Exploring Next.js: A Step-by-Step Guide to Building Your First Application
Next.js is a powerful open-source JavaScript framework built on top of React.js. It’s designed for building server-rendered and/or static websites and apps, making it a great choice for modern web development. Because of its robust features, many businesses decide to hire Next.js developers for creating performant, scalable applications. In this comprehensive guide, we will explore Next.js and create a basic application, giving you a clear understanding of its features and showing you why it’s beneficial to consider hiring experienced Next.js developers for your projects.
Introduction to NEXT.js
Next.js was launched by Vercel (previously Zeit) and has since attracted a considerable following due to its robust features, including server-side rendering, static site generation, hot code reloading, automatic routing, and code splitting. All these features improve performance, SEO, and developer experience.
React.js provides the flexibility of client-side rendering. However, it lacks out-of-the-box support for server-side rendering or static site generation. That’s where Next.js steps in, providing a complete solution to build scalable, high-performance React applications.
Setting Up Your Development Environment
To get started with Next.js, you need to have Node.js and npm (node package manager) installed in your system. If you don’t have these, you can download and install them from the official Node.js website. Node.js comes with npm, so you get both. If the setup process seems complex, or if you want to save time and ensure your project is set up professionally from the start, you might want to hire Next.js developers. These experts have a deep understanding of the environment setup and can help you hit the ground running with your Next.js project.
Once installed, you can check their versions using the following commands in your terminal or command prompt:
```bash node -v npm -v ```
You should see the installed versions of Node.js and npm respectively.
Creating a New NEXT.js Application
Once the environment setup is complete, we can create a new Next.js application.
Create a new directory for your project and navigate into it:
```bash mkdir next-app cd next-app ```
Then, you can set up a new Next.js app using `create-next-app`:
```bash npx create-next-app . ```
This will create a new Next.js application in your `next-app` directory.
Understanding the File Structure
A default Next.js app comes with a standard file structure:
– `pages/` : This directory contains your application’s pages. Each file corresponds to a route based on its filename. For example, `pages/index.js` maps to the ‘/’ route.
– `public/` : This is where you store static files, like images, which can be served from the root of the application.
– `styles/` : This is where your CSS styles go.
– `package.json` : This is your npm configuration file, containing all the dependencies for your project.
Creating Your First Page
Let’s create our first page. In Next.js, adding a new page is as simple as adding a new file in the `pages` directory. Let’s create a `about.js` file:
```bash touch pages/about.js ```
In `about.js`, let’s add a simple functional component:
```jsx export default function About() { return ( <div> <h1>About Page</h1> <p>This is the about page of our Next.js app.</p> </div> ) } ```
To view this page, start your Next.js app:
```bash npm run dev ```
This will start the development server. Open a browser and navigate to `http://localhost:3000/about`, and you should see your new about page.
Adding Navigation
Next.js comes with a built-in `Link` component for navigation. To add navigation to our about page, we’ll need to modify `pages/index.js`:
```jsx import Link from 'next/link' export default function Home() { return ( <div> <h1>Home Page</h1> <Link href="/about"> <a>About</a> </Link> </div> ) } ```
This creates a link to the about page from the home page.
Server-Side Rendering (SSR)
One of the standout features of Next.js is server-side rendering (SSR). To understand SSR, let’s create a new page, `randomUser.js`, which fetches a random user from the API `https://randomuser.me/api`:
```jsx import fetch from 'isomorphic-unfetch' export async function getServerSideProps() { const res = await fetch('https://randomuser.me/api') const data = await res.json() return { props: { user: data.results[0] } } } export default function RandomUser({ user }) { return ( <div> <h1>Random User</h1> <div> <h2>{user.name.first} {user.name.last}</h2> <p>Email: {user.email}</p> </div> </div> ) } ```
Here, `getServerSideProps` fetches the data on each request from the server side. The data is then passed to our `RandomUser` component as props.
Static Site Generation (SSG)
Next.js also supports static site generation (SSG), where it generates static HTML at build time. Let’s create a page `staticUser.js`:
```jsx import fetch from 'isomorphic-unfetch' export async function getStaticProps() { const res = await fetch('https://randomuser.me/api') const data = await res.json() return { props: { user: data.results[0] } } } export default function StaticUser({ user }) { return ( <div> <h1>Static User</h1> <div> <h2>{user.name.first} {user.name.last}</h2> <p>Email: {user.email}</p> </div> </div> ) } ```
In this example, `getStaticProps` fetches the data at build time, and the HTML is generated statically.
Conclusion
We’ve just scratched the surface of what’s possible with Next.js in this guide. We learned how to create a new Next.js app, add pages, use server-side rendering and static site generation, and much more. The power and flexibility of Next.js make it an excellent platform, not just for beginners, but also for experienced developers. If you’re looking to build a robust, scalable application, you may want to hire Next.js developers to leverage their expertise. The beauty of Next.js is its simplicity and versatility, making it a great tool in the arsenal of any developer. To delve deeper, explore the official Next.js documentation for more in-depth knowledge.
Table of Contents
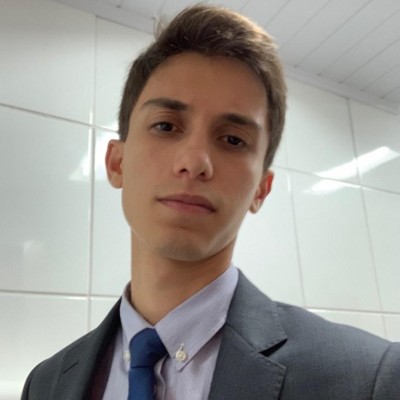
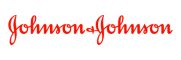