Server-side Caching in NEXT.js: Boosting Performance
In the competitive world of web development, delivering high-performance applications is essential for retaining users and satisfying their expectations. NEXT.js, a popular React framework, is known for its server-side rendering capabilities, which help create fast and SEO-friendly applications. However, even with NEXT.js’s inherent performance benefits, server-side caching can take your app’s speed and responsiveness to the next level.
In this blog post, we will explore the power of server-side caching in NEXT.js and how it can significantly boost your application’s performance. We’ll cover different caching strategies and code examples, equipping you with the knowledge to implement caching techniques tailored to your specific use case.
1. What is Server-side Caching?
Server-side caching is a technique used to store and serve pre-rendered or computed content directly from the server’s memory or storage. Instead of generating the same content repeatedly for each user request, the server caches the content once and delivers it swiftly whenever the same or similar request comes in. This reduces the server load and improves response times, resulting in a more responsive and performant application.
In the context of NEXT.js, which supports server-side rendering, caching becomes a powerful tool to enhance performance. NEXT.js allows us to cache entire pages or specific data fetch operations, thereby reducing the time taken to generate content for each request.
2. Benefits of Server-side Caching in NEXT.js
Let’s explore the benefits of implementing server-side caching in your NEXT.js application:
2.1. Faster Page Load Times
One of the most significant advantages of server-side caching is the considerable improvement in page load times. When a page is cached, subsequent visits by users or search engine crawlers result in lightning-fast load times since the pre-rendered content is served immediately. This reduces the time users spend waiting for content to load, leading to a better overall user experience.
2.2. Reduced Server Load
Server-side caching reduces the server’s workload by serving cached content instead of generating it on every request. As a result, the server can handle more requests with fewer resources, leading to improved scalability and cost efficiency.
2.3. Improved User Experience
With faster load times and reduced waiting periods, users are more likely to stay engaged with your application. Improved user experience directly impacts user retention, bounce rates, and conversions, making caching an essential performance optimization.
3. Server-side Caching Techniques in NEXT.js
Let’s explore various server-side caching techniques you can use in your NEXT.js application:
3.1. Page Level Caching
NEXT.js provides built-in support for page-level caching using the getServerSideProps and getStaticProps functions. These functions allow you to fetch data at build time or request time and pre-render pages with the fetched data. When a user visits a page, the pre-rendered content is served from the cache, reducing the need for frequent data fetches.
To enable caching for a specific page, you can use the revalidate option in getStaticProps, which specifies the time (in seconds) for revalidation. For example, if you set revalidate: 60, the content will be revalidated every 60 seconds, and the cache will be updated if necessary.
jsx // pages/blog/[slug].js import { getServerSideProps } from 'next'; const BlogPost = ({ title, content }) => { return ( <div> <h1>{title}</h1> <p>{content}</p> </div> ); }; export const getServerSideProps = async (context) => { const { slug } = context.query; // Fetch blog post data based on slug from your data source const data = await fetch(`/api/blog/${slug}`); const blogPost = await data.json(); return { props: { title: blogPost.title, content: blogPost.content, }, }; }; export default BlogPost;
In this example, the blog post data is fetched using getServerSideProps, and the page is pre-rendered with the fetched data. The page will be revalidated every time a user visits it, but the actual data fetching will occur only when the cache expires or when a new request arrives.
3.2. Data Fetching with getServerSideProps
The getServerSideProps function is ideal for pages that require real-time data or data that changes frequently. With this approach, the server fetches the data on each request, ensuring users receive the most up-to-date information. However, this can impact the server’s performance if the data source is slow or if the data is requested frequently.
jsx // pages/products/[id].js import { getServerSideProps } from 'next'; const ProductDetails = ({ product }) => { return ( <div> <h1>{product.name}</h1> <p>{product.description}</p> </div> ); }; export const getServerSideProps = async (context) => { const { id } = context.query; // Fetch product details based on id from your data source const data = await fetch(`/api/products/${id}`); const product = await data.json(); return { props: { product, }, }; }; export default ProductDetails;
In this example, the getServerSideProps function fetches product details based on the id from the URL. The data is fetched and served on each request, ensuring that users receive the latest product information.
3.3. Data Fetching with getStaticProps
On the other hand, if your data doesn’t change frequently and can be pre-rendered at build time, you can use getStaticProps. This approach generates static HTML files for each page during the build process and serves them directly from the cache.
jsx // pages/blog/[slug].js import { getStaticPaths, getStaticProps } from 'next'; const BlogPost = ({ title, content }) => { return ( <div> <h1>{title}</h1> <p>{content}</p> </div> ); }; export const getStaticPaths = async () => { // Fetch all blog post slugs from your data source const data = await fetch('/api/blog'); const blogPosts = await data.json(); const paths = blogPosts.map((post) => ({ params: { slug: post.slug }, })); return { paths, fallback: false, // or 'blocking' for incremental static regeneration }; }; export const getStaticProps = async (context) => { const { slug } = context.params; // Fetch blog post data based on slug from your data source const data = await fetch(`/api/blog/${slug}`); const blogPost = await data.json(); return { props: { title: blogPost.title, content: blogPost.content, }, }; }; export default BlogPost;
In this example, getStaticPaths fetches all blog post slugs and generates static paths for pre-rendering. The getStaticProps function then fetches the specific blog post data based on the slug and pre-renders the page with the fetched data during the build process. The resulting HTML files are served from the cache, resulting in faster page loads.
3.4. Stale-While-Revalidate (SWR) Library
The Stale-While-Revalidate (SWR) library is a popular choice for client-side caching in NEXT.js applications. SWR caches data on the client-side, allowing for quick retrieval and display. If the data is stale (i.e., the cache is expired), SWR makes a background request to get fresh data and then updates the cache and UI accordingly.
To use SWR, first, install the library:
bash npm install swr
Then, you can use it in your components like this:
jsx // components/PostList.js import useSWR from 'swr'; const fetcher = (url) => fetch(url).then((res) => res.json()); const PostList = () => { const { data, error } = useSWR('/api/posts', fetcher); if (error) return <div>Failed to load</div>; if (!data) return <div>Loading...</div>; return ( <ul> {data.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }; export default PostList;
In this example, useSWR hooks into the /api/posts endpoint and fetches the data using the fetcher function. The data is then cached on the client-side, and subsequent renders of the component will use the cached data until it expires. When the cache is stale, SWR will automatically revalidate the data and update the UI.
3.5. Cache-Control Headers
Cache-Control headers are a powerful way to control caching behavior on the client-side and proxy servers. You can set these headers in your server’s response to instruct the client and intermediary servers on how to cache the content.
jsx // pages/api/blog/[slug].js const getBlogPostBySlug = (slug) => { // Fetch blog post data from your data source return fetch(`/api/blog/${slug}`).then((res) => res.json()); }; const getCacheControlHeader = () => { // Set the Cache-Control header dynamically based on your criteria const maxAge = 60 * 60; // Cache for 1 hour return `public, max-age=${maxAge}`; }; const handler = async (req, res) => { const { slug } = req.query; const blogPost = await getBlogPostBySlug(slug); res.setHeader('Cache-Control', getCacheControlHeader()); res.json(blogPost); }; export default handler;
In this example, we set the Cache-Control header with a max-age of 1 hour for the blog post endpoint. This tells the client and intermediary servers to cache the response for 1 hour, significantly reducing the number of requests hitting the server.
4. Code Samples for Server-side Caching in NEXT.js
Now that we’ve covered the different server-side caching techniques, let’s provide some code samples to illustrate the implementation.
4.1. Page Level Caching Example
jsx // pages/blog/[slug].js import { getServerSideProps } from 'next'; const BlogPost = ({ title, content }) => { return ( <div> <h1>{title}</h1> <p>{content}</p> </div> ); }; export const getServerSideProps = async (context) => { const { slug } = context.query; // Fetch blog post data based on slug from your data source const data = await fetch(`/api/blog/${slug}`); const blogPost = await data.json(); return { props: { title: blogPost.title, content: blogPost.content, }, }; }; export default BlogPost;
4.2. getServerSideProps Example
jsx // pages/products/[id].js import { getServerSideProps } from 'next'; const ProductDetails = ({ product }) => { return ( <div> <h1>{product.name}</h1> <p>{product.description}</p> </div> ); }; export const getServerSideProps = async (context) => { const { id } = context.query; // Fetch product details based on id from your data source const data = await fetch(`/api/products/${id}`); const product = await data.json(); return { props: { product, }, }; }; export default ProductDetails;
4.3. Data Fetching with getStaticProps Example
jsx // pages/blog/[slug].js import { getStaticPaths, getStaticProps } from 'next'; const BlogPost = ({ title, content }) => { return ( <div> <h1>{title}</h1> <p>{content}</p> </div> ); }; export const getStaticPaths = async () => { // Fetch all blog post slugs from your data source const data = await fetch('/api/blog'); const blogPosts = await data.json(); const paths = blogPosts.map((post) => ({ params: { slug: post.slug }, })); return { paths, fallback: false, // or 'blocking' for incremental static regeneration }; }; export const getStaticProps = async (context) => { const { slug } = context.params; // Fetch blog post data based on slug from your data source const data = await fetch(`/api/blog/${slug}`); const blogPost = await data.json(); return { props: { title: blogPost.title, content: blogPost.content, }, }; }; export default BlogPost;
4.4. Stale-While-Revalidate (SWR) Library Example
jsx // components/PostList.js import useSWR from 'swr'; const fetcher = (url) => fetch(url).then((res) => res.json()); const PostList = () => { const { data, error } = useSWR('/api/posts', fetcher); if (error) return <div>Failed to load</div>; if (!data) return <div>Loading...</div>; return ( <ul> {data.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }; export default PostList;
4.5. Cache-Control Headers Example
jsx // pages/api/blog/[slug].js const getBlogPostBySlug = (slug) => { // Fetch blog post data from your data source return fetch(`/api/blog/${slug}`).then((res) => res.json()); }; const getCacheControlHeader = () => { // Set the Cache-Control header dynamically based on your criteria const maxAge = 60 * 60; // Cache for 1 hour return `public, max-age=${maxAge}`; }; const handler = async (req, res) => { const { slug } = req.query; const blogPost = await getBlogPostBySlug(slug); res.setHeader('Cache-Control', getCacheControlHeader()); res.json(blogPost); }; export default handler;
5. Best Practices for Server-side Caching
To make the most of server-side caching in your NEXT.js application, follow these best practices:
5.1. Determine Cacheable Content
Identify the content that benefits the most from caching. Dynamic data that changes frequently may not be suitable for long-term caching, while static content can be safely cached for longer periods.
5.2. Set the Right Expiry Time
Set appropriate expiry times for your cached content. Short-lived caches are suitable for frequently updated data, while longer-lived caches are ideal for content that changes less often.
5.3. Handle Cache Invalidation
Implement cache invalidation mechanisms to ensure that users receive the latest content when necessary. Depending on the data update frequency, choose a suitable approach, such as using the revalidate option in getStaticProps or getServerSideProps.
5.4. Monitor Cache Performance
Regularly monitor your application’s cache performance to identify potential bottlenecks or issues. Measure the cache hit rate, response times, and server load to fine-tune your caching strategies.
Conclusion
Server-side caching is a powerful technique that can significantly boost the performance of your NEXT.js application. By reducing load times, server requests, and improving user experience, caching helps you deliver a seamless and responsive application.
In this blog post, we explored various server-side caching techniques available in NEXT.js, including page level caching, data fetching with getServerSideProps and getStaticProps, using the SWR library, and setting Cache-Control headers. We also discussed best practices to maximize the benefits of caching.
By implementing these caching strategies and adhering to best practices, you can create blazing-fast NEXT.js applications that leave a lasting positive impression on your users. As you continue to optimize and refine your application, always keep performance in mind and leverage caching as a valuable tool to deliver exceptional user experiences.
Table of Contents
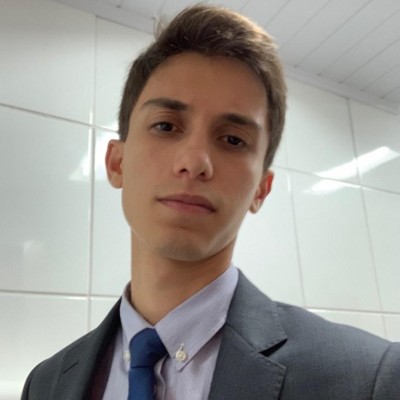
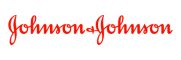