Exploring Serverless Functions in NEXT.js
Serverless architecture has revolutionized the way developers build and deploy applications. It enables developers to focus on writing code without having to worry about managing servers or infrastructure. In the world of serverless, NEXT.js has emerged as a popular framework for building React applications. With its seamless integration of serverless functions, NEXT.js empowers developers to create scalable backend logic with ease.
In this comprehensive guide, we will delve into the world of serverless functions in NEXT.js. We will explore the benefits of serverless architecture, understand how NEXT.js integrates with serverless functions, and dive into practical examples and code samples to demonstrate their power and versatility.
1. What are Serverless Functions?
Serverless functions, also known as Function-as-a-Service (FaaS), are units of computing that execute a specific piece of code in response to an event. Unlike traditional server-based applications, serverless functions do not require permanent server instances to handle incoming requests. Instead, they are executed on-demand and scale automatically based on the workload.
2. Benefits of Serverless Functions in NEXT.js
2.1 Scalability and Cost-Effectiveness
One of the key benefits of serverless functions in NEXT.js is their ability to scale effortlessly. With serverless architecture, the infrastructure automatically provisions resources as needed, ensuring that your application can handle sudden spikes in traffic without any downtime or manual intervention. Moreover, you only pay for the actual execution time and resources consumed, making it a cost-effective solution for applications with variable workloads.
2.2 Simplified Backend Development
By leveraging serverless functions in NEXT.js, developers can focus more on writing business logic rather than managing infrastructure. Serverless functions abstract away the complexities of server management, allowing developers to concentrate on building robust and scalable backend functionality. This simplification of backend development accelerates the development process and improves time-to-market for applications.
2.3 Seamless Integration with NEXT.js
NEXT.js provides a seamless integration with serverless functions, making it a powerful combination for building full-stack applications. NEXT.js allows you to define serverless functions as API routes, enabling you to build custom APIs directly within your NEXT.js application. This integration provides a cohesive development experience and eliminates the need for separate backend services.
3. Setting Up Serverless Functions in NEXT.js
To get started with serverless functions in NEXT.js, you need to set up your development environment. Here’s a step-by-step guide:
3.1 Installing Dependencies
First, ensure that you have Node.js and npm (Node Package Manager) installed on your machine. Then, create a new NEXT.js project using the following command:
shell npx create-next-app my-app
3.2 Configuring the Serverless Environment
Next, install the necessary dependencies to enable serverless functions in your NEXT.js application. Run the following command within your project directory:
shell npm install serverless-http serverless
After installing the dependencies, create a serverless.js file at the root of your project. This file serves as the entry point for serverless deployments. Configure the serverless environment by adding the following code:
javascript const { createServer, proxy } = require('serverless-http'); const { default: app } = require('./.next/serverless/pages/api'); const server = createServer(app); module.exports.nextHandler = (event, context) => proxy(server, event, context);
4. Creating and Deploying Serverless Functions
4.1 Anatomy of a Serverless Function
In NEXT.js, serverless functions are defined as API routes. An API route is a JavaScript file that exports a function. This function receives an incoming HTTP request and returns an HTTP response. Let’s create a basic serverless function that returns a JSON response:
Create a new file hello.js under the pages/api directory:
javascript export default function handler(req, res) { res.status(200).json({ message: 'Hello, Serverless!' }); }
4.2 Handling HTTP Requests
Serverless functions in NEXT.js can handle different types of HTTP requests, such as GET, POST, PUT, DELETE, etc. The type of request is determined by the HTTP method used.
To handle different types of requests, you can modify the serverless function as follows:
javascript export default function handler(req, res) { if (req.method === 'GET') { // Handle GET request res.status(200).json({ message: 'GET request handled.' }); } else if (req.method === 'POST') { // Handle POST request res.status(200).json({ message: 'POST request handled.' }); } else { // Handle other types of requests res.status(405).json({ error: 'Method Not Allowed' }); } }
4.3 Accessing Environment Variables
Serverless functions in NEXT.js can access environment variables, allowing you to store sensitive information securely. You can define environment variables in a .env file at the root of your project.
To access environment variables within a serverless function, use the process.env object:
javascript export default function handler(req, res) { const apiKey = process.env.API_KEY; // Use the apiKey for further processing }
5. Advanced Functionality and Use Cases
5.1 Authentication and Authorization
Serverless functions in NEXT.js can be used to handle authentication and authorization logic. By integrating with authentication providers such as Auth0 or Firebase, you can protect your API routes and ensure that only authorized users can access sensitive data.
5.2 External Service Integrations
Serverless functions can seamlessly integrate with external services and APIs. You can make HTTP requests to external services, retrieve data, perform computations, and return the results as responses to your API calls. This allows you to build powerful and dynamic applications by leveraging third-party services.
5.3 Serverless Function Composition
In complex applications, you might need to compose multiple serverless functions to achieve a specific task. Serverless function composition enables you to combine and orchestrate multiple functions to perform complex operations. This approach promotes code reusability and modular architecture.
6. Best Practices for Serverless Functions in NEXT.js
6.1 Keep Functions Stateless
Serverless functions are designed to be stateless, meaning they should not rely on any server-side state or store any data between invocations. Instead, use external data storage services such as databases or caching solutions to persist and retrieve data as needed.
6.2 Optimize Cold Start Times
Serverless functions experience a cold start time when invoked for the first time or after being idle for a certain period. To optimize performance, minimize cold start times by using serverless function warmers or implementing keep-alive mechanisms.
6.3 Secure Your Functions
Ensure that your serverless functions are secure by implementing appropriate authentication, authorization, and input validation mechanisms. Be mindful of potential security vulnerabilities and follow best practices to protect your application and data.
Conclusion
Serverless functions in NEXT.js provide a powerful and flexible way to handle backend logic in your applications. They offer scalability, cost-effectiveness, and simplified development, making them an ideal choice for building modern web applications.
In this guide, we explored the benefits of serverless functions, learned how to set them up in NEXT.js, created and deployed serverless functions, and examined advanced functionality and best practices. Armed with this knowledge and the provided code samples, you are well-equipped to unlock the full potential of serverless functions in NEXT.js and take your application development to the next level.
Table of Contents
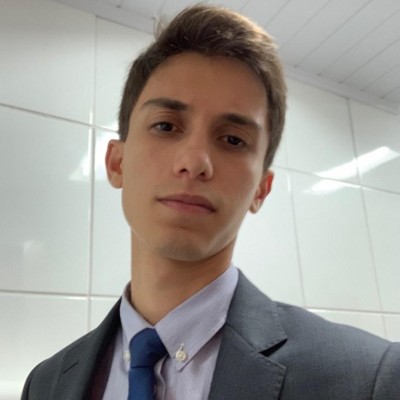
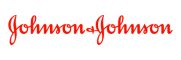