Building a Real-time Chat App with NEXT.js and Pusher
In today’s interconnected world, real-time communication has become a cornerstone of modern web applications. From messaging platforms to collaborative tools, real-time chat apps play a pivotal role in enhancing user engagement and experience. In this tutorial, we’ll explore how to build a real-time chat app using NEXT.js, a popular React framework for building server-rendered applications, and Pusher, a powerful real-time communication platform. By the end of this guide, you’ll have a solid understanding of how to create a dynamic and responsive chat application.
1. Prerequisites
Before we dive into the coding process, make sure you have the following prerequisites installed on your system:
- Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed. You can download them from the official Node.js website.
- Pusher Account: Sign up for a free account on the Pusher platform (https://pusher.com/) to obtain the necessary credentials for real-time communication.
- Basic knowledge of React: Familiarity with React concepts will be beneficial, as NEXT.js is built on top of React.
2. Setting Up the Project
Let’s begin by setting up the project structure and installing the required dependencies.
Create a New NEXT.js Project:
1. Open your terminal and run the following commands:
bash npx create-next-app@12 chat-app cd chat-app
2. Install Required Dependencies:
In your project directory, install the Pusher package using npm:
bash npm install pusher-js
3. Creating the Chat Interface
The first step is to create a simple chat interface where users can send and receive messages in real-time.
3.1. Create Components:
Inside the components directory of your project, create two new files: ChatApp.js and Message.js.
jsx // components/ChatApp.js import React, { useState } from 'react'; import Message from './Message'; const ChatApp = () => { const [messages, setMessages] = useState([]); // Add message sending logic here return ( <div className="chat-app"> <div className="messages"> {messages.map((message, index) => ( <Message key={index} message={message} /> ))} </div> {/* Add message input and sending button */} </div> ); }; export default ChatApp; jsx // components/Message.js import React from 'react'; const Message = ({ message }) => { return <div className="message">{message.text}</div>; }; export default Message;
3.2. Styling:
Create a basic CSS file (e.g., styles.css) in the styles directory to style the chat interface.
css /* styles/styles.css */ .chat-app { width: 100%; max-width: 400px; margin: 0 auto; } .messages { height: 300px; overflow-y: scroll; border: 1px solid #ccc; padding: 10px; } .message { padding: 5px; margin: 5px 0; background-color: #f0f0f0; border-radius: 5px; }
3.3. Integrating Pusher:
Now, let’s integrate Pusher for real-time communication. Open the ChatApp.js file and add the following code to establish a connection with Pusher and receive messages.
jsx // components/ChatApp.js import React, { useState, useEffect } from 'react'; import pusher from 'pusher-js'; import Message from './Message'; const ChatApp = () => { const [messages, setMessages] = useState([]); useEffect(() => { const pusher = new Pusher('YOUR_PUSHER_APP_KEY', { cluster: 'YOUR_PUSHER_CLUSTER', }); const channel = pusher.subscribe('chat-channel'); channel.bind('message', (data) => { setMessages((prevMessages) => [...prevMessages, data]); }); return () => { pusher.disconnect(); }; }, []); // Add message sending logic here return ( <div className="chat-app"> <div className="messages"> {messages.map((message, index) => ( <Message key={index} message={message} /> ))} </div> {/* Add message input and sending button */} </div> ); }; export default ChatApp;
4. Sending and Receiving Messages
With the real-time communication foundation in place, let’s implement the functionality to send and receive messages.
4.1. Sending Messages:
Update the ChatApp.js component to include an input field and a button for sending messages.
jsx // components/ChatApp.js // ... Previous code const ChatApp = () => { const [messages, setMessages] = useState([]); const [newMessage, setNewMessage] = useState(''); const handleSendMessage = () => { if (newMessage.trim() !== '') { // Send the message to Pusher // Clear the input field setNewMessage(''); } }; useEffect(() => { // Pusher integration code }, []); return ( <div className="chat-app"> <div className="messages"> {messages.map((message, index) => ( <Message key={index} message={message} /> ))} </div> <div className="message-input"> <input type="text" value={newMessage} onChange={(e) => setNewMessage(e.target.value)} placeholder="Enter your message..." /> <button onClick={handleSendMessage}>Send</button> </div> </div> ); }; export default ChatApp;
4.2. Receiving Messages:
On the server-side, you’ll need an endpoint that handles incoming messages and sends them to the appropriate channel.
jsx // pages/api/messages.js import Pusher from 'pusher'; export default async (req, res) => { if (req.method === 'POST') { const { text } = req.body; // Store the message in a database (optional) // Trigger the 'message' event on the 'chat-channel' in Pusher const pusher = new Pusher({ appId: 'YOUR_PUSHER_APP_ID', key: 'YOUR_PUSHER_APP_KEY', secret: 'YOUR_PUSHER_APP_SECRET', cluster: 'YOUR_PUSHER_CLUSTER', useTLS: true, }); await pusher.trigger('chat-channel', 'message', { text }); return res.status(200).json({ status: 'Message sent successfully' }); } return res.status(405).json({ error: 'Method not allowed' }); };
Conclusion
Congratulations! You’ve successfully built a real-time chat app using NEXT.js and Pusher. By combining the power of modern web technologies and real-time communication, you’ve created a dynamic and interactive chat experience for users. This tutorial serves as a solid foundation for further customization and enhancement of your chat app.
Throughout this guide, you’ve learned how to set up a NEXT.js project, integrate Pusher for real-time communication, create a chat interface, and implement sending and receiving messages. This is just the beginning of what you can achieve with real-time features in web applications. Feel free to explore additional features like user authentication, message persistence, and group chats to further enhance your chat app’s functionality.
Remember to refer to the official documentation of NEXT.js and Pusher for more advanced features and customization options. Happy coding and building your own real-time chat applications!
Table of Contents
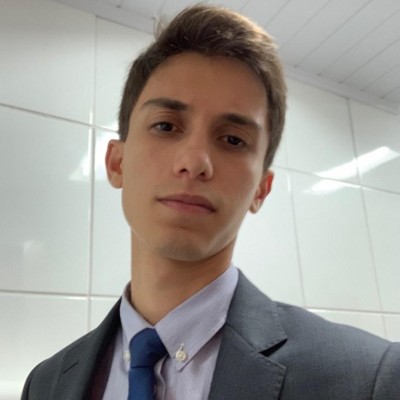
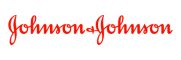