Working with GraphQL in NEXT.js: Integrating Apollo Client
GraphQL has gained significant popularity in recent years as a powerful alternative to traditional RESTful APIs. Its flexibility and efficiency make it an ideal choice for modern web development. When combined with the NEXT.js framework, GraphQL can unlock a whole new level of functionality and performance. In this blog post, we will explore how to integrate Apollo Client, a popular GraphQL client, into a NEXT.js project. We will dive into step-by-step instructions, code samples, and best practices for a seamless integration. So let’s get started!
1. What is GraphQL?
GraphQL is a query language and runtime for APIs that was developed by Facebook. Unlike traditional RESTful APIs, where clients have limited control over the data they receive, GraphQL allows clients to request specific data structures and reduce over-fetching or under-fetching of data. With GraphQL, clients can specify the exact data requirements, which leads to more efficient and flexible communication between the client and the server.
2. Benefits of using GraphQL in NEXT.js
Integrating GraphQL into a NEXT.js application brings several advantages:
2.1. Enhanced performance: GraphQL allows clients to fetch only the data they need, reducing unnecessary network requests and minimizing over-fetching of data. This results in improved performance and faster page loads.
2.2. Flexible data fetching: With GraphQL, clients can combine multiple data requirements into a single request, eliminating the need for multiple round trips to the server. This improves the efficiency of data fetching, especially in scenarios where multiple data sources are involved.
2.3. Strongly typed schemas: GraphQL comes with a strong type system that enables automatic validation of queries and responses. This reduces the chances of runtime errors and enhances the developer experience by providing early feedback.
2.4. Rapid iteration and development: GraphQL’s introspective capabilities allow developers to explore and understand the available data and schemas. This leads to faster development cycles and easier integration with frontend frameworks like NEXT.js.
3. Getting started with Apollo Client
Apollo Client is a powerful and flexible GraphQL client that simplifies working with GraphQL in JavaScript applications. To integrate Apollo Client into a NEXT.js project, follow these steps:
3.1. Installing Apollo Client
Start by installing the required packages:
bash npm install @apollo/client graphql
or
bash yarn add @apollo/client graphql
3.2. Setting up Apollo Provider
Create a file called apolloClient.js and add the following code:
javascript import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://api.example.com/graphql', // Replace with your GraphQL API endpoint cache: new InMemoryCache(), }); export default client;
In your NEXT.js application, wrap your root component with the ApolloProvider component from @apollo/client:
javascript import { ApolloProvider } from '@apollo/client'; import client from './apolloClient'; function MyApp({ Component, pageProps }) { return ( <ApolloProvider client={client}> <Component {...pageProps} /> </ApolloProvider> ); } export default MyApp;
This sets up the Apollo Client and makes it available to all components in your NEXT.js application.
4. Writing GraphQL queries in NEXT.js
To fetch data from a GraphQL server in a NEXT.js application, we need to write GraphQL queries. Here’s how to do it:
4.1. Creating a GraphQL query file
Create a file called myQuery.graphql and define your GraphQL query:
graphql query MyQuery { users { id name email } }
4.2. Executing the query in a component
In a component where you want to fetch and display data, import the necessary components and hooks:
javascript import { gql, useQuery } from '@apollo/client'; const MY_QUERY = gql` query MyQuery { users { id name email } } `; function MyComponent() { const { loading, error, data } = useQuery(MY_QUERY); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div> {data.users.map((user) => ( <div key={user.id}> <h3>{user.name}</h3> <p>{user.email}</p> </div> ))} </div> ); } export default MyComponent;
This example demonstrates how to use the useQuery hook from Apollo Client to fetch and display data in a NEXT.js component.
5. Implementing GraphQL mutations
In addition to fetching data, GraphQL also allows us to modify data on the server. Mutations are used to create, update, or delete data. Here’s how to implement GraphQL mutations in a NEXT.js application:
5.1. Defining a mutation
Create a GraphQL mutation file called createUserMutation.graphql:
graphql mutation CreateUser($input: CreateUserInput!) { createUser(input: $input) { id name email } }
5.2. Executing the mutation
In a component, import the necessary components and hooks:
javascript import { gql, useMutation } from '@apollo/client'; const CREATE_USER_MUTATION = gql` mutation CreateUser($input: CreateUserInput!) { createUser(input: $input) { id name email } } `; function CreateUserForm() { const [createUser, { loading, error }] = useMutation(CREATE_USER_MUTATION); const handleSubmit = async (event) => { event.preventDefault(); const input = { name: event.target.name.value, email: event.target.email.value, }; try { const { data } = await createUser({ variables: { input } }); console.log('New user created:', data.createUser); // Handle success } catch (error) { console.error('Error creating user:', error); // Handle error } }; return ( <form onSubmit={handleSubmit}> <input type="text" name="name" placeholder="Name" required /> <input type="email" name="email" placeholder="Email" required /> <button type="submit" disabled={loading}> {loading ? 'Creating...' : 'Create User'} </button> {error && <p>Error: {error.message}</p>} </form> ); } export default CreateUserForm;
This example demonstrates how to use the useMutation hook from Apollo Client to execute a mutation and handle the loading and error states in a NEXT.js component.
6. Caching and performance optimization
Apollo Client provides a built-in caching mechanism that helps improve the performance of your application. Here are some ways to optimize caching in your NEXT.js application:
6.1. Configuring Apollo Client cache
By default, Apollo Client uses an in-memory cache to store fetched data. You can customize the cache configuration to suit your application’s needs. For example, you can enable data normalization, define custom cache resolvers, or specify cache eviction policies.
javascript import { InMemoryCache } from '@apollo/client'; const cache = new InMemoryCache({ typePolicies: { Query: { fields: { users: { // Custom cache resolver for users field read(existing, { args }) { // Implement your custom logic here }, }, }, }, }, });
6.2. Using Apollo Client DevTools
Apollo Client DevTools is a browser extension that provides a visual representation of the Apollo Client cache and network activity. It allows you to inspect queries, mutations, and cache updates, helping you debug and optimize your GraphQL operations.
7. Handling authentication with Apollo Client
To handle authentication with Apollo Client in a NEXT.js application, you can use various techniques such as setting authentication headers, persisting tokens in local storage, or using authentication libraries like next-auth. Implementing authentication is specific to your application and outside the scope of this blog post, but Apollo Client provides the necessary tools to integrate with your chosen authentication mechanism.
8. Error handling and loading states
Apollo Client handles loading and error states for you. The useQuery and useMutation hooks return loading and error states that you can use to display loading spinners or error messages in your components.
9. Best practices and tips
- Organize your GraphQL queries and mutations in separate files to improve code readability and maintainability.
- Use the Apollo Client cache effectively to reduce unnecessary network requests and improve performance.
- Leverage the power of Apollo Client DevTools for debugging and optimizing your GraphQL operations.
- Consider using TypeScript to take advantage of GraphQL’s strong type system and enhance the development experience.
- Follow GraphQL best practices when designing your schema and resolvers to ensure a well-structured and efficient API.
Conclusion
Integrating Apollo Client into your NEXT.js application opens up a world of possibilities for leveraging the power and flexibility of GraphQL. In this blog post, we explored step-by-step instructions, code samples, and best practices for working with GraphQL in NEXT.js. From setting up Apollo Client to executing queries and mutations, we covered the essential aspects of integrating GraphQL into your NEXT.js projects. Remember to optimize caching, handle authentication, and follow best practices to build robust and performant applications. With GraphQL and Apollo Client, you can take your NEXT.js development to the next level. Happy coding!
Table of Contents
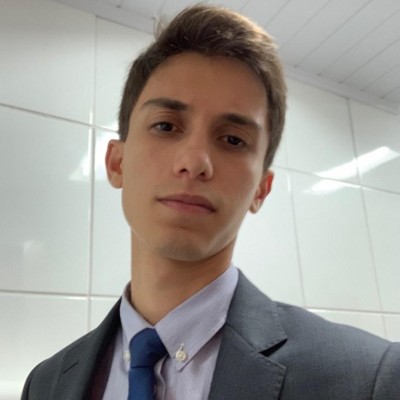
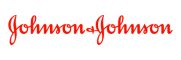