What is a child process in Node.js?
In Node.js, a child process is a separate instance of the Node.js runtime that can be spawned from the main or parent process. Child processes allow Node.js applications to execute external commands, run shell scripts, perform CPU-intensive or long-running tasks asynchronously, and utilize multiple CPU cores efficiently.
Node.js provides the child_process module to facilitate communication with child processes and manage their lifecycle. The child_process module offers several functions for creating and interacting with child processes, including spawn(), exec(), execFile(), and fork().
- spawn(): The spawn() function spawns a new process and returns a ChildProcess object that represents the spawned process. It is suitable for executing long-running commands or scripts with large amounts of data.
- exec(): The exec() function is similar to spawn() but provides a higher-level interface for executing shell commands. It buffers the output of the command and passes it to a callback function when the command completes.
- execFile(): The execFile() function is similar to exec() but directly executes a file instead of a shell command. It’s suitable for executing executable files, shell scripts, or other binaries.
- fork(): The fork() function is a specialized form of spawn() specifically designed for creating Node.js child processes. It allows communication between the parent and child processes using inter-process communication (IPC) and event listeners.
Here’s a basic example of using spawn() to execute a shell command in a child process:
javascript
const { spawn } = require('child_process'); const ls = spawn('ls', ['-lh', '/usr']); ls.stdout.on('data', (data) => { console.log(`stdout: ${data}`); }); ls.stderr.on('data', (data) => { console.error(`stderr: ${data}`); }); ls.on('close', (code) => { console.log(`child process exited with code ${code}`); });
Copy code
By leveraging child processes in Node.js, developers can improve application performance, scalability, and reliability by offloading CPU-intensive tasks and executing concurrent operations in parallel.
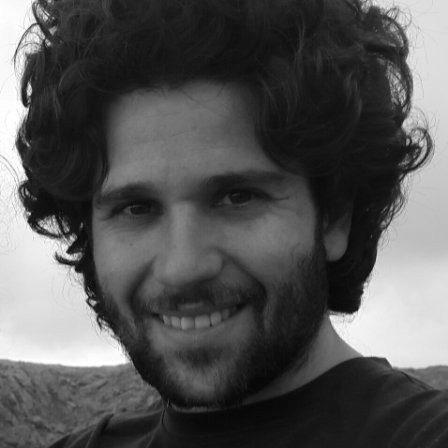
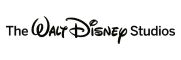