What is callback hell in Node.js?
Callback hell, also known as the Pyramid of Doom, is a common problem in Node.js caused by excessive nesting of asynchronous callback functions. In complex asynchronous codebases, callback functions can become deeply nested, leading to code that is difficult to read, understand, and maintain.
Here’s an example of callback hell in Node.js:
javascript Copy code asyncFunction1((err, result1) => { if (err) { console.error('Error:', err); return; } asyncFunction2(result1, (err, result2) => { if (err) { console.error('Error:', err); return; } asyncFunction3(result2, (err, result3) => { if (err) { console.error('Error:', err); return; } // More nested callback functions... }); }); });
As you can see, as more asynchronous operations are nested within each other, the code becomes increasingly difficult to read and maintain. This can lead to issues such as callback hell, where it becomes challenging to reason about the flow of control and handle errors effectively.
To mitigate callback hell, Node.js developers have adopted various techniques such as modularization, using Promises, and adopting async/await syntax. These approaches help flatten the callback pyramid and make asynchronous code more readable and manageable.
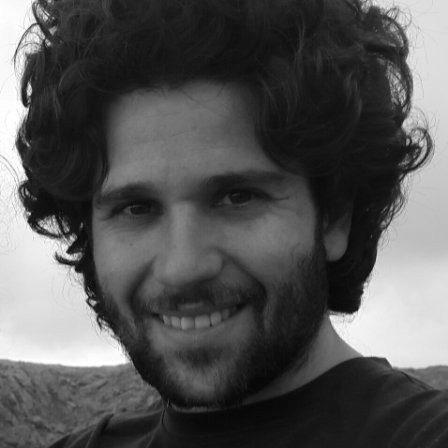
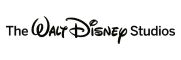