Node.js Uncovered: A Comprehensive Beginner’s Guide to Server-Side JavaScript Development
Welcome to this beginner’s guide on Node.js! In the realm of web development, there is an array of technologies to choose from. One that stands out particularly for server-side programming is Node.js, a technology that many companies hire Node.js developers for due to its efficiency and versatility. As a beginner, you may wonder: what is Node.js and how can I use it? Let’s embark on an enlightening journey to demystify this powerful tool and help you get started on your Node.js journey!
What is Node.js?
Node.js is an open-source runtime environment that uses JavaScript to build server-side and networking applications. It uses the Chrome V8 JavaScript engine, and it’s built on an event-driven, non-blocking I/O model. This makes it lightweight, efficient, and excellent for data-intensive real-time applications. Its effectiveness has led many companies to hire Node.js developers to leverage this technology.
Here’s a fun fact: Node.js was created by Ryan Dahl in 2009 to rectify the problem of the concurrent request handling that he perceived in popular web servers of the time. Now, Node.js is used by millions of developers and big-name companies like Netflix, LinkedIn, and Uber, all of which hire Node.js developers to harness the power of this runtime environment.
Why Use Node.js?
Node.js is known for its speed and efficiency, making it a perfect choice for applications that handle many simultaneous client connections with low latency.
Here are some advantages of using Node.js:
– Single-threaded, Non-blocking Model: Node.js operates on a single thread, using non-blocking I/O calls, allowing it to support thousands of concurrent connections without incurring the cost of thread context switching. This makes Node.js incredibly efficient and lightweight.
– JavaScript Everywhere: Since Node.js uses JavaScript, you can use the same language for both the client and server sides. This makes it easier to share code between the frontend and backend and promotes greater coherence in web development.
– Rich Ecosystem: Node.js has an extensive ecosystem of libraries and frameworks available through the Node Package Manager (NPM), making it easy to extend the functionality of your applications.
Getting Started with Node.js
To start, you’ll need to install Node.js on your machine. Head to the official Node.js website (https://nodejs.org) and download the LTS (Long Term Support) version. This will also install NPM (Node Package Manager), a useful tool for managing Node.js packages.
To check if Node.js is installed correctly, open your terminal or command line and type:
```bash node -v npm -v ```
These commands will return the installed versions of Node.js and NPM, respectively.
Your First Node.js Application
Let’s create a simple “Hello, World!” application. Create a new file named `app.js` and add the following code:
```javascript console.log('Hello, World!'); ```
To run this Node.js application, type the following command in your terminal:
```bash node app.js ```
If everything is correctly set up, “Hello, World!” will be displayed in your console. Congratulations, you just ran your first Node.js application!
Building a Simple HTTP Server
Node.js excels at building networking applications like web servers. Let’s create a basic HTTP server that responds with a “Hello, World!” message.
Create a new file named `server.js` and add the following code:
```javascript const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, World!\n'); }); const port = 3000; server.listen(port, () => { console.log(`Server running at http://localhost:${port}/`); }); ``` To run this server, type the following command in your terminal: ```bash node server.js ```
If everything works correctly, you should see the message “Server running at http://localhost:3000/” in your console. Open this URL in your web browser. You should see “Hello, World!” displayed in the browser.
Using NPM and External Modules
As mentioned earlier, Node.js has a vast ecosystem of external libraries available through NPM. Let’s use an external library, `axios`, to make HTTP requests.
First, we need to initialize our application with NPM. In your terminal, type:
```bash npm init -y ```
This command creates a `package.json` file in your application directory, which will keep track of your application’s dependencies.
To install `axios`, type:
```bash npm install axios ```
Now, let’s create a simple application that fetches data from an external API. Create a new file named `api.js` and add the following code:
```javascript const axios = require('axios'); axios.get('https://api.github.com/users/github') .then(response => { console.log(response.data); }) .catch(error => { console.error(error); }); ```
To run this application, type the following command in your terminal:
```bash node api.js ```
This application will output data about the ‘github’ user from the GitHub API to your console.
Conclusion
That’s it for our beginner’s guide to Node.js. We’ve explored what Node.js is, why it’s beneficial, and how to start creating applications with it. If your project needs advanced features, you might want to consider hiring Node.js developers. Remember, Node.js is a tool, and like any tool, its effectiveness depends on how it’s used. As you continue your Node.js journey, strive to understand its strengths and weaknesses, and use it to its fullest potential. Good luck!
Table of Contents
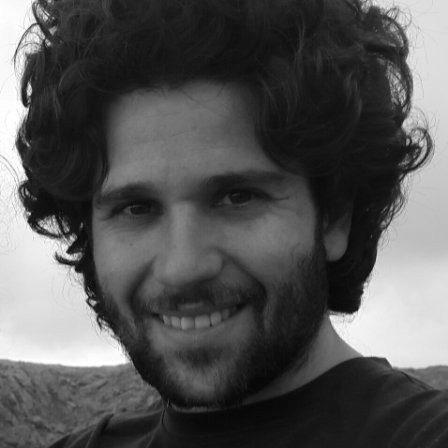
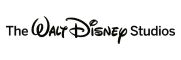