Scaling NEXT.js Apps: Strategies for Handling High Traffic
In today’s digital age, building web applications that can handle high traffic is a crucial aspect of delivering a seamless user experience. With the rise of modern frameworks like NEXT.js, developers have powerful tools at their disposal to create dynamic and interactive applications. However, as user demands grow and your app gains popularity, ensuring it can handle the increased load becomes paramount. In this blog post, we will explore strategies for scaling NEXT.js apps to handle high traffic effectively.
1. Understanding the Scaling Challenge
As your NEXT.js app gains popularity, the demand on your servers increases, leading to potential performance bottlenecks and downtime. Scalability is the ability of your application to handle growing user loads without compromising on performance. When it comes to scaling NEXT.js apps, the challenge lies in distributing the incoming traffic efficiently while maintaining a consistent and smooth user experience. Let’s delve into some strategies to tackle this challenge.
1.1. Server Optimization
1.1.1. Efficient Code and Data Handling
One of the first steps in scaling your NEXT.js app is optimizing your server-side code. Make sure your codebase is well-organized, follows best practices, and avoids unnecessary computations. Minimizing the data transfer between the server and the client can significantly improve your app’s performance. Utilize server-side rendering (SSR) and incremental static regeneration (ISR) to generate and deliver only the necessary content, reducing the server load and improving the time-to-interactive for users.
javascript // Utilizing ISR in NEXT.js export async function getStaticProps() { const data = await fetchData(); return { props: { data }, revalidate: 60, // Re-generate every 60 seconds }; }
1.1.2. Load Balancing
Implementing a load balancing strategy is essential to evenly distribute incoming traffic across multiple servers or instances of your application. This helps prevent any single server from becoming a bottleneck and ensures a more consistent performance, even during high traffic periods. Load balancers like Nginx or cloud-based solutions such as Amazon Elastic Load Balancing (ELB) can intelligently distribute requests, enhancing the overall reliability and scalability of your app.
1.2. Caching for Performance
1.2.1. Client-Side Caching
Caching is a technique that stores frequently accessed data in a temporary storage location, reducing the need to fetch the same data repeatedly. Leverage client-side caching to store assets like images, stylesheets, and scripts locally in the user’s browser. This can significantly reduce the load on your server and enhance page load times.
html <!-- Implementing browser caching --> <link rel="stylesheet" href="styles.css" /> <script src="script.js"></script>
1.2.2. Server-Side Caching
For dynamic content that doesn’t change frequently, server-side caching can provide substantial performance improvements. Utilize caching mechanisms like Redis or Memcached to store rendered pages or API responses. This ensures that the server doesn’t need to regenerate content for every request, leading to faster response times and reduced server load.
javascript // Implementing server-side caching with Redis const redis = require('redis'); const client = redis.createClient(); app.get('/data', async (req, res) => { const cachedData = await client.get('cachedData'); if (cachedData) { return res.json(JSON.parse(cachedData)); } else { const newData = await fetchData(); client.set('cachedData', JSON.stringify(newData)); return res.json(newData); } });
1.3. Content Delivery Networks (CDNs)
1.3.1. What is a CDN?
A Content Delivery Network (CDN) is a globally distributed network of servers that store cached copies of your static assets. CDNs have servers located in various geographic regions, allowing them to deliver content from a server that’s physically closer to the user. This results in reduced latency and faster loading times.
1.3.2. Integrating a CDN with NEXT.js
Integrating a CDN with your NEXT.js app can significantly enhance its performance, especially for users spread across different parts of the world. By caching your static assets on the CDN’s servers, you can offload traffic from your own infrastructure, leading to reduced server load and improved scalability.
javascript // Integrating a CDN for static assets in NEXT.js // next.config.js module.exports = { images: { domains: ['cdn.example.com'], }, };
1.4. Database Optimization
1.4.1. Efficient Queries
Database queries can often be a source of performance bottlenecks, especially during high traffic periods. Optimize your database queries by indexing frequently accessed columns and avoiding unnecessary joins. Consider using an Object-Relational Mapping (ORM) library to streamline your query code and improve database performance.
javascript // Using an ORM to optimize database queries const { User } = require('orm-library'); app.get('/users', async (req, res) => { const users = await User.findAll({ where: { isActive: true, }, }); return res.json(users); });
1.4.2. Connection Pooling
Connection pooling is a technique that maintains a pool of pre-established database connections, reducing the overhead of creating and closing connections for every request. By reusing existing connections, you can improve the efficiency of your application and ensure a smoother experience for users.
javascript // Implementing connection pooling for a database in NEXT.js const { Pool } = require('pg'); const pool = new Pool(); app.get('/data', async (req, res) => { const client = await pool.connect(); try { const result = await client.query('SELECT * FROM data'); return res.json(result.rows); } finally { client.release(); } });
1.5. Horizontal Scaling
1.5.1. What is Horizontal Scaling?
Horizontal scaling involves adding more servers or instances to your infrastructure to distribute the incoming load. This approach allows you to handle increased traffic by simply adding more resources, making it easier to accommodate sudden spikes in user activity.
1.5.2. Implementing Horizontal Scaling
Cloud platforms like Amazon Web Services (AWS) and Microsoft Azure provide tools for easily scaling your applications horizontally. By deploying your NEXT.js app on a cloud-based infrastructure, you can take advantage of auto-scaling groups that automatically adjust the number of instances based on traffic.
yaml # Example configuration for AWS Auto Scaling Group Resources: MyAutoScalingGroup: Type: AWS::AutoScaling::AutoScalingGroup Properties: DesiredCapacity: 2 MaxSize: 4 MinSize: 2 LaunchConfigurationName: MyLaunchConfiguration
Conclusion
Scaling your NEXT.js app to handle high traffic is a multifaceted challenge that requires a combination of server optimization, caching, content delivery networks, database tuning, and horizontal scaling. By implementing these strategies, you can ensure that your application remains responsive, reliable, and capable of delivering a seamless user experience even as your user base grows. As technology continues to advance, staying informed about the latest scaling techniques and best practices will be essential for keeping your app performant in the face of increasing demands.
Table of Contents
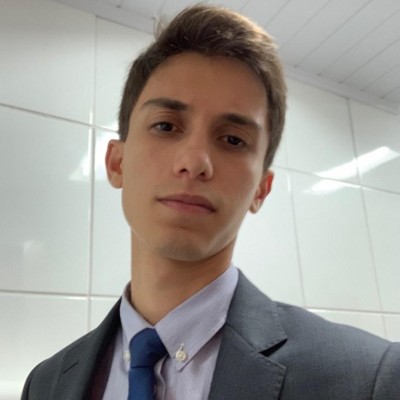
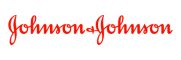