Maximize Audience Interaction: Real-Time Polling with Pusher Channels
In the fast-paced world of technology, real-time applications have become the norm. Whether it’s live chat, notifications, or polling apps, users expect information to update instantly. In this article, we’ll walk you through the process of building a real-time polling app using NEXT.js and Pusher Channels. By the end of this tutorial, you’ll have a fully functional polling app that keeps your audience engaged.
Table of Contents
1. Prerequisites
Before we dive into the development process, make sure you have the following tools and technologies installed:
- Node.js: Ensure you have Node.js installed on your system. You can download it from https://nodejs.org/.
- NEXT.js: If you haven’t already, set up a NEXT.js project by following the official guide https://nextjs.org/docs/getting-started.
- Pusher Channels: Sign up for a Pusher Channels account https://pusher.com/channels to access real-time messaging features.
2. Setting Up Your Project
Let’s start by creating a new NEXT.js project and setting up the necessary dependencies.
- Create a new NEXT.js app:
```bash npx create-next-app polling-app cd polling-app ```
- Install the required packages:
```bash npm install pusher-js axios ```
- Create a Pusher account and get your API keys. You’ll need these to set up real-time communication.
3. Building the Polling App
3.1 Frontend
We’ll create a simple user interface for our polling app. You can customize it further based on your branding and design preferences. Create a new file `pages/index.js` and add the following code:
```jsx import React, { useState } from 'react'; import axios from 'axios'; function PollingApp() { const [question, setQuestion] = useState(''); const [options, setOptions] = useState([]); // Add your polling logic here return ( <div> <h1>Real-time Polling App</h1> <div> <input type="text" placeholder="Enter your question" value={question} onChange={(e) => setQuestion(e.target.value)} /> </div> {/* Add options input fields */} {/* Create a list of options */} <button onClick={/* Create a function to submit the poll */}> Create Poll </button> <div> {/* Display the poll results */} </div> </div> ); } export default PollingApp; ```
In this code snippet, we’ve set up a basic structure for the polling app. Now, let’s implement the real-time functionality using Pusher Channels.
3.2 Backend
Create a new file `pages/api/poll.js` for handling the polling logic on the server-side:
```javascript import Pusher from 'pusher'; import axios from 'axios'; export default async (req, res) => { // Set up Pusher Channels with your API keys if (req.method === 'POST') { const { question, options } = req.body; // Create a new poll and broadcast it using Pusher Channels } else if (req.method === 'GET') { // Retrieve poll results and send them as JSON } }; ```
In this code, we’re using Pusher Channels to handle real-time communication. You should replace `’your-app-id’`, `’your-key’`, and `’your-secret’` with your actual Pusher API credentials.
3.3 Connecting the Frontend and Backend
To connect the frontend and backend, add the following code to the `pages/index.js` file:
```jsx // Import necessary libraries import Pusher from 'pusher-js'; import axios from 'axios'; // Initialize Pusher with your API credentials const pusher = new Pusher({ appId: 'your-app-id', key: 'your-key', secret: 'your-secret', cluster: 'your-cluster', encrypted: true, }); function PollingApp() { // Existing code const createPoll = async () => { try { // Send a POST request to the server to create a new poll await axios.post('/api/poll', { question, options }); // Clear input fields after creating the poll setQuestion(''); setOptions([]); } catch (error) { console.error('Error creating poll:', error); } }; // Existing code } // Export the PollingApp component export default PollingApp; ```
Now, your frontend is connected to the backend, and when a user creates a poll, it will be broadcasted in real-time to all connected clients using Pusher Channels.
Conclusion
In this tutorial, we’ve built a real-time polling app using NEXT.js and Pusher Channels. We’ve covered the frontend and backend setup, as well as the integration with Pusher for real-time communication. You can further enhance this app by adding user authentication, real-time result updates, and more.
Feel free to explore the Pusher Channels documentation: https://pusher.com/docs/channels) for more advanced features and customization options.
Start engaging your audience with real-time polls today and watch your brand and culture thrive as you provide interactive experiences for your users.
Additional Resources:
- Node.js Official Website – https://nodejs.org/)
- NEXT.js Getting Started Guide – https://nextjs.org/docs/getting-started
- Pusher Channels Documentation – https://pusher.com/docs/channels
Table of Contents
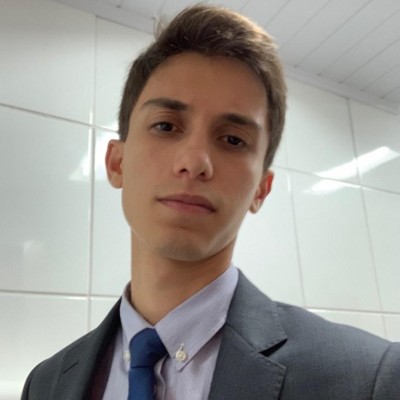
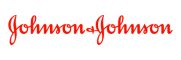