Exploring Next.js Incremental Static Regeneration (ISR)
In the ever-evolving world of web development, staying up-to-date with the latest technologies is crucial. One of the recent breakthroughs that has revolutionized the way we build and optimize websites is Next.js Incremental Static Regeneration (ISR). If you’re looking to boost your website’s performance, improve user experience, and streamline your development process, then you’ve come to the right place.
1. What is Incremental Static Regeneration (ISR)?
Incremental Static Regeneration (ISR) is a cutting-edge feature introduced in Next.js that allows you to generate static pages on-demand, at runtime. This means you can have the best of both worlds: the blazing-fast load times of static sites and the dynamic content updates of server-rendered apps.
1.1. How Does ISR Work?
At the core of ISR is the concept of revalidating and regenerating specific pages when needed. When a user requests a page, Next.js will check if it’s time to regenerate that page based on a specified interval. If the page is stale (i.e., hasn’t been regenerated recently), Next.js will automatically generate a new static version of that page, and the user will be served the most up-to-date content.
To illustrate this, let’s take a look at a simple code example:
jsx // pages/products/[id].js import { getProductById } from '../../lib/api'; export async function getStaticPaths() { // Fetch a list of product IDs from your data source const productIds = await fetchProductIds(); // Generate paths for each product ID const paths = productIds.map((id) => ({ params: { id }, })); return { paths, fallback: 'blocking', // Enable ISR with "blocking" fallback }; } export async function getStaticProps({ params }) { const product = await getProductById(params.id); return { props: { product }, revalidate: 60 * 60, // Revalidate and regenerate this page every hour }; } export default function ProductPage({ product }) { return ( <div> <h1>{product.name}</h1> <p>{product.description}</p> </div> ); }
In this example, we have a product page that uses ISR. Here’s a breakdown of the key parts:
- getStaticPaths: This function generates the paths for all product pages. It specifies the fallback option as ‘blocking’, indicating that Next.js should use ISR for these pages.
- getStaticProps: This function fetches the product data for a specific ID and sets a revalidation time of one hour.
- fallback: ‘blocking’: This tells Next.js to use ISR for this page. If a user requests a product page that hasn’t been generated yet, Next.js will generate it on-demand (hence, “incremental”) and serve it once it’s ready.
With ISR, your website remains fast and responsive, even with frequently changing content. Now, let’s dive deeper into the advantages of using ISR.
2. Benefits of Incremental Static Regeneration
2.1. Improved Performance
One of the most significant advantages of ISR is the tremendous boost in performance. By serving pre-rendered static pages, you reduce server load and minimize the time it takes to load a page. Users experience near-instantaneous page loads, resulting in higher user satisfaction and engagement.
2.2. Seamless User Experience
ISR ensures that users always see the latest content. When a page is regenerated, the changes are immediately reflected, providing a seamless and up-to-date browsing experience. Users won’t encounter stale information or have to wait for the page to update.
2.3. Scalability
With ISR, your website can easily handle traffic spikes and high demand for specific pages. As pages are generated on-demand, your server resources are used efficiently, allowing you to scale your application more effectively.
2.4. SEO Benefits
Search engine optimization is crucial for any website. ISR helps improve your SEO by delivering fast-loading, indexable pages that search engines love. When your website ranks higher in search results, you’ll attract more organic traffic.
2.5. Development Speed
Developers also benefit from ISR. It simplifies the development process by enabling you to work with static files while still having the ability to update content dynamically. This reduces the complexity of your codebase and makes it easier to maintain.
3. Use Cases for ISR
Now that you understand the advantages of ISR, let’s explore some use cases where it shines.
3.1. E-Commerce Websites
E-commerce websites often have a vast catalog of products that can change frequently. ISR allows you to maintain fast load times for product pages while ensuring that customers always see accurate pricing and availability information.
3.2. News and Blog Sites
For news and blog websites, keeping content up-to-date is essential. ISR lets you publish articles, updates, and breaking news instantly without compromising performance.
3.3. Dashboards and Analytics
In applications that display real-time data, ISR can be a game-changer. You can provide users with fresh insights while ensuring rapid page loads.
3.4. Event Listings
Event websites can benefit from ISR by showing the latest event details while maintaining excellent performance, even during ticket sales or registration periods.
5. Implementing ISR in Next.js
Now that you’re eager to harness the power of ISR, let’s walk through how to implement it in your Next.js application.
5.1. Set Up a Next.js Project
If you haven’t already, create a new Next.js project. You can use the following command to set up a new project:
bash npx create-next-app my-nextjs-app
5.2. Enable ISR for a Page
To enable ISR for a specific page, follow these steps:
- Create a new page or choose an existing one.
- Implement the getStaticPaths and getStaticProps functions, as demonstrated in the earlier code example.
5.3. Configure Revalidation Time
In the getStaticProps function, set the revalidate option to specify how often the page should be regenerated. For example, to regenerate the page every 30 minutes, use:
javascript revalidate: 1800 // 30 minutes in seconds
5.4. Run Your Next.js Application
Start your Next.js application using the following command:
bash npm run dev
Your application is now taking advantage of ISR for the specified page(s).
6. Fine-Tuning ISR
While ISR provides significant benefits out of the box, you can fine-tune its behavior to suit your specific needs.
6.1. Custom Loading Indicators
When using ISR with “blocking” fallback, you can create custom loading indicators to display to users while a page is being regenerated. This ensures a smooth user experience, even for pages that take a little longer to generate.
6.2. Adjusting Revalidation Times
Depending on your content update frequency, you can adjust the revalidate time for each page. Pages with frequent updates might benefit from a shorter revalidation time, while others can have longer intervals.
6.3. Handling Error Cases
Consider implementing error handling for cases where regeneration fails. You can provide fallback content or display an error message to users.
Conclusion
Next.js Incremental Static Regeneration is a game-changer in web development. It combines the benefits of static sites with dynamic content updates, resulting in blazing-fast performance and an exceptional user experience. By implementing ISR in your Next.js applications, you can ensure that your websites are not only fast but also always up-to-date, scalable, and SEO-friendly. So, don’t wait—start exploring ISR and unlock its potential for your projects today!
Incorporating ISR into your Next.js applications is a powerful way to enhance user experience and streamline development. Whether you’re building an e-commerce site, a news platform, or a data dashboard, ISR can make your application faster and more dynamic. Start experimenting with ISR today and discover how it can supercharge your Next.js projects. Happy coding!
Table of Contents
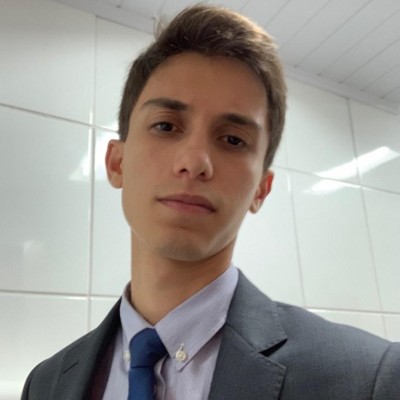
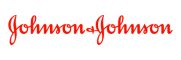