Unlock SEO Dominance: The NEXT.js Guide to Server-side Rendering
In the fast-paced world of web development, delivering a seamless and lightning-fast user experience is a top priority. Server-side rendering (SSR) is a technique that can significantly enhance your web application’s performance and user satisfaction. In this post, we’ll dive deep into SSR, with a focus on implementing it in NEXT.js, a popular React framework.
Table of Contents
1. What is Server-side Rendering (SSR)?
Server-side rendering is a technique used to generate HTML on the server side, rather than in the user’s browser. When a user requests a web page, the server processes the request and sends back a fully rendered HTML page. This contrasts with client-side rendering (CSR), where the initial page load is a blank HTML shell that is later populated with content using JavaScript.
2. Why SSR Matters for SEO and User Experience
SSR offers several advantages, especially for early-stage startup founders, investors, and tech leaders aiming to create a robust online presence:
- Improved SEO: Search engines like Google prefer content that’s readily available in the HTML source code. With SSR, your content is present when the page loads, enhancing your SEO rankings.
- Faster Initial Load: SSR reduces the time it takes for your web pages to become interactive. Users experience quicker load times, which can lead to higher engagement and conversion rates.
- Enhanced User Experience: SSR provides a smoother and more consistent user experience, even on slower internet connections or less powerful devices.
3. Implementing SSR in NEXT.js
NEXT.js makes implementing SSR straightforward. Here’s a step-by-step guide:
3.1. Setting up a NEXT.js Project
To get started, create a new NEXT.js project using the following command:
```bash npx create-next-app my-nextjs-app ```
3.2. Creating a SSR Page
In NEXT.js, pages that require server-side rendering are placed in the `pages` directory. To create an SSR page, simply create a JavaScript file in this directory with the desired route. For example, to create an SSR page at `/about`, create a file named `about.js`:
```javascript // pages/about.js function About({ data }) { return ( <div> <h1>About Us</h1> <p>{data}</p> </div> ); } export async function getServerSideProps() { // Fetch data from an API or database const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data, }, }; } export default About; ```
In this example, we have an `About` component that fetches data using the `getServerSideProps` function, which runs on the server and passes the data as props to the page.
3.3. Running the NEXT.js App
To start your NEXT.js app, run the following command:
```bash npm run dev ```
Now, when you visit `/about`, you’ll see the fully rendered page with the fetched data.
4. External Resources for Further Learning
To deepen your understanding of SSR in NEXT.js, here are three external resources you can explore:
- Official NEXT.js Documentation – https://nextjs.org/docs: The official documentation provides comprehensive information on SSR, routing, and other aspects of NEXT.js.
- React Server Components – https://reactjs.org/server-components: Server Components are a promising addition to the React ecosystem, enabling even more advanced SSR techniques.
- Advanced React SSR Techniques – https://www.smashingmagazine.com/2021/06/advanced-react-ssr-techniques-next-js/: This Smashing Magazine article delves into advanced SSR techniques in NEXT.js for those looking to push the boundaries of performance.
Conclusion
Server-side Rendering (SSR) in NEXT.js is a powerful tool that can help early-stage startup founders, investors, and tech leaders create faster, more SEO-friendly, and user-centric web applications. By following the steps outlined in this post and exploring external resources, you’ll be well on your way to mastering SSR in NEXT.js.
Table of Contents
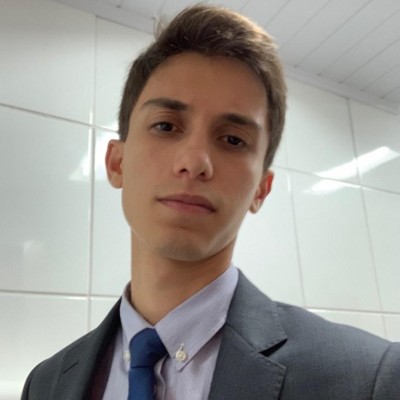
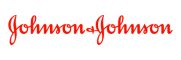