Testing NEXT.js Applications: Strategies and Tools
In the world of web development, Next.js has gained significant popularity due to its robust features and seamless integration with React. As developers strive to build efficient and reliable applications, testing becomes a crucial aspect of the development process. In this blog post, we will explore effective strategies and essential tools for testing Next.js applications. Whether you’re new to Next.js testing or looking to enhance your existing testing practices, this guide will provide you with valuable insights and code samples to ensure the quality and reliability of your projects.
1. The Importance of Testing in Next.js Applications
Next.js applications can be complex, with various components, API routes, and integrations. Testing is essential to ensure that these applications function as intended and deliver a high-quality user experience. It helps identify bugs, ensures code correctness, and improves overall reliability. By investing time and effort into testing, developers can save debugging time in the long run and deliver applications that meet user expectations.
2. Testing Frameworks for Next.js
Next.js supports multiple testing frameworks, but two popular choices are Jest and Testing Library.
2.1. Jest
Jest is a widely used testing framework for JavaScript applications. It provides a simple and intuitive interface, making it suitable for testing Next.js components and API routes. Jest offers features like snapshot testing, code coverage, and powerful mocking capabilities. Its vast ecosystem and community support make it an excellent choice for Next.js developers.
2.2. Testing Library
Testing Library, specifically React Testing Library, is another valuable tool for testing Next.js applications. It promotes a user-centric testing approach by focusing on testing components’ behavior rather than their implementation details. Testing Library encourages developers to write tests that closely resemble how users interact with the application. This approach ensures better test coverage and more robust tests.
3. Unit Testing Next.js Components
Unit testing is the foundation of any testing strategy. With Next.js, unit testing components is straightforward due to its seamless integration with React. Jest provides an excellent platform for writing unit tests in Next.js. Let’s take a look at an example:
jsx // MyComponent.test.js import { render, screen } from '@testing-library/react'; import MyComponent from '../MyComponent'; describe('MyComponent', () => { it('renders correctly', () => { render(<MyComponent />); expect(screen.getByText('Hello, World!')).toBeInTheDocument(); }); });
In this example, we render MyComponent using the render function from @testing-library/react and assert that the “Hello, World!” text is present in the rendered output.
4. Testing Next.js API Routes
Next.js API routes allow you to create serverless API endpoints within your Next.js application. These API routes can be tested using Jest’s built-in testing capabilities. Here’s an example of testing a Next.js API route:
javascript // api.test.js import { createMocks } from 'node-mocks-http'; import handler from '../pages/api/hello'; describe('/api/hello', () => { it('returns a JSON response with the correct message', async () => { const { req, res } = createMocks({ method: 'GET', }); await handler(req, res); expect(res._getStatusCode()).toBe(200); expect(JSON.parse(res._getData())).toEqual({ message: 'Hello, API!' }); }); });
In this example, we create mock request and response objects using node-mocks-http and test the API handler’s behavior.
5. Integration Testing with Next.js
Integration testing ensures that different parts of an application work together correctly. Next.js applications often involve interactions between components, API routes, and external services. Tools like Jest and Testing Library are well-suited for integration testing Next.js applications. Here’s an example:
jsx // integration.test.js import { render, screen, waitFor } from '@testing-library/react'; import userEvent from '@testing-library/user-event'; import App from '../pages/index'; describe('Integration Test', () => { it('should render a greeting when the button is clicked', async () => { render(<App />); userEvent.click(screen.getByRole('button', { name: 'Show Greeting' })); await waitFor(() => expect(screen.getByText('Hello, World!')).toBeInTheDocument() ); }); });
In this example, we render the App component, simulate a button click using @testing-library/user-event, and assert that the “Hello, World!” greeting is displayed after the button click.
6. Snapshot Testing in Next.js
Snapshot testing is a useful technique to capture the rendered output of a component and compare it against a previously saved snapshot. This ensures that the component’s UI doesn’t unintentionally change over time. Jest provides built-in snapshot testing support for Next.js. Here’s an example:
jsx // MyComponent.test.js import { render } from '@testing-library/react'; import MyComponent from '../MyComponent'; describe('MyComponent', () => { it('renders correctly', () => { const { container } = render(<MyComponent />); expect(container.firstChild).toMatchSnapshot(); }); });
In this example, we render MyComponent and assert that the rendered output matches the previously saved snapshot.
7. End-to-End Testing Next.js Applications
End-to-end (E2E) testing involves testing the complete flow of an application from start to finish. Tools like Cypress and Playwright can be used for E2E testing in Next.js. These tools allow you to simulate user interactions and assert the application’s behavior in a real browser environment. Here’s an example using Cypress:
javascript // myComponent.spec.js describe('MyComponent', () => { it('renders correctly', () => { cy.visit('/'); cy.findByText('Hello, World!').should('exist'); }); });
In this example, we use Cypress to visit the application’s root URL and assert that the “Hello, World!” text exists.
8. Code Coverage in Next.js
Code coverage measures how much of your application’s code is being tested. Jest provides built-in code coverage support, allowing you to analyze the effectiveness of your tests. To generate a code coverage report for your Next.js application, run the following command:
css $ jest --coverage
This command will produce a detailed report indicating which parts of your code are covered by tests and which are not.
9. Continuous Integration and Deployment (CI/CD) for Next.js Testing
Integrating testing into your CI/CD pipeline ensures that your Next.js application is thoroughly tested before deployment. Popular CI/CD platforms like GitHub Actions, GitLab CI/CD, and CircleCI support Next.js testing. By automating your testing process, you can catch issues early and deliver high-quality applications consistently.
Conclusion
Testing Next.js applications is crucial for ensuring their quality and reliability. By adopting effective testing strategies and utilizing essential tools like Jest, Testing Library, Cypress, and others, you can confidently build robust applications that meet user expectations. Incorporate testing into your development workflow from the early stages to save time and effort in the long run. Happy testing!
In conclusion, testing Next.js applications plays a vital role in delivering high-quality software. With the strategies and tools discussed in this blog post, you’re equipped to enhance your Next.js testing practices and build reliable applications. Stay diligent in your testing efforts, continuously improve your test suite, and enjoy the benefits of a well-tested Next.js application.
Table of Contents
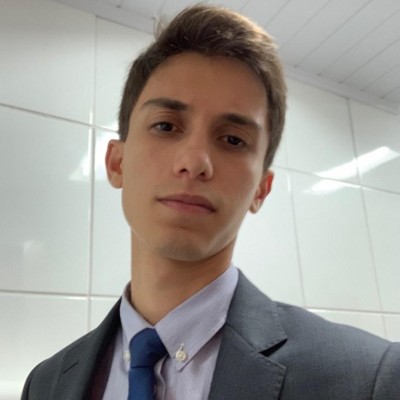
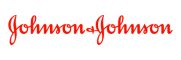