Node.js Q & A
How do you handle caching in Node.js?
Caching in Node.js involves storing frequently accessed data in memory or a persistent storage mechanism to improve performance and reduce response times. Caching can be implemented at various levels within a Node.js application, including application-level caching, database query caching, and HTTP response caching.
Here are some common techniques for handling caching in Node.js:
- Application-Level Caching: Node.js applications can cache data in memory using objects, arrays, or caching libraries like memory-cache, node-cache, or lru-cache. This allows you to store frequently accessed data, such as computed results, database query results, or API responses, in memory for fast access and retrieval.
const cache = require('memory-cache'); // Cache data cache.put('key', 'value', 10000); // Cache expires after 10 seconds // Retrieve cached data const cachedValue = cache.get('key');
- Database Query Caching: When working with databases, you can implement query caching to store the results of frequently executed database queries. This reduces the load on the database server and improves response times for subsequent identical queries.
const { promisify } = require('util'); const redis = require('redis'); const client = redis.createClient(); // Promisify Redis commands const getAsync = promisify(client.get).bind(client); const setAsync = promisify(client.set).bind(client); // Example of caching database query results using Redis async function getCachedData(key) { const cachedData = await getAsync(key); if (cachedData) { console.log('Data retrieved from cache'); return JSON.parse(cachedData); } else { console.log('Fetching data from database'); const data = await fetchDataFromDatabase(); await setAsync(key, JSON.stringify(data), 'EX', 60); // Cache expires after 60 seconds return data; } }
- HTTP Response Caching: In web applications, you can cache HTTP responses to improve performance and reduce server load. This involves setting appropriate cache-control headers in HTTP responses to instruct clients (browsers) and intermediary caches (e.g., CDN) to cache the responses for a specified period.
// Example of setting cache-control headers in Express.js app.get('/data', (req, res) => { res.setHeader('Cache-Control', 'public, max-age=3600'); // Cache response for 1 hour // Send response });
By implementing caching techniques in your Node.js application, you can optimize performance, reduce resource consumption, and enhance scalability, leading to improved user experiences and cost-effectiveness.
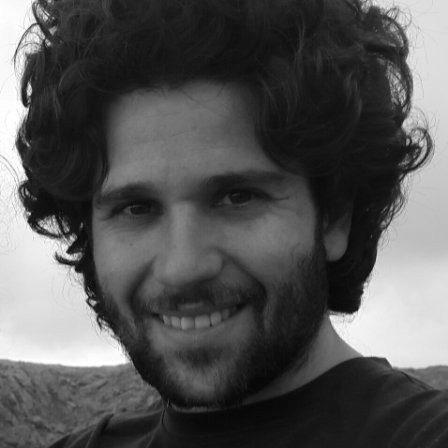
Previously at
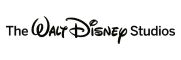
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.