Scroll No More: NEXT.js Pagination for Seamless Data Navigation
In the fast-paced world of web development, creating a seamless and efficient user experience is paramount. One common requirement for achieving this is implementing pagination, especially when dealing with large sets of data. If you’re building a web application using NEXT.js and React, you’re in luck. In this article, we’ll dive into how to implement pagination using the popular library, React Paginate.
Table of Contents
1. What is Pagination?
Pagination is a technique used to break down a large dataset into smaller, manageable chunks. It allows users to navigate through these chunks of data, typically displayed as pages, making it easier to consume and interact with the content.
2. Why Use React Paginate?
React Paginate is a flexible and user-friendly pagination library for React applications. It simplifies the process of adding pagination to your web application by providing a customizable and responsive component. With React Paginate, you can quickly implement a pagination system that aligns with the aesthetics and functionality of your project.
3. Getting Started with NEXT.js and React Paginate
Before diving into the implementation, make sure you have NEXT.js set up in your project. If you haven’t already, you can follow the official documentation for NEXT.js to get started.
Step 1: Installation
First, install React Paginate using npm or yarn:
```bash npm install react-paginate # or yarn add react-paginate ```
Step 2: Importing
Import the necessary components and styles in your NEXT.js page:
```javascript import React, { useState } from 'react'; import ReactPaginate from 'react-paginate'; import './pagination.css'; // Import the styles (you can customize this) ```
Step 3: Implementing Pagination
Now, let’s create a simple example of implementing pagination in your NEXT.js application. Suppose you have a list of items you want to paginate, such as a list of blog posts.
```javascript const itemsPerPage = 10; // Number of items to display per page const data = [...]; // Your data array const PaginatedList = () => { const [currentPage, setCurrentPage] = useState(0); const handlePageClick = (selectedPage) => { setCurrentPage(selectedPage.selected); }; const offset = currentPage * itemsPerPage; const paginatedData = data.slice(offset, offset + itemsPerPage); return ( <div> {/* Render your paginated data here */} {paginatedData.map((item) => ( <div key={item.id}>{item.title}</div> ))} {/* Pagination component */} <ReactPaginate pageCount={Math.ceil(data.length / itemsPerPage)} pageRangeDisplayed={5} marginPagesDisplayed={2} onPageChange={handlePageClick} containerClassName={'pagination'} activeClassName={'active'} /> </div> ); }; export default PaginatedList; ```
In the code above, we define the number of items to display per page (`itemsPerPage`) and the data you want to paginate (`data`). We use the `useState` hook to manage the current page state and update it when a user clicks on a pagination button.
The `handlePageClick` function is called when a page is clicked, and it updates the current page state accordingly. We calculate the `offset` to slice the data array and display the corresponding items on the current page.
Step 4: Styling
Don’t forget to style your pagination component to match your project’s aesthetics. You can customize the styles in the `pagination.css` file imported earlier.
Conclusion
In this tutorial, we’ve explored how to implement pagination in a NEXT.js application using React Paginate. Pagination is a crucial feature when dealing with large datasets, as it enhances user experience and makes data navigation more manageable. With React Paginate, you can easily create a responsive and customizable pagination system for your web application.
Start improving your user experience today by implementing pagination in your NEXT.js project with React Paginate!
External References:
- React Paginate GitHub Repository – https://github.com/AdeleD/react-paginate
- NEXT.js Official Documentation – https://nextjs.org/docs/getting-started/introduction
- Web UI Pagination Design Trends – https://uxdesign.cc/web-ui-pagination-design-trends-e0f65b3a3200
Table of Contents
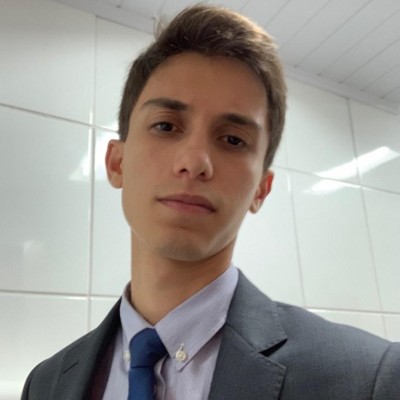
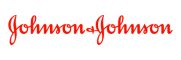