Building RESTful Web Services with Node.js and Hapi.js
Are you ready to dive into the world of building robust and scalable RESTful web services using Node.js and Hapi.js? In this guide, we’ll explore the ins and outs of RESTful architecture, learn how to implement it using Node.js and Hapi.js, and provide you with real-world examples to solidify your understanding.
Understanding RESTful Architecture
Representational State Transfer (REST) is an architectural style for designing networked applications. It emphasizes a stateless client-server model where web services are treated as resources and can be identified by their unique URIs. RESTful services use standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on these resources, making them highly scalable and easy to integrate with other systems.
Getting Started with Node.js and Hapi.js
Node.js is a powerful runtime environment for building server-side applications in JavaScript. Hapi.js, on the other hand, is a rich framework for building web applications and services. Together, they provide a solid foundation for creating RESTful APIs with ease.
To begin, make sure you have Node.js installed on your machine. You can download it from here.
Next, let’s create a new Node.js project and install Hapi.js:
mkdir my-rest-api cd my-rest-api npm init -y npm install @hapi/hapi
Now that we have our project set up, let’s dive into building our RESTful web service.
Building a RESTful API with Hapi.js
First, let’s create a basic Hapi.js server:
const Hapi = require('@hapi/hapi'); const init = async () => { const server = Hapi.server({ port: 3000, host: 'localhost' }); server.route({ method: 'GET', path: '/', handler: (request, h) => { return 'Hello World!'; } }); await server.start(); console.log('Server running on %s', server.info.uri); }; init();
Save this code in a file named server.js and run it using node server.js. You should see “Server running on http://localhost:3000” printed to the console.
Now, let’s add some routes to create a basic RESTful API:
server.route({ method: 'GET', path: '/api/users', handler: (request, h) => { // Retrieve and return a list of users } }); server.route({ method: 'GET', path: '/api/users/{id}', handler: (request, h) => { // Retrieve and return a specific user } }); server.route({ method: 'POST', path: '/api/users', handler: (request, h) => { // Create a new user } }); server.route({ method: 'PUT', path: '/api/users/{id}', handler: (request, h) => { // Update an existing user } }); server.route({ method: 'DELETE', path: '/api/users/{id}', handler: (request, h) => { // Delete a user } });
These routes represent typical CRUD operations (Create, Read, Update, Delete) for managing users.
Real-World Examples
To better understand how to build RESTful web services with Node.js and Hapi.js, let’s explore some real-world examples:
- Todo List Application: Build a simple todo list application with CRUD operations for managing tasks.
- TodoMVC – TodoMVC provides a collection of examples for building todo list applications using different JavaScript frameworks.
- Blog API: Create an API for managing blog posts, comments, and users.
- JSONPlaceholder – JSONPlaceholder is a free online REST API that you can use for testing and prototyping.
- E-commerce Backend: Develop a backend system for an e-commerce website with product listings, shopping carts, and orders.
- Stripe API – Stripe provides powerful APIs for processing payments and managing e-commerce transactions.
Conclusion
Building RESTful web services with Node.js and Hapi.js opens up a world of possibilities for creating scalable and maintainable applications. By following the principles of RESTful architecture and leveraging the capabilities of Node.js and Hapi.js, you can build robust APIs that power your applications with ease.
Now that you have the knowledge and tools at your disposal, it’s time to start building your own RESTful web services and take your projects to the next level!
Happy coding!
External Resources:
- Node.js Official Website
- Hapi.js Documentation
- RESTful API Design: Best Practices
Table of Contents
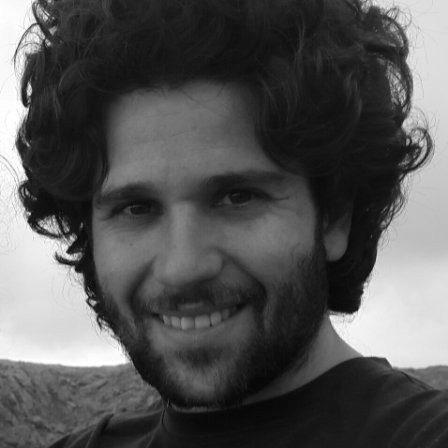
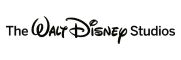