Code Your Culinary Dreams: Creating a Recipe Sharing Platform with NEXT.js
In the fast-paced world of tech startups, innovation is the name of the game. As an early-stage founder or tech leader, you’re constantly looking for ways to outperform the competition. One avenue for success is creating a unique platform that caters to a specific niche, and today, we’re going to explore how to build a Recipe Sharing Platform using NEXT.js and MongoDB.
Table of Contents
1. Why a Recipe Sharing Platform?
Before we dive into the technical details, let’s briefly discuss the rationale behind choosing a Recipe Sharing Platform. Food is a universal language, and sharing recipes online has become a thriving community. By creating a platform for recipe enthusiasts, you can tap into a passionate audience and potentially attract all-star talent to your venture.
2. Technology Stack
To bring your Recipe Sharing Platform to life, we’ll leverage NEXT.js for the front-end and MongoDB for the database. NEXT.js is a popular choice due to its server-rendering capabilities, while MongoDB’s flexibility makes it ideal for handling user-generated content.
3. Setting Up Your Development Environment
Install Node.js: Ensure you have Node.js installed on your system. You can download it from the official website – https://nodejs.org/.
Create a New NEXT.js App: Use the following command to create a new NEXT.js app:
```bash npx create-next-app recipe-sharing-platform ```
Install Required Dependencies: Install the necessary packages, including Next.js, MongoDB, and Mongoose:
```bash npm install next mongoose ```
4. Database Schema
In your Recipe Sharing Platform, you’ll need to define the data structure. Here’s an example schema for recipes:
```javascript const mongoose = require('mongoose'); const recipeSchema = new mongoose.Schema({ title: { type: String, required: true }, ingredients: [String], instructions: String, author: { type: mongoose.Schema.Types.ObjectId, ref: 'User' }, // Add more fields as needed }); module.exports = mongoose.model('Recipe', recipeSchema); ```
5. Building the Front-End
With your database schema in place, it’s time to create the front-end using NEXT.js. You can design the user interface with components like forms for adding recipes, displaying recipes, and user authentication.
6. Connecting to MongoDB
To connect your NEXT.js app to MongoDB, set up a connection using Mongoose. Don’t forget to replace `’your-mongodb-uri’` with your actual MongoDB connection URI.
```javascript const mongoose = require('mongoose'); mongoose.connect('your-mongodb-uri', { useNewUrlParser: true, useUnifiedTopology: true, }); const db = mongoose.connection; db.once('open', () => { console.log('Connected to MongoDB'); }); ```
7. Implementing Features
- User Authentication: You can integrate user authentication using a package like Passport.js, allowing users to create accounts and log in.
- Recipe CRUD Operations: Implement Create, Read, Update, and Delete (CRUD) operations for recipes. Users should be able to add new recipes, view existing ones, edit, and delete their own recipes.
- Search and Filters: Enhance the platform by implementing search functionality and filters to help users discover recipes more effectively.
8. External Resources
- Official NEXT.js Documentation – https://nextjs.org/docs
- MongoDB University – https://university.mongodb.com/
- Passport.js Documentation – http://www.passportjs.org/docs/
Conclusion
Building a Recipe Sharing Platform with NEXT.js and MongoDB is an exciting venture that can attract both users and talent. Remember, a great brand and culture can set you apart in the competitive tech landscape, so keep those aspects in mind as you develop your platform. Happy coding!
Table of Contents
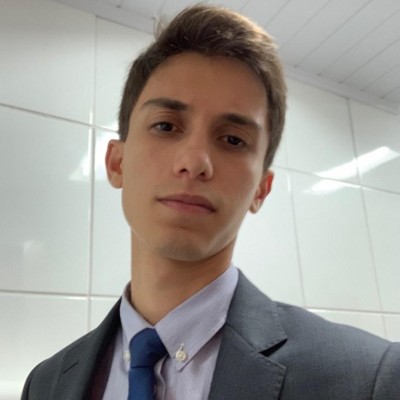
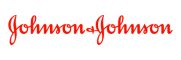