Using NEXT.js with AWS: S3, Lambda, and DynamoDB Integration
In today’s fast-paced digital world, building web applications that are not only performant but also highly scalable is crucial. This is where the combination of NEXT.js, a popular React framework, and Amazon Web Services (AWS) can work wonders. NEXT.js is a React framework that enables server-side rendering, static site generation, and routing out of the box. However, to create web applications that can scale effortlessly, we need more than just a front-end framework. That’s where Amazon Web Services (AWS) steps in, offering a wide array of cloud services to complement NEXT.js.
In this guide, we will focus on three key AWS services: S3 for storing static assets, Lambda for serverless functions, and DynamoDB for NoSQL database storage. By the end of this tutorial, you will be equipped with the knowledge and tools to build high-performance web applications that leverage the power of AWS seamlessly with NEXT.js.
1. Setting Up Your NEXT.js Project
Before diving into AWS integration, let’s set up a basic NEXT.js project if you don’t already have one.
bash npx create-next-app my-nextjs-app cd my-nextjs-app npm run dev
This will create a new NEXT.js project and start a development server at http://localhost:3000. Now that we have our project ready, let’s move on to integrating AWS services.
2. AWS S3: Storing Static Assets
2.1. Setting Up an S3 Bucket
AWS S3 is an object storage service that is perfect for hosting static assets like images, stylesheets, and JavaScript files for your NEXT.js application. Here’s how to set it up:
- Log in to AWS Console: If you don’t have an AWS account, you can create one here.
- Navigate to S3: Once logged in, go to the S3 service in the AWS Console.
- Create a New Bucket: Click the “Create bucket” button and follow the steps to create a new bucket.
- Configure Bucket Settings: Set up your bucket settings, including region and access permissions.
2.2. Uploading Static Assets
Now that you have an S3 bucket, you can upload your static assets. You can use the AWS CLI, SDKs, or even the AWS Console web interface for this. Here’s an example using the AWS CLI:
bash aws s3 cp my-assets/* s3://my-nextjs-bucket/
Make sure to replace my-assets/* with the path to your static assets and my-nextjs-bucket with the name of your S3 bucket.
3. AWS Lambda: Serverless Functions
AWS Lambda allows you to run code without provisioning or managing servers. This makes it an excellent choice for implementing serverless functions in your NEXT.js application.
3.1. Creating a Lambda Function
- Navigate to Lambda: In the AWS Console, go to the Lambda service.
- Create a New Function: Click the “Create function” button.
- Choose Author from Scratch: Select this option to create a custom function.
- Configure Function: Give your function a name, choose Node.js as the runtime, and configure permissions as needed.
- Write Your Function Code: You can write your Lambda function code inline or upload a .zip file containing your code.
Here’s a simple example of a Lambda function that can be used in your NEXT.js application:
javascript exports.handler = async (event) => { // Your code here const response = { statusCode: 200, body: JSON.stringify('Hello from Lambda!'), }; return response; };
Remember to replace the code with your specific functionality.
4. AWS DynamoDB: NoSQL Database
DynamoDB is a managed NoSQL database service provided by AWS. It is highly scalable and can handle large amounts of data with low latency. Let’s set up a DynamoDB table for your NEXT.js application.
4.1. Creating a DynamoDB Table
- Navigate to DynamoDB: In the AWS Console, go to the DynamoDB service.
- Create Table: Click the “Create table” button.
- Configure Table: Provide a table name, primary key, and other settings as needed for your application.
- Review and Create: Review your settings and create the table.
Now you have a DynamoDB table ready to store data for your application.
5. Integrating AWS Services with NEXT.js
With your AWS services set up, it’s time to integrate them into your NEXT.js application. Here are some common integration points:
5.1. Using S3 for Static Assets
In your NEXT.js project, you can reference static assets hosted on S3 by simply providing the S3 bucket URL. For example:
jsx <img src="https://my-nextjs-bucket.s3.amazonaws.com/my-image.jpg" alt="My Image" />
5.2. Invoking Lambda Functions
To invoke your Lambda functions, you can use the AWS SDK for JavaScript. Here’s an example of how to call a Lambda function from your NEXT.js code:
javascript import AWS from 'aws-sdk'; AWS.config.update({ region: 'us-east-1' }); // Set your region const lambda = new AWS.Lambda(); const params = { FunctionName: 'myLambdaFunction', // Replace with your Lambda function name InvocationType: 'RequestResponse', }; lambda.invoke(params, (err, data) => { if (err) { console.error(err); } else { console.log(data.Payload); } });
5.3. Working with DynamoDB
To interact with DynamoDB, you can use the AWS SDK as well. Here’s an example of how to put an item into a DynamoDB table:
javascript const AWS = require('aws-sdk'); AWS.config.update({ region: 'us-east-1' }); // Set your region const docClient = new AWS.DynamoDB.DocumentClient(); const params = { TableName: 'myDynamoDBTable', // Replace with your table name Item: { // Your item data here userId: '123', username: 'john_doe', }, }; docClient.put(params, (err, data) => { if (err) { console.error(err); } else { console.log('Item added to DynamoDB:', data); } });
6. Deploying Your Application
Once you’ve integrated AWS services into your NEXT.js application, it’s time to deploy it to a production environment. There are several hosting options available, including AWS Elastic Beanstalk, AWS Amplify, and even serverless deployments using AWS Lambda and API Gateway.
Choose the hosting option that best suits your project’s requirements and follow the deployment instructions provided by that service.
Conclusion
By integrating NEXT.js with AWS services like S3, Lambda, and DynamoDB, you can create high-performance web applications that are not only scalable but also efficient to develop and maintain. Whether you’re building a content-rich website or a data-driven web app, the combination of NEXT.js and AWS gives you the power to deliver exceptional user experiences.
In this guide, we’ve covered the basics of setting up your NEXT.js project, configuring AWS services, and integrating them into your application. With this knowledge, you can take your web development skills to the next level and build web applications that can handle the demands of the modern digital world. Happy coding!
Table of Contents
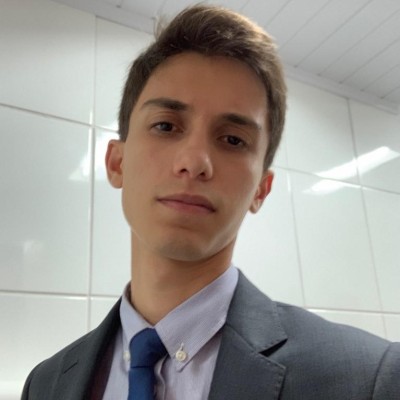
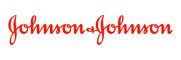