What is the purpose of the cluster module in Node.js?
The cluster module in Node.js allows developers to create child processes (workers) that share the same server port, enabling efficient utilization of multi-core CPUs and improving application scalability and performance. The primary purpose of the cluster module is to facilitate process forking and load balancing in Node.js applications.
Key functionalities of the cluster module include:
- Forking Child Processes:
-
-
- The cluster.fork() method forks a new child process from the master process, allowing multiple instances of the application to run concurrently and handle incoming requests simultaneously.
- Example:
- javascript
-
- Copy code
const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { // Fork workers based on the number of CPU cores for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { // Worker process logic http.createServer((req, res) => { res.writeHead(200); res.end('Hello, World!'); }).listen(3000); }
- Load Balancing:
-
-
- The cluster module performs automatic round-robin load balancing by distributing incoming connections across worker processes. It helps distribute workload evenly across CPU cores and prevents any single worker from becoming a bottleneck.
-
- Inter-Process Communication (IPC):
-
-
- Worker processes can communicate with the master process using IPC (Inter-Process Communication) channels provided by the cluster module. They can exchange messages, share data, and coordinate tasks efficiently.
-
- Fault Tolerance and Resilience:
-
-
- The cluster module enhances application fault tolerance and resilience by isolating worker processes. If a worker process crashes or becomes unresponsive, the master process can restart it automatically without affecting other workers.
-
- Graceful Shutdown:
-
-
- The cluster module supports graceful shutdown of worker processes and the master process. When the master process receives termination signals (e.g., SIGINT, SIGTERM), it gracefully shuts down worker processes and exits cleanly.
-
- Monitoring and Management:
-
- Developers can monitor and manage worker processes using built-in monitoring tools like cluster.schedulingPolicy, cluster.workers, and cluster.on(‘exit’) event listeners. They can collect performance metrics, handle worker failures, and scale the application dynamically based on workload.
The cluster module is particularly useful for building scalable and high-performance Node.js applications that can leverage multi-core CPUs effectively. By utilizing the cluster module, developers can achieve better concurrency, improved throughput, and enhanced fault tolerance, making their applications more robust and efficient.
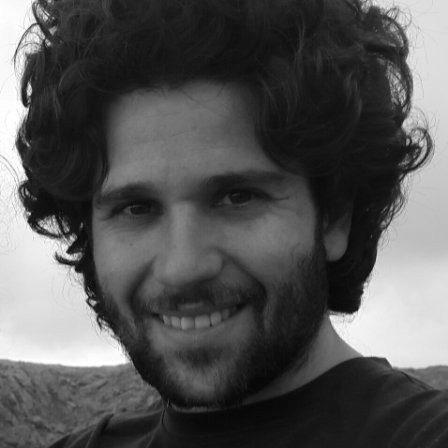
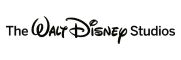