Implementing User Authentication in NEXT.js: A Step-by-Step Guide
Authentication is an essential part of most modern web applications. This process helps maintain the security of user data by ensuring only authorized individuals have access to certain information. With its complexity, many businesses opt to hire Next.js developers for efficient and secure implementation. In this blog post, we will explore how to build an authentication system using NEXT.js, a popular framework based on React.js that enables server-side rendering and generates static websites.
NEXT.js has gained traction in the developer community for its performance, scalability, and ease of use. However, managing user authentication can still be a challenge, which is another reason why hiring Next.js developers can be beneficial. Our goal in this article is to make this task easier for you by providing concrete examples, whether you choose to implement it yourself or hire Next.js developers to do it for you.
Getting Started with NEXT.js
Before we delve into authentication, let’s briefly touch upon how to get started with a NEXT.js project. First, make sure you have Node.js and npm installed on your computer. Then, you can create a new NEXT.js application by running the following command in your terminal:
```bash npx create-next-app@latest ```
This will generate a new NEXT.js application in your current directory.
Understanding Authentication
Authentication is the process of verifying a user’s identity, an integral task often managed by skilled professionals such as Next.js developers. This process typically involves the user providing some credentials, like a username and password, and checking these credentials against a database. If the credentials match, the user is granted access.
Authentication can be implemented in many ways, but one common approach especially when you hire Next.js developers, is the use of JWT (JSON Web Tokens). JWTs allow you to store user data in a secure, encoded token that can be sent between the client and the server. This token can be stored in the user’s browser and sent with each request to ensure the user is authenticated, a strategy often used by experienced Next.js developers.
Building an Authentication System in NEXT.js
Now that we’ve laid the groundwork, let’s dive into how we can build an authentication system using NEXT.js.
1. Setting up a User Model
Firstly, we need a User model to store our user data. We will use MongoDB for this purpose, but you can choose any database that suits your needs.
Install Mongoose, a MongoDB object modeling tool, by running `npm install mongoose` in your terminal.
Here’s an example of a basic User schema:
```javascript const mongoose = require('mongoose'); const UserSchema = new mongoose.Schema({ username: { type: String, required: true, unique: true, }, password: { type: String, required: true, }, }); module.exports = mongoose.models.User || mongoose.model('User', UserSchema); ```
In this model, we have a username that must be unique, and a password.
2. Creating Routes
NEXT.js uses a file-based routing system. It automatically routes files in the `pages` folder, so you don’t need to create a separate router file. To create a route, you simply create a file in the `pages` folder.
For authentication, we’ll need two routes: a signup route and a login route.
Signup Route
In your `pages` folder, create a file named `signup.js`. This file will handle the signup requests. We need to collect user credentials and store them in our database. Here’s an example:
```javascript import { useRouter } from 'next/router'; import User from '../models/User'; export default function Signup() { const router = useRouter(); const handleSubmit = async event => { event.preventDefault(); const { username, password } = event.target; // store user in database const user = new User({ username: username.value, password: password.value }); await user.save(); // redirect to home page router.push('/'); }; return ( <form onSubmit={handleSubmit}> <input name="username" type="text" placeholder="Username" required /> <input name="password" type="password" placeholder="Password" required /> <button type="submit">Sign up</button> </form> ); } ```
Login Route
Similarly, create a `login.js` file in your `pages` folder. This file will handle the login requests. We need to collect user credentials and compare them with our database. If the credentials match, the user will be authenticated.
```javascript import { useRouter } from 'next/router'; import jwt from 'jsonwebtoken'; import User from '../models/User'; export default function Login() { const router = useRouter(); const handleSubmit = async event => { event.preventDefault(); const { username, password } = event.target; // check if user exists const user = await User.findOne({ username: username.value, password: password.value }); if (!user) { return alert('Invalid credentials'); } // create jwt const token = jwt.sign({ id: user._id }, 'mySecretKey'); // store token in cookie document.cookie = `token=${token}; path=/`; // redirect to home page router.push('/'); }; return ( <form onSubmit={handleSubmit}> <input name="username" type="text" placeholder="Username" required /> <input name="password" type="type" placeholder="Password" required /> <button type="submit">Login</button> </form> ); } ```
Please note that in a real-world application, you should hash the user’s password before storing it and compare hashed values during the login process. Libraries like `bcrypt` can help with this.
3. Authenticated Routes
Now, let’s create a route that should only be accessible to authenticated users. In the `pages` folder, create a file called `secret.js`. We can use the `getServerSideProps` function provided by NEXT.js to check if the user is authenticated before rendering the page.
```javascript import jwt from 'jsonwebtoken'; export default function Secret({ user }) { return <h1>Hello, {user.username}!</h1>; } export async function getServerSideProps(context) { // get the user token from the cookies const token = context.req.cookies.token; if (!token) { return { props: {} }; } // verify user token const { id } = jwt.verify(token, 'mySecretKey'); const user = await User.findById(id); if (!user) { return { props: {} }; } // send user data to the client return { props: { user } }; } ```
If the user is not authenticated, they will not see the personalized greeting message.
Conclusion
Building an authentication system in NEXT.js can seem daunting at first, but with a step-by-step approach, it becomes manageable. This may be why many choose to hire Next.js developers to streamline the process. Remember that this simple example doesn’t cover every aspect of a robust authentication system, such as hashing passwords, handling tokens securely, and error management. However, it provides a solid foundation for Next.js developers to expand upon.
By understanding these basic principles, you, or the Next.js developers you hire, can create more complex systems that suit your application’s unique needs, always keeping security as a priority.
Table of Contents
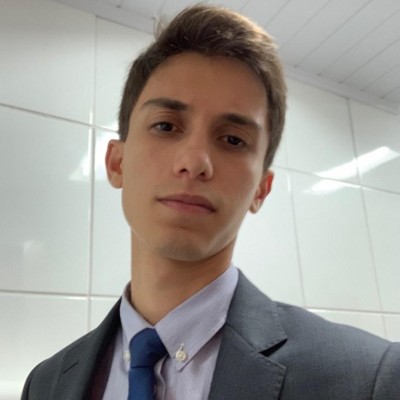
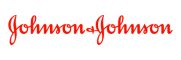