Internationalization and Localization in NEXT.js: Reaching Global Audiences
In today’s interconnected world, the internet has bridged the gap between people from diverse linguistic and cultural backgrounds. To effectively serve a global audience, it is crucial for web applications to provide content in multiple languages and cater to the preferences of users from different regions. This process involves two main concepts: Internationalization (i18n) and Localization (l10n). In this blog, we will explore how NEXT.js, a popular React framework, supports these concepts, and how you can leverage it to create a truly inclusive web application.
1. Understanding Internationalization (i18n) and Localization (l10n)
Before diving into the specifics of how NEXT.js handles internationalization and localization, let’s understand the core concepts:
1.1. Internationalization (i18n)
Internationalization, often abbreviated as i18n, is the process of designing and developing a web application in a way that makes it easy to adapt its content to different languages, regions, and cultures without altering the codebase. The primary goal of i18n is to decouple the content from the code, allowing developers to dynamically change the language displayed to users based on their preferences.
1.2. Localization (l10n)
Localization, or l10n, is the process of adapting the content and user interface of a web application to suit a specific language, region, or culture. Unlike internationalization, which focuses on the technical aspect of enabling multilingual support, localization deals with translating and customizing the application’s content to resonate with local users effectively.
2. Leveraging NEXT.js for Internationalization and Localization
NEXT.js provides built-in support for i18n and l10n, making it easier for developers to reach a global audience. Here’s a step-by-step guide on how to implement these features in your NEXT.js application:
Step 1: Project Setup
Start by creating a new NEXT.js project or using an existing one. You can do this by running the following command:
bash npx create-next-app@12 my-i18n-app cd my-i18n-app
Step 2: Install and Configure Next.js i18n
In recent versions of NEXT.js, the i18n support is provided through the next.config.js file. Add the following code to enable i18n and set up the supported locales:
javascript // next.config.js module.exports = { i18n: { locales: ['en', 'fr', 'es'], // Add your supported locales here defaultLocale: 'en', // Set the default locale for your application }, }
Step 3: Language Switcher Component
To allow users to switch between different languages, create a language switcher component that modifies the locale based on user input. Here’s a simple example:
jsx // components/LanguageSwitcher.js import { useRouter } from 'next/router' const LanguageSwitcher = () => { const router = useRouter() const handleChange = (e) => { const locale = e.target.value router.push(router.pathname, router.asPath, { locale }) } return ( <select onChange={handleChange} defaultValue={router.locale}> <option value="en">English</option> <option value="fr">Français</option> <option value="es">Español</option> </select> ) } export default LanguageSwitcher
Step 4: Translations
Next, create translation files for each supported locale inside a folder named locales in the root of your project. For example, you could have en.json, fr.json, and es.json. These files will contain key-value pairs for each piece of text you want to translate.
Sample en.json:
json { "home.title": "Welcome to My App", "home.subtitle": "The best place to explore and learn.", // Add more translations here }
Step 5: Use Translations in Components
To use the translations in your components, you can leverage the useTranslation hook provided by next-i18next library, which is automatically integrated with NEXT.js:
jsx // components/HomePage.js import { useTranslation } from 'next-i18next' const HomePage = () => { const { t } = useTranslation('common') return ( <div> <h1>{t('home.title')}</h1> <p>{t('home.subtitle')}</p> </div> ) } export default HomePage
Step 6: Dynamic Routes for Localization
In some cases, you might need to create dynamic routes with localized content. For example, if your website displays blog posts, you’ll want each blog post to be available in different languages. Here’s how you can achieve this:
jsx // pages/blog/[slug].js import { useTranslation } from 'next-i18next' const BlogPost = () => { const { t } = useTranslation('common') const router = useRouter() const { slug } = router.query // Fetch blog content based on the slug and language return ( <div> <h1>{t('blog.postTitle')}</h1> <p>{t('blog.postContent')}</p> </div> ) } export default BlogPost
Step 7: Date and Number Formatting
When dealing with dates, numbers, and currencies, different locales have specific formats. You can use the date-fns and react-intl libraries to handle date and number formatting based on the user’s locale:
bash npm install date-fns react-intl
jsx // pages/_app.js import { IntlProvider } from 'react-intl' function MyApp({ Component, pageProps }) { // Get the locale from NEXT.js router const { locale } = useRouter() return ( <IntlProvider locale={locale}> <Component {...pageProps} /> </IntlProvider> ) } export default MyApp
Conclusion
With NEXT.js’ excellent support for internationalization and localization, reaching global audiences has become significantly easier. By following the steps outlined in this blog, you can create a web application that caters to users from diverse backgrounds, languages, and cultures, leading to a more inclusive and user-friendly experience. So, start implementing i18n and l10n in your NEXT.js projects and open the doors to a truly global audience.
Remember, embracing diversity through internationalization and localization not only makes your application accessible to more people but also shows that your brand is committed to fostering inclusivity on the web. Happy coding!
Table of Contents
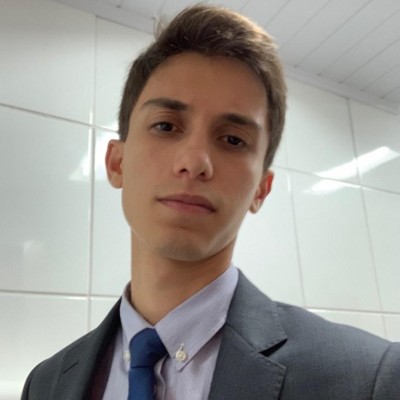
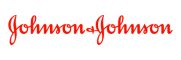