Exploring the NEXT.js Plugin Ecosystem: Tailwind CSS, Redux, and more
In the ever-evolving world of web development, staying ahead of the curve is crucial. NEXT.js, a popular React framework, empowers developers to build high-performance, production-ready applications. But what makes NEXT.js even more impressive is its plugin ecosystem, which allows you to seamlessly integrate a variety of tools and libraries, such as Tailwind CSS and Redux, to enhance your development process.
In this blog post, we will dive deep into the NEXT.js plugin ecosystem, exploring essential plugins like Tailwind CSS and Redux, and showcasing how they can elevate your web development projects. Get ready to supercharge your development workflow with the power of NEXT.js and its versatile plugins.
1. What is NEXT.js?
Before we delve into the exciting world of NEXT.js plugins, let’s briefly discuss what NEXT.js is and why it’s gaining immense popularity in the web development community.
1.1. NEXT.js: A Brief Overview
NEXT.js is an open-source React framework that simplifies the process of building server-rendered React applications. It provides developers with a set of tools and conventions that make it easier to create production-ready applications with features like server-side rendering (SSR), static site generation (SSG), and routing right out of the box.
Here are some key benefits of using NEXT.js:
1.1.1. Improved Performance
NEXT.js optimizes your application for performance by enabling features like code splitting, lazy loading, and automatic tree shaking. This results in faster load times and a smoother user experience.
1.1.2. SEO-Friendly
With server-side rendering and static site generation, NEXT.js makes it easier to create SEO-friendly web applications. Search engines can easily crawl and index your content, improving your site’s visibility in search results.
1.1.3. Routing Made Simple
NEXT.js provides a simple and intuitive routing system, so you can easily define and manage your application’s routes, making navigation a breeze.
1.1.4. Rich Plugin Ecosystem
The NEXT.js plugin ecosystem is vast and diverse, offering a wide range of tools and libraries to enhance your development workflow.
Now that we have a basic understanding of NEXT.js, let’s explore some of the essential plugins that can take your NEXT.js projects to the next level.
2. Tailwind CSS: Styling Simplified
One of the most popular plugins in the NEXT.js ecosystem is Tailwind CSS. Tailwind CSS is a utility-first CSS framework that simplifies the process of designing and styling web applications. It’s a game-changer for developers who want to streamline their styling workflow.
2.1. Installing Tailwind CSS in NEXT.js
To get started with Tailwind CSS in your NEXT.js project, follow these steps:
Step 1: Install Tailwind CSS and Dependencies
bash # Install Tailwind CSS and its dependencies npm install tailwindcss postcss autoprefixer
Step 2: Create a Configuration File
Generate a Tailwind CSS configuration file using the following command:
bash npx tailwindcss init -p
This will create a tailwind.config.js file in your project directory.
Step 3: Configure PostCSS
Edit your project’s postcss.config.js file to include the following:
javascript module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, }, }
Step 4: Create a CSS File
Create a CSS file (e.g., styles.css) in your project directory and import it in your application:
css /* styles.css */ @import 'tailwindcss/base'; @import 'tailwindcss/components'; @import 'tailwindcss/utilities'; /* Other custom styles */
Step 5: Apply Tailwind CSS Classes
Now you can start using Tailwind CSS classes in your components to style your application.
jsx // Example component using Tailwind CSS classes import React from 'react'; const MyComponent = () => { return ( <div className="bg-blue-500 text-white p-4"> This is a Tailwind CSS styled component. </div> ); }; export default MyComponent;
With these simple steps, you can integrate Tailwind CSS into your NEXT.js project and start designing stunning user interfaces effortlessly.
3. Benefits of Using Tailwind CSS with NEXT.js
Tailwind CSS offers several advantages when used with NEXT.js:
3.1. Rapid Prototyping
Tailwind CSS’s utility-first approach allows you to quickly prototype and style your components without writing custom CSS from scratch. This saves you a significant amount of development time.
3.2. Consistent Design
Tailwind CSS enforces a consistent design system across your application, making it easier to maintain and scale your project.
3.3. Responsiveness
Tailwind CSS includes responsive design utilities, making it simple to create mobile-friendly layouts and adapt to various screen sizes.
3.4. Extensibility
Tailwind CSS is highly customizable. You can easily extend or modify the default styles to match your project’s unique design requirements.
4. Redux: State Management Made Easy
State management is a critical aspect of building complex web applications, and Redux is a battle-tested library for managing application state. Integrating Redux into your NEXT.js project can help you efficiently handle data and ensure a seamless user experience.
4.1. Adding Redux to Your NEXT.js Project
Here’s how you can add Redux to your NEXT.js project:
Step 1: Install Redux and Dependencies
bash # Install Redux and React-Redux npm install redux react-redux
Step 2: Create a Redux Store
In your project, create a Redux store by defining reducers and combining them into a root reducer. Here’s an example:
javascript // reducers.js import { combineReducers } from 'redux'; const initialState = { // Define your initial state here }; const rootReducer = (state = initialState, action) => { switch (action.type) { // Define your action types and reducers here default: return state; } }; export default combineReducers({ // Add your reducers here rootReducer, });
Step 3: Configure Redux in Your NEXT.js App
Next, you’ll need to configure Redux in your application. Create a _app.js file in your project if you don’t already have one, and configure Redux as follows:
javascript // _app.js import { Provider } from 'react-redux'; import { store } from '../redux/store'; function MyApp({ Component, pageProps }) { return ( <Provider store={store}> <Component {...pageProps} /> </Provider> ); } export default MyApp;
Step 4: Connect Components to Redux
Now you can connect your components to Redux and start managing state and actions. Use the connect function from react-redux to connect components to the Redux store.
javascript // Example component connected to Redux import React from 'react'; import { connect } from 'react-redux'; const MyReduxComponent = ({ myData }) => { return ( <div> <p>{myData}</p> </div> ); }; const mapStateToProps = (state) => ({ myData: state.rootReducer.myData, // Replace with your state structure }); export default connect(mapStateToProps)(MyReduxComponent);
With Redux integrated into your NEXT.js project, you can easily manage application state and handle complex data interactions.
5. Benefits of Using Redux with NEXT.js
Here are some key benefits of using Redux in your NEXT.js applications:
5.1. Centralized State Management
Redux provides a centralized store to manage your application’s state, making it easier to keep track of data and ensure consistency across components.
5.2. Predictable State Changes
Redux enforces a unidirectional data flow, ensuring that state changes are predictable and traceable, which is especially valuable in large applications.
5.3. Time-Travel Debugging
Redux’s DevTools extension allows for time-travel debugging, enabling you to rewind and replay state changes, making debugging and troubleshooting more efficient.
5.4. Middleware Support
Redux supports middleware, allowing you to add custom logic, such as logging or async actions, to your state management process.
6. Other Essential NEXT.js Plugins
Tailwind CSS and Redux are just the tip of the iceberg when it comes to the NEXT.js plugin ecosystem. Here are a few more essential plugins worth exploring:
6.1. @next/bundle-analyzer
This plugin helps you analyze your bundle size, allowing you to identify and optimize any performance bottlenecks in your application.
bash # Install the bundle analyzer plugin npm install @next/bundle-analyzer
6.2. next-images
The next-images plugin simplifies the process of importing and optimizing images in your NEXT.js application.
bash # Install the next-images plugin npm install next-images
6.3. next-auth
If your application requires authentication, the next-auth plugin provides a comprehensive solution with support for various authentication providers.
bash # Install the next-auth plugin npm install next-auth
6.4. next-seo
SEO is crucial for your application’s visibility. The next-seo plugin simplifies SEO optimization by providing easy-to-use components and utilities.
bash # Install the next-seo plugin npm install next-seo
6.5. next-i18next
For internationalization and localization, the next-i18next plugin offers a powerful solution with support for multiple languages and translation management.
bash # Install the next-i18next plugin npm install next-i18next
Conclusion
The NEXT.js plugin ecosystem is a treasure trove of tools and libraries that can significantly enhance your web development projects. Whether you want to streamline your styling with Tailwind CSS, manage state seamlessly with Redux, optimize performance, or handle authentication and SEO, there’s a plugin to meet your needs.
As you explore and integrate these plugins into your NEXT.js projects, you’ll discover how they can boost your productivity, improve application performance, and help you build robust, production-ready applications with ease. So, roll up your sleeves, dive into the ecosystem, and take your NEXT.js development to the next level!
Table of Contents
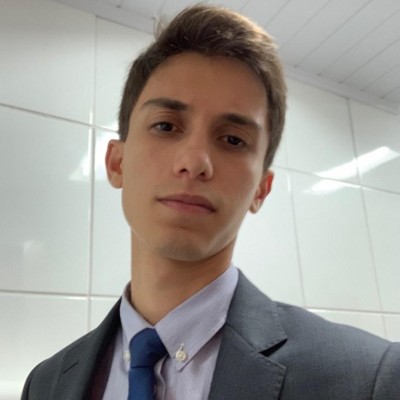
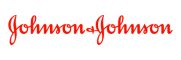