crucial role in maintaining code organization and scalability. In the project root, create the following folders:
- pages: Contains all the pages of your e-commerce website.
- components: Houses reusable components.
- styles: Stores CSS styles for your components.
- public: Holds static assets like images and icons.
Building the Product Listing Page
The product listing page is where customers can browse and search for products. Create a new file called products.js in the pages folder. This file will serve as the entry point for the product listing page.
jsx import React from 'react'; const ProductsPage = () => { return ( <div> <h1>Product Listing Page</h1> {/* Add your product listing code here */} </div> ); }; export default ProductsPage;
Within the return statement, you can add the necessary components to display the list of products. You can fetch the product data from an API or use mock data for testing purposes.
Implementing Product Detail Pages
Product detail pages provide detailed information about a specific product. Create a new file called [slug].js in the pages folder. This file will serve as the dynamic route for product detail pages.
jsx import React from 'react'; import { useRouter } from 'next/router'; const ProductDetailPage = () => { const router = useRouter(); const { slug } = router.query; return ( <div> <h1>Product Detail Page - {slug}</h1> {/* Add your product detail code here */} </div> ); }; export default ProductDetailPage;
In this code snippet, we utilize the useRouter hook provided by NEXT.js to access the dynamic route parameter (slug) and fetch the corresponding product data.
Creating the Shopping Cart
The shopping cart allows users to add products, update quantities, and proceed to checkout. Create a new file called Cart.js in the components folder. This component will handle the shopping cart logic.
jsx import React from 'react'; const Cart = () => { // Add your cart logic here return ( <div> <h1>Shopping Cart</h1> {/* Add your cart UI here */} </div> ); }; export default Cart;
Within the return statement, you can design the cart UI and implement functionalities like adding items, updating quantities, and removing items.
Integrating Stripe for Payments
Integrating Stripe into your e-commerce website enables secure payment processing. First, sign up for a Stripe account and retrieve your API keys. Then, install the stripe package by running the following command:
bash npm install stripe
In the component responsible for handling payments (e.g., Checkout.js), import the stripe package and initialize it with your API keys.
jsx import React from 'react'; import Stripe from 'stripe'; const stripe = new Stripe('YOUR_STRIPE_SECRET_KEY'); const Checkout = () => { // Add your checkout logic here return ( <div> <h1>Checkout</h1> {/* Add your checkout UI here */} </div> ); }; export default Checkout;
Implementing Checkout and Order Confirmation
The checkout process involves collecting customer information and processing the payment. You can create a checkout form using React components and handle the payment processing with Stripe.
After a successful payment, you can display an order confirmation page to users. This page should provide a summary of the order and any additional information.
Deploying Your E-commerce Website
Once you’ve completed building your e-commerce website, it’s time to deploy it to a hosting platform. NEXT.js supports various hosting options, including Vercel, Netlify, and AWS Amplify. Choose the hosting platform that best suits your needs and follow their deployment instructions.
Conclusion
In this guide, we explored how to build powerful e-commerce websites using NEXT.js and Stripe. We covered the setup process, creating the project structure, building the product listing and detail pages, implementing the shopping cart, integrating Stripe for payments, and implementing checkout and order confirmation. By leveraging the capabilities of NEXT.js and Stripe, you can create exceptional e-commerce experiences that delight your customers and drive business growth.
Table of Contents
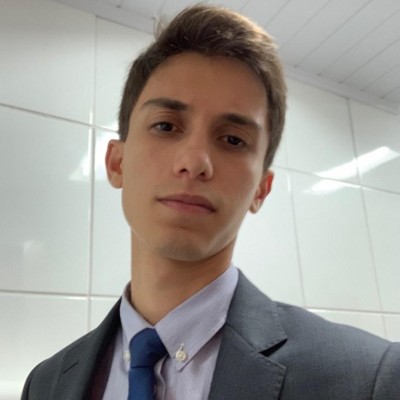
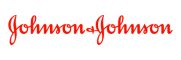